How to Concatenate Two DataFrames in Pandas Python
- Concatenate Two DataFrames in Pandas Python
-
Use the
concat()
Function to Concatenate Two DataFrames in Pandas Python - Combine Two Pandas DataFrames Using a Common Field
-
Use the
append()
Method to Concatenate Two DataFrames in Pandas Python
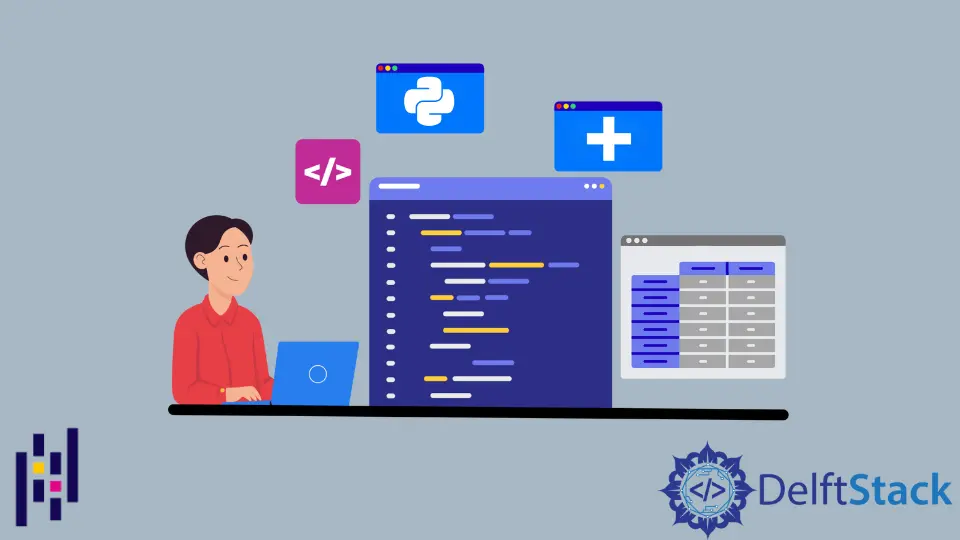
Series, which have a list-like structure, and data frames, which have a tabular structure, are two new types of objects for storing data introduced by Pandas. Pandas data frames are among the most useful data structures in any toolkit.
You might consider a dataframe as a 2-dimensional labeled data structure containing columns that may be of multiple types, similar to an SQL table or a dict of series objects. It is often the Pandas item that is utilized the most.
Concatenate Two DataFrames in Pandas Python
With the help of Pandas, it is possible to quickly combine series or data frames with different types of set logic for the indexes and relational algebra capabilities for join and merge-type operations. Additionally, Pandas offer tools for comparing two series or data frames and highlighting their differences using the Pandas concat()
technique; two or more data frames may be joined together.
The Pandas concat()
function joins data frames across rows or columns. We can combine many data frames by concatenating them along rows or columns.
Use the concat()
Function to Concatenate Two DataFrames in Pandas Python
The concat()
is a function in Pandas that appends columns or rows from one dataframe to another. It combines data frames as well as series.
In the following code, we have created two data frames and combined them using the concat()
function. We have passed the two data frames as a list to the concat()
function.
Example Code:
import pandas as pd
df1 = pd.DataFrame(
{"id": ["ID1", "ID2", "ID3", "!D4"], "Names": ["Harry", "Petter", "Daniel", "Ron"]}
)
df2 = pd.DataFrame(
{"id": ["ID5", "ID6", "ID7", "ID8"], "Names": ["Kelvin", "Henry", "Emma", "Sofia"]}
)
display(" First DataFrame: ", df1)
display(" Second DataFrame: ", df2)
frames = [df1, df2]
d = pd.concat(frames)
display(d)
Output:
Combine Two Pandas DataFrames Using a Common Field
To concatenate them, we added our data frames to one another, either vertically or side by side. Utilizing columns from each dataset with similar values is another method of combining data frames (a unique common id).
Joining is the process of combining data frames utilizing a shared field. The join
key(s) refers to the shared values columns.
When one dataframe contains a lookup table containing additional data that we wish to incorporate into the other, joining data frames in this fashion is frequently helpful.
When joining several data frames, you have an option of how to handle the different axes (other than the one being concatenated).
To show you how this can be used, take the union of them all, join='outer'
. Consider the intersection with join='inner'
because it causes no information loss and is the default choice.
Example Code:
import pandas as pd
df1 = pd.DataFrame(
{"id": ["ID1", "ID2", "ID3", "!D4"], "Names": ["Harry", "Petter", "Daniel", "Ron"]}
)
df2 = pd.DataFrame(
{"id": ["ID5", "ID6", "ID7", "ID8"], "Names": ["Kelvin", "Henry", "Emma", "Sofia"]}
)
print(" First DataFrame: ", df1)
print(" Second DataFrame: ", df2)
frames = [df1, df2]
d = pd.concat([df1, df2], axis=1, join="inner")
print(d)
Output:
Use the append()
Method to Concatenate Two DataFrames in Pandas Python
The append()
method can be used to concatenate data frames, as append()
is a useful shortcut instance method on series and dataframe. This technique existed before concat()
.
Example Code:
import pandas as pd
import pandas as pd
df1 = pd.DataFrame(
{"id": ["ID1", "ID2", "ID3", "!D4"], "Names": ["Harry", "Petter", "Daniel", "Ron"]}
)
df2 = pd.DataFrame(
{"id": ["ID5", "ID6", "ID7", "ID8"], "Names": ["Kelvin", "Henry", "Emma", "Sofia"]}
)
frames = [df1, df2]
d = pd.concat([df1, df2], axis=1, join="inner")
result = df1.append(df2)
display(result)
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn