在 Pandas Dataframe 中设置列作为索引
Manav Narula
2023年1月30日
-
使用
set_index()
在 Pandas DataFrame 中指定列作为索引 -
使用
read_excel
或read_csv
中的index_col
参数在 Pandas DataFrame 中将列作为索引
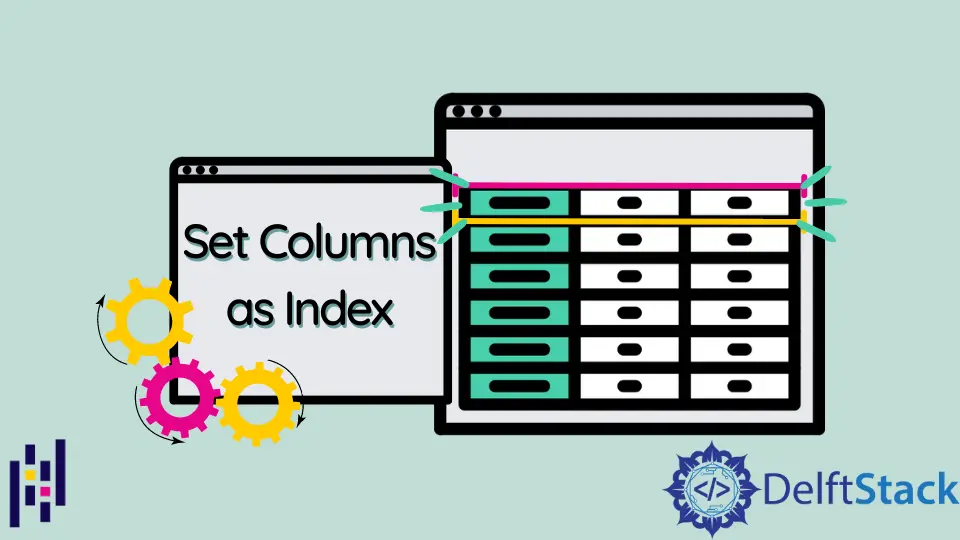
通常,在 Pandas Dataframe 中,我们默认以 0 到对象长度的序列号作为索引。我们也可以将 DataFrame 中的某一列作为其索引。为此,我们可以使用 pandas 中提供的 set_index()
,也可以在从 excel 或 CSV 文件中导入 DataFrame 时指定列作为索引。
使用 set_index()
在 Pandas DataFrame 中指定列作为索引
set_index()
可以应用于列表、序列或 DataFrame 来改变它们的索引。对于 DataFrame,set_index()
也可以将多个列作为它们的索引。
例:
import pandas as pd
import numpy as np
colnames = ["Name", "Time", "Course"]
df = pd.DataFrame(
[["Jay", 10, "B.Tech"], ["Raj", 12, "BBA"], ["Jack", 11, "B.Sc"]], columns=colnames
)
print(df)
输出:
Name Time Course
0 Jay 10 B.Tech
1 Raj 12 BBA
2 Jack 11 B.Sc
将列作为索引的语法:
dataframe.set_index(Column_name, inplace=True)
使用 set_index()
将一列作为索引。
import pandas as pd
import numpy as np
colnames = ["Name", "Time", "Course"]
df = pd.DataFrame(
[["Jay", 10, "B.Tech"], ["Raj", 12, "BBA"], ["Jack", 11, "B.Sc"]], columns=colnames
)
df.set_index("Name", inplace=True)
print(df)
输出:
Time Course
Name
Jay 10 B.Tech
Raj 12 BBA
Jack 11 B.Sc
使多列作为索引:
import pandas as pd
import numpy as np
colnames = ["Name", "Time", "Course"]
df = pd.DataFrame(
[["Jay", 10, "B.Tech"], ["Raj", 12, "BBA"], ["Jack", 11, "B.Sc"]], columns=colnames
)
df.set_index(["Name", "Course"], inplace=True)
print(df)
输出:
Time
Name Course
Jay B.Tech 10
Raj BBA 12
Jack B.Sc 11
使用 read_excel
或 read_csv
中的 index_col
参数在 Pandas DataFrame 中将列作为索引
当从 excel 或 CSV 文件中读取 DataFrame 时,我们可以指定我们想要的列作为 DataFrame 的索引。
例:
import pandas as pd
import numpy as np
df = pd.read_excel("data.xlsx", index_col=2)
print(df)
输出:
Name Time
Course
B.Tech Mark 12
BBA Jack 10
B.Sc Jay 11
作者: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn