How to Rename Specific DataFrame Columns in Pandas
-
Use the
rename()
Method to Rename a DataFrame Column in Pandas -
Use the
rename()
Method to Rename Multiple DataFrame Columns in Pandas - Use the Lambda Function to Rename DataFrame Columns in Pandas
-
Use the
set_axis()
Function to Rename DataFrame Columns in Pandas -
Use
columns.str.replace()
Function to Replace Specific Texts of Column Names in Pandas - Rename Columns by Passing the Updated List of Column Names in Pandas
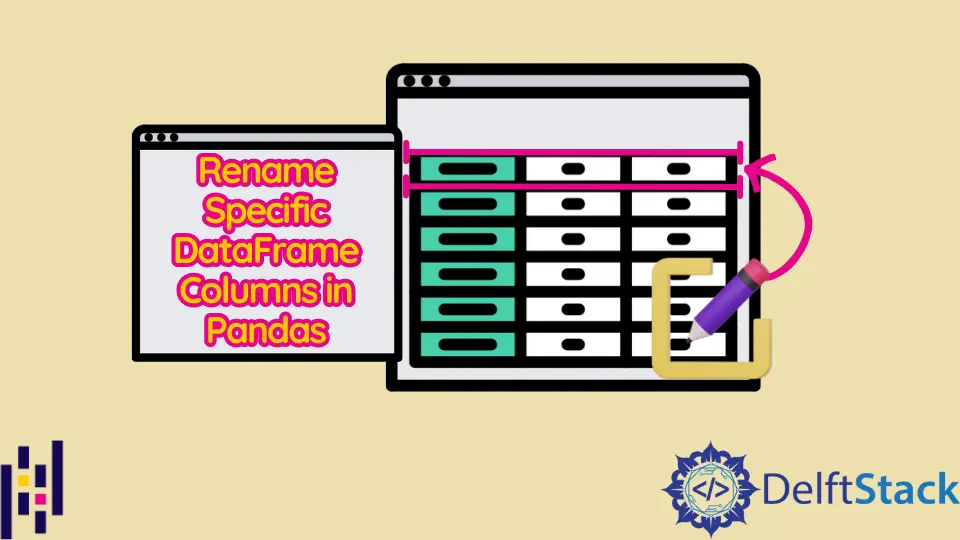
The rectangular grid where the data is stored in rows and columns in Python is known as a Pandas dataframe. Each row represents a measurement of a single instance, and each column is a data vector for a single attribute or variable.
Dataframe rows can have homogeneous or heterogeneous data across any given row, but each dataframe column contains homogeneous data throughout any given column. Unlike a two-dimensional array, a dataframe in Pandas has labeled axes.
Use the rename()
Method to Rename a DataFrame Column in Pandas
The Pandas dataframe columns and indexes can be renamed using the rename()
method. For clarity, keyword arguments are advised.
Depending on the values in the dictionary, we may use this method to rename a single column or many columns.
Example Code:
import pandas as pd
d1 = {"Names": ["Harry", "Petter", "Daniel", "Ron"], "ID": [1, 2, 3, 4]}
df = pd.DataFrame(d1)
display(df)
# rename columns
df1 = df.rename(columns={"Names": "Student Names"})
display("After renaming the columns:", df1)
Output:
Use the rename()
Method to Rename Multiple DataFrame Columns in Pandas
In the below example, it is shown that we can rename multiple columns at a time. The id
column is renamed with Roll No
, and Student Names
replace Names
.
Example Code:
import pandas as pd
df1 = pd.DataFrame(
{"id": ["ID1", "ID2", "ID3", "ID4"], "Names": ["Harry", "Petter", "Daniel", "Ron"]}
)
display(df1)
df = pd.DataFrame(df1)
df1.rename({"id": "Roll No", "Names": "Student Names"}, axis="columns", inplace=True)
display(df1)
Output:
Use the Lambda Function to Rename DataFrame Columns in Pandas
The lambda function is a straightforward anonymous function that only supports one expression but accepts an unlimited number of arguments. We may utilize it if we need to change all the columns simultaneously.
It is helpful if there are several columns, and renaming them using a list or dictionary is difficult. In the example below, we added a colon (:
) at the end of every column name using the lambda function.
Example Code:
import pandas as pd
df1 = pd.DataFrame(
{"id": ["ID1", "ID2", "ID3", "ID4"], "Names": ["Harry", "Petter", "Daniel", "Ron"]}
)
display(df1)
frames = [df1, df2]
df = df1.rename(columns=lambda x: "Student " + x)
display(df)
Output:
Use the set_axis()
Function to Rename DataFrame Columns in Pandas
In the below example, we’ll use the set_axis()
method to rename the column’s name. As an argument, we’ll supply the new column name and the axis that has to have its name changed.
import pandas as pd
df1 = pd.DataFrame(
{"id": ["ID1", "ID2", "ID3", "ID4"], "Names": ["Harry", "Petter", "Daniel", "Ron"]}
)
display(df1)
df1.set_axis(["A", "B"], axis="columns", inplace=True)
display(df1)
Output:
Use columns.str.replace()
Function to Replace Specific Texts of Column Names in Pandas
In this example, we’ll use the replace()
function to rename the column’s name. As an argument for the column, we’ll supply the old name and the new one.
import pandas as pd
df1 = pd.DataFrame(
{
"id": ["ID1", "ID2", "ID3", "ID4"],
"Names": ["Harry", "Petter", "Daniel", "Ron"],
"Department": ["English", "Science", "General Knowledge", "Arts"],
}
)
display(df1)
df1.set_axis(["A", "B", "C"], axis="columns", inplace=True)
df1.columns = df1.columns.str.replace("Id", "Rollno")
df1.columns = df1.columns.str.replace("Names", "Students")
df1.columns = df1.columns.str.replace("Department", "Program")
df1.head()
display(df1)
Output:
Rename Columns by Passing the Updated List of Column Names in Pandas
The columns can also be changed by directly changing the names of the columns by setting a list having the new names to the columns property of the Dataframe object. The drawback of this approach is that we must offer names for all of them, even if we only wish to rename part of the columns.
Example Code:
import pandas as pd
df1 = pd.DataFrame(
{
"id": ["ID1", "ID2", "ID3", "ID4"],
"Names": ["Harry", "Petter", "Daniel", "Ron"],
"Department": ["English", "Science", "General Knowledge", "Arts"],
}
)
display(df1)
df1.columns = ["Rollno", "Students", "Program"]
display(df1)
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn