Python Contextlib
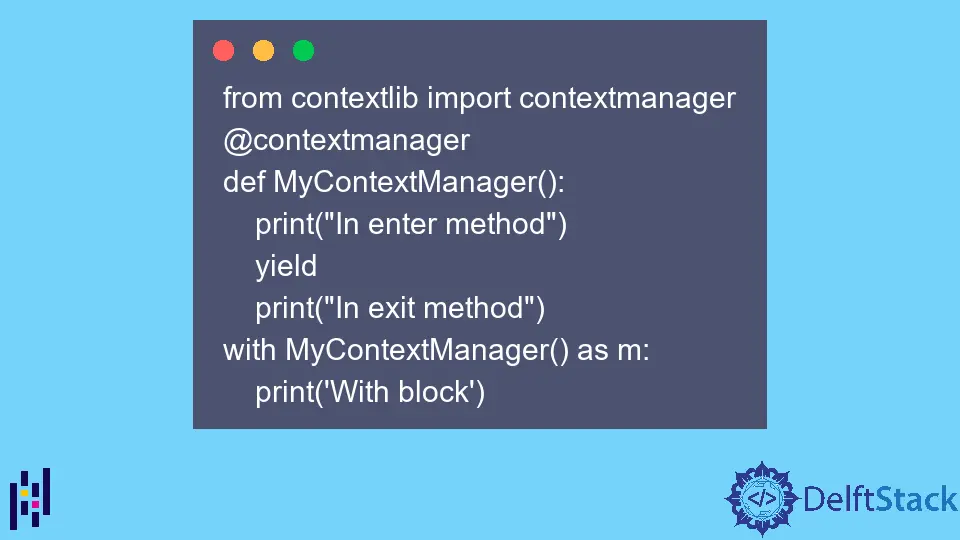
有時,我們會使用一些資源,例如檔案。當我們完成它的工作時,我們開啟它並忘記關閉它。
這是一種不好的編碼習慣,當開啟太多檔案時會產生問題。即使我們不再需要這些資源,該程式也可能無限期地獲取它們。
這種情況會導致記憶體洩漏。為了自動處理這些事情,Python 有上下文管理器。
他們負責管理資源。contextlib
是一個 Python 庫,它使用 with
關鍵字提供資源管理實用程式。
在 Python 中使用 with
語句作為上下文管理器
在 Python 中,with
語句管理資源並處理異常。所以它作為一個上下文管理器工作。
當程式碼到達 with
語句時,它會臨時分配資源。當 with
語句塊完成其執行時,先前獲取的資源將被釋放。
在下面的程式碼中,我們建立並開啟了一個檔案。當控制元件脫離 with
塊時,with
塊中獲取的資源將被釋放。
示例程式碼:
with open("file.txt", "w+") as f:
print("File Opened")
輸出:
File Opened
我們也可以在我們的程式中包含上下文管理器功能。為此,我們的類必須有方法 __enter__()
和 __exit__()
。
為了理解上下文管理器的流程,我們使用 print
語句執行了以下程式碼,該語句在控制轉移到相應塊時執行。
示例程式碼:
class MyContextManager:
def __init__(self):
print("In init method")
def __enter__(self):
print("In enter method")
def __exit__(self, exc_type, exc_value, exc_traceback):
print("In exit method")
with MyContextManager() as m:
print("With block")
輸出:
In init method
In enter method
With block
In exit method
在 Python 中使用函式裝飾器@contextmanager
另一種將函式作為上下文管理器的方法是使用函式裝飾器@contextmanager
。裝飾器是一個函式,它接受另一個函式作為輸入,擴充套件它的功能/修改它的行為,並返回另一個函式作為輸出。
使用這個裝飾器時,我們不必編寫單獨的類或 __enter__()
和 __exit__()
函式。我們必須從 contextlib
模組匯入上下文管理器。
我們將在函式上方使用@contextmanager
。yield
關鍵字類似於用於返回值/控制的 return
語句。
yield
關鍵字之前的所有內容都將被視為 __enter__
部分,而 yield
之後的所有內容將被視為 __exit__
部分。
示例程式碼:
from contextlib import contextmanager
@contextmanager
def MyContextManager():
print("In enter method")
yield
print("In exit method")
with MyContextManager() as m:
print("With block")
輸出:
In enter method
With block
In exit method
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn