How to Set Columns as Index in Pandas Dataframe
- Method 1: Using set_index() Function
- Method 2: Using index_col Parameter When Reading a File
- Conclusion
- FAQ
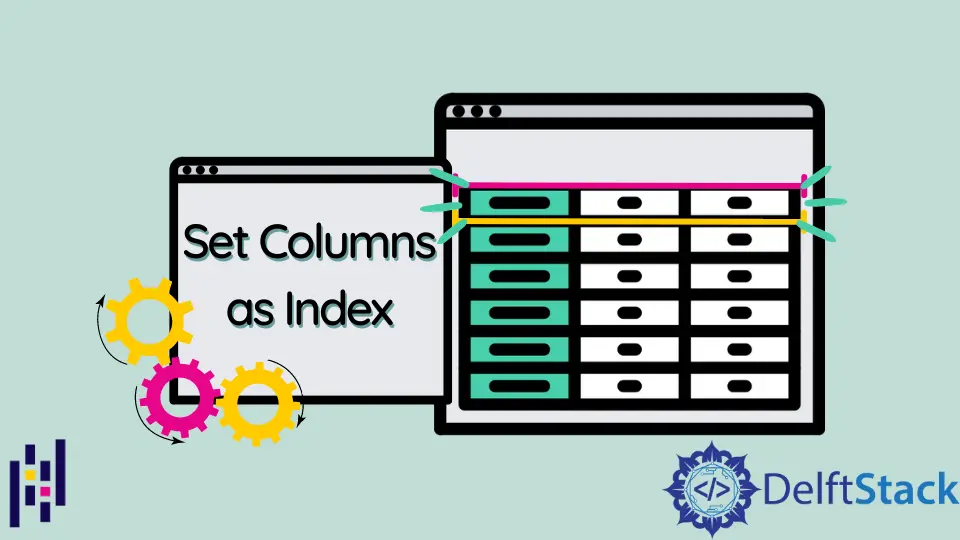
Setting columns as an index in a Pandas DataFrame is a fundamental operation that can streamline your data analysis process. Whether you’re preparing data for machine learning, performing data manipulation, or simply organizing your DataFrame, understanding how to set an index is crucial.
In this tutorial, we will explore two primary methods to achieve this: using the set_index()
function and the index_col
parameter while reading a file. By the end of this article, you will have a solid grasp of how to manipulate DataFrames efficiently, enhancing your data handling skills in Python.
Method 1: Using set_index() Function
The set_index()
function in Pandas allows you to specify one or more columns as the index of your DataFrame. This method is particularly useful when you already have a DataFrame and want to change its index.
Here’s how you can use the set_index()
function:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
df.set_index('Name', inplace=True)
print(df)
Output:
Age City
Name
Alice 25 New York
Bob 30 Los Angeles
Charlie 35 Chicago
In this example, we first create a DataFrame from a dictionary containing names, ages, and cities. We then use the set_index()
function to set the ‘Name’ column as the index of the DataFrame. The inplace=True
argument modifies the original DataFrame directly, so the changes are saved without needing to assign the output to a new variable. After executing this code, you’ll notice that the index is now based on the names, which can make data look neater and facilitate easier access to rows based on names.
Method 2: Using index_col Parameter When Reading a File
Another efficient way to set an index in a DataFrame is by using the index_col
parameter when reading a file, such as a CSV. This method is particularly handy when you’re importing data directly from external sources.
Here’s how to do it:
import pandas as pd
df = pd.read_csv('data.csv', index_col='Name')
print(df)
Output:
Age City
Name
Alice 25 New York
Bob 30 Los Angeles
Charlie 35 Chicago
In this example, we use the pd.read_csv()
function to read a CSV file named ‘data.csv’. By specifying index_col='Name'
, we instruct Pandas to use the ‘Name’ column as the index right from the moment the data is loaded. This approach is efficient because it avoids the need for a separate step to set the index afterward. The result is a DataFrame where the ‘Name’ column serves as the index, allowing for quick lookups and better organization of the data.
Conclusion
Setting columns as an index in a Pandas DataFrame is a powerful technique that can enhance your data analysis capabilities. Whether you choose to use the set_index()
function or the index_col
parameter when reading a file, both methods provide flexibility and efficiency. Mastering these techniques will not only make your data manipulation tasks easier but also improve the readability of your DataFrames. With practice, you’ll find these methods become second nature, allowing you to focus more on analyzing your data rather than dealing with its structure.
FAQ
-
What is the purpose of setting an index in a DataFrame?
Setting an index helps organize your data, making it easier to access specific rows and perform operations like filtering and grouping. -
Can I set multiple columns as an index in Pandas?
Yes, you can set multiple columns as an index by passing a list of column names to theset_index()
function. -
What happens to the original column when I set it as an index?
The original column becomes the index and is removed from the DataFrame’s columns, but you can keep it by settingdrop=False
. -
Is it possible to reset the index back to the default?
Yes, you can reset the index using thereset_index()
function, which will revert the index back to the default integer index. -
Can I set an index when reading different file formats?
Yes, similar to CSV files, you can set an index when reading other file formats like Excel or JSON using the appropriate Pandas functions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn