Python Contextlib
-
Use the
with
Statement as a Context Manager in Python -
Use Function Decorator
@contextmanager
in Python
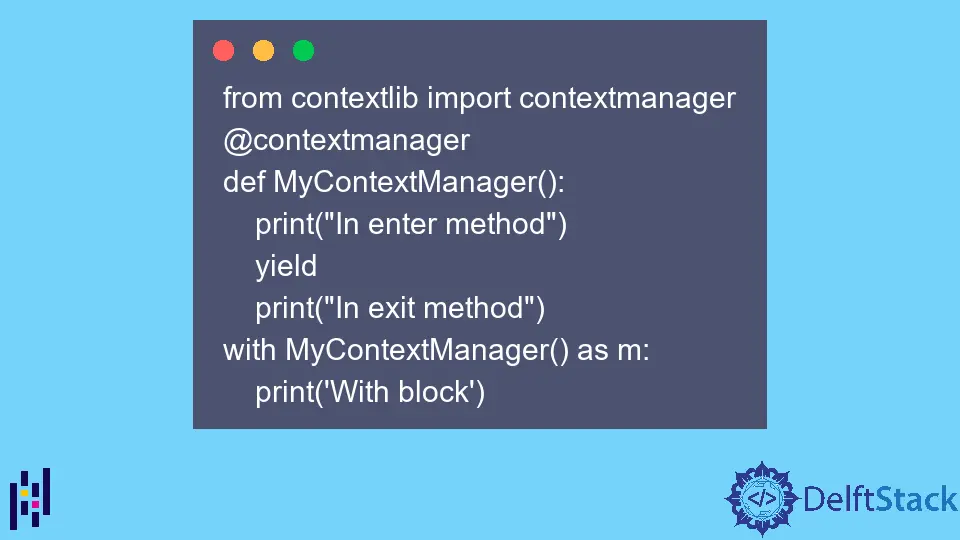
Sometimes, we use some resources such as a file. We open it and forget to close it when we finish working with it.
It is a bad coding practice and creates problems when too many files are open. Even if we no longer require those resources, the program may acquire them indefinitely.
This situation causes memory leaks. To handle these things automatically, Python has context managers.
They take care of managing the resources. The contextlib
is a Python library that provides utilities for resource management using the with
keyword.
Use the with
Statement as a Context Manager in Python
In Python, the with
statement manages resources and handles exceptions. So it works as a context manager.
When the code reaches a with
statement, it temporarily allocates resources. The previously acquired resources are released when the with
statement block finishes its execution.
In the following code, we have created and opened a file. The resources acquired in the with
block will release when the control is out of the with
block.
Example Code:
with open("file.txt", "w+") as f:
print("File Opened")
Output:
File Opened
We can include context manager functionality in our programs as well. For this, our class must have the methods __enter__()
and __exit__()
.
To understand the flow of the context manager, we have executed the following code with the print
statement that runs when the control transfers to the respective block.
Example Code:
class MyContextManager:
def __init__(self):
print("In init method")
def __enter__(self):
print("In enter method")
def __exit__(self, exc_type, exc_value, exc_traceback):
print("In exit method")
with MyContextManager() as m:
print("With block")
Output:
In init method
In enter method
With block
In exit method
Use Function Decorator @contextmanager
in Python
Another method to make a function as a context manager is to use the function decorator @contextmanager
. A decorator is a function that takes another function as an input, extends its functionality/modifies its behavior, and returns another function as an output.
We don’t have to write the separate class or __enter__()
and __exit__()
functions when using this decorator. We have to import the context manager from the contextlib
module.
We will use the @contextmanager
above our function. The yield
keyword is like the return
statement used for returning values/control.
Everything before the yield
keyword will be considered the __enter__
section, and everything after the yield
will be considered the __exit__
section.
Example Code:
from contextlib import contextmanager
@contextmanager
def MyContextManager():
print("In enter method")
yield
print("In exit method")
with MyContextManager() as m:
print("With block")
Output:
In enter method
With block
In exit method
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn