How to Append to Empty Dataframe in Pandas
- Make an Empty Dataframe and Append Rows and Columns in Pandas
- Append Rows to an Empty Dataframe Having Columns in Pandas
-
Create an Empty Dataframe Including Columns With Indices and Append Rows Using the
.loc()
Function in Pandas
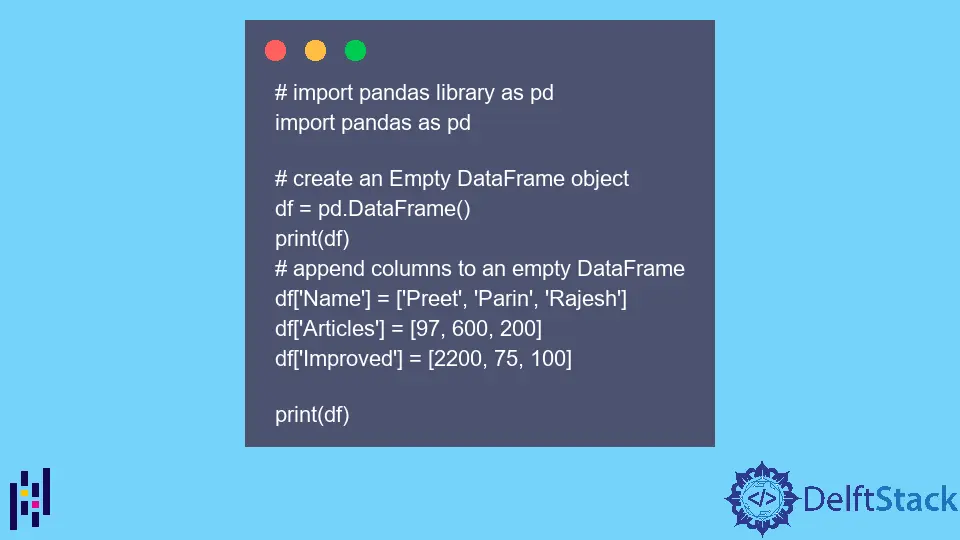
As we have learned before, Pandas in Python is an open-source module that we can use for data analysis and make machine learning models. It is used along with one more package known as Numpy
as they go hand in hand to support multidimensional arrays.
Many data science modules can work with Pandas inside the Python ecosystem. We will learn different operations for appending to an empty dataframe in Pandas.
A dataframe is two-dimensional and potential heterogeneous data in the form of a table.
This tutorial teaches how to append rows and columns in an empty dataframe in Pandas using Python. We will look at three different methods to add data to an empty dataframe since there are multiple ways.
Make an Empty Dataframe and Append Rows and Columns in Pandas
Below is the code for importing the required package, making an empty dataframe and appending columns.
# import pandas library as pd
import pandas as pd
# create an Empty DataFrame object
df = pd.DataFrame()
print(df)
# append columns to an empty DataFrame
df["Name"] = ["Preet", "Parin", "Rajesh"]
df["Articles"] = [97, 600, 200]
df["Improved"] = [2200, 75, 100]
print(df)
The output for the above code snippet is as follows.
Empty DataFrame
Columns: []
Index: []
Name Articles Improved
0 Preet 97 2200
1 Parin 600 75
2 Rajesh 200 100
Before we appended the columns and rows, the dataframe was empty. So printing the empty dataframe gave us the output Empty DataFrame, Columns: [], Index: []
as an output, which was expected since the data is null.
Append Rows to an Empty Dataframe Having Columns in Pandas
In this method, the dataframe would be empty, but there will be predefined column names, where our only task is to insert the data in the rows below it.
Below is the code for the above method where we initially import the library, Pandas, create a dataframe with columns in it and then append the values in the form of rows.
# import pandas library as pd
import pandas as pd
# create an Empty DataFrame
# object With column names only
df = pd.DataFrame(columns=["Name", "Articles", "Improved"])
print(df)
# append rows to an empty DataFrame
df = df.append({"Name": "Preet", "Articles": 97, "Improved": 2200}, ignore_index=True)
df = df.append({"Name": "Parin", "Articles": 30, "Improved": 50}, ignore_index=True)
df = df.append({"Name": "Rajesh", "Articles": 17, "Improved": 220}, ignore_index=True)
print(df)
The code will give us the following output.
Empty DataFrame
Columns: [Name, Articles, Improved]
Index: []
Name Articles Improved
0 Preet 97 2200
1 Parin 30 50
2 Rajesh 17 220
As we can observe, since we had already added the names of the columns in the dataframe, the output consists of Columns: [Name, Articles, Improved]
, which is the columns’ names in an array.
The following output is because of the .append()
function that we have used.
Create an Empty Dataframe Including Columns With Indices and Append Rows Using the .loc()
Function in Pandas
The .loc()
method in Pandas helps the user retrieve the values from the dataframe with ease without any complexities. One can access the values inside a particular row and columns based on the index value passed in the function.
In this method, we would create an empty dataframe and column names. Each column will be recognized using an index to access it.
Followed by which we will append the row one after the other.
The code that justifies this method is given below.
# import pandas library as pd
import pandas as pd
# create an Empty DataFrame object With
# column names and indices
df = pd.DataFrame(columns=["Name", "Articles", "Improved"], index=["a", "b", "c"])
print("Empty DataFrame With NaN values : \n\n", df)
# adding rows to an empty
# dataframe at existing index
df.loc["a"] = ["Preet", 50, 100]
df.loc["b"] = ["Parin", 60, 120]
df.loc["c"] = ["Rajesh", 30, 60]
print(df)
The output of the code given above is as follows.
Empty DataFrame With NaN values :
Name Articles Improved
a NaN NaN NaN
b NaN NaN NaN
c NaN NaN NaN
Name Articles Improved
a Preet 50 100
b Parin 60 120
c Rajesh 30 60
As we observe, the NaN
values in the first table are because we have mentioned the indices, but the values remain empty.
Providing the number of indices bounds the table to that many values for that particular instance. Increasing the number of indices allows more values to be inserted.
So, in this tutorial, we have learned about three different methods to append values to the dataframe in Pandas.