How to Save Pandas DataFrame as HTML Using Python
-
Use the
to_html()
Method to Save a Pandas DataFrame as HTML File -
Convert Pandas DataFrame to
HTML
Table Manually
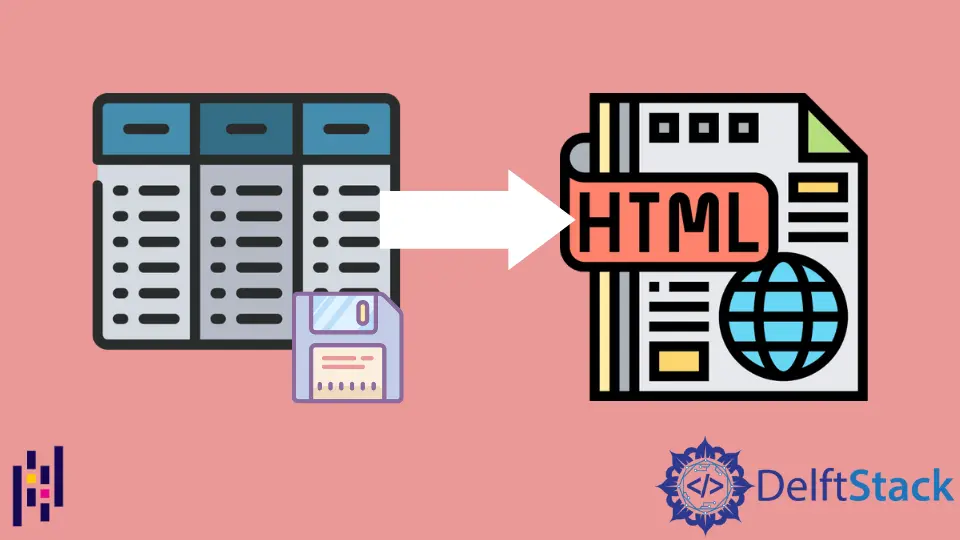
Sometimes we render the dataframe to an HTML table to represent it in web pages. If we want to display the same table in HTML, we don’t need to write its code in HTML to make that table again.
We can use a built-in method or write code manually in python to convert a Pandas dataframe to an HTML table, which will be discussed in this article.
Use the to_html()
Method to Save a Pandas DataFrame as HTML File
In the following code, we have the students’ data. We converted the Pandas dataframe to HTML using the method to_html()
available in the pandas library.
We’ll call this method with our dataframe object and pass the name for the new HTML file representing the table. If we only pass the name of the HTML file, it will be created in the current directory.
We can also give a path along with the name of the HTML file to save it somewhere else. This method will convert the whole dataframe into a <table>
element, and the columns will be wrapped inside the <thead>
element.
Each row of the dataframe will be wrapped inside the <tr>
element of HTML. This method will return the HTML format of the dataframe.
Example Code:
# Python 3.x
import pandas as pd
df = pd.read_csv("Student.csv")
display(df)
df.to_html("Student.html")
Output:
The output will be inside the Student.html
file.
HTML - Code:
<table border="1" class="dataframe">
<thead>
<tr style="text-align: right;">
<th></th>
<th>ST_Name</th>
<th>Department</th>
<th>Marks</th>
</tr>
</thead>
<tbody>
<tr>
<th>0</th>
<td>Jhon</td>
<td>CS</td>
<td>60</td>
</tr>
<tr>
<th>1</th>
<td>Alia</td>
<td>EE</td>
<td>80</td>
</tr>
<tr>
<th>2</th>
<td>Sam</td>
<td>EE</td>
<td>90</td>
</tr>
<tr>
<th>3</th>
<td>Smith</td>
<td>CS</td>
<td>88</td>
</tr>
</tbody>
</table>
Convert Pandas DataFrame to HTML
Table Manually
Another way to save Pandas dataframe as HTML is to write the code from scratch for conversion manually.
First, we have opened a file student.html
with w+
mode in the following code. This mode will create a file if it doesn’t exist already.
In this file, our HTML code will be saved. Here, we have wrapped the whole table inside <table>
, and then we have iterated over the columns of the dataframe and wrapped them inside <th>
.
Finally, we have iterated upon the rows and wrapped them in <tr>
.
Example Code:
# Python 3.x
import pandas as pd
df = pd.read_csv("Student.csv")
display(df)
with open(r"student.html", "w+") as f:
f.write("<table>")
for header in df.columns.values:
f.write("<th>" + str(header) + "</th>")
for i in range(len(df)):
f.write("<tr>")
for col in df.columns:
value = df.iloc[i][col]
f.write("<td>" + str(value) + "</td>")
f.write("</tr>")
f.write("</table>")
Output:
HTML - Code:
<table><th>ST_Name</th><th>Department</th><th>Marks</th><tr><td>Jhon</td><td>CS</td><td>60</td></tr><tr><td>Alia</td><td>EE</td><td>80</td></tr><tr><td>Sam</td><td>EE</td><td>90</td></tr><tr><td>Smith</td><td>CS</td><td>88</td></tr></table>
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn