How to Append a Column in Pandas DataFrame
- Method 1: Using Direct Assignment
-
Method 2: Using the
assign()
Method -
Method 3: Using
insert()
Method -
Method 4: Using
concat()
- Conclusion
- FAQ
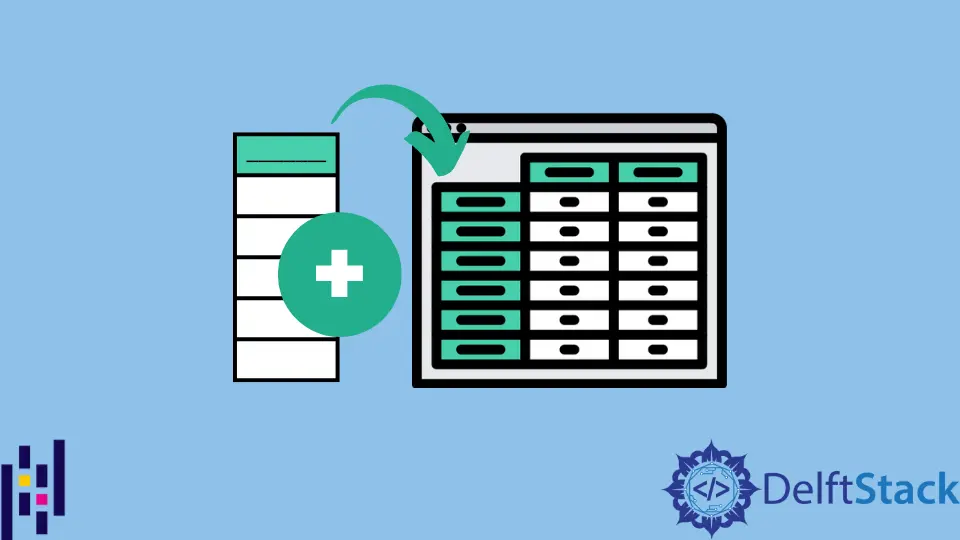
Appending a column to a Pandas DataFrame is a common task that can significantly enhance data manipulation and analysis. Whether you’re adding new calculated values, merging datasets, or simply organizing your data better, knowing how to append a column effectively is crucial.
In this tutorial, we’ll explore various methods to append a column in a Pandas DataFrame using Python. From basic assignments to more complex operations, you’ll learn how to expand your DataFrame with ease. Let’s dive into the world of Pandas and discover how to make your data work for you!
Method 1: Using Direct Assignment
One of the simplest ways to append a column to a Pandas DataFrame is through direct assignment. This method allows you to create a new column by assigning values to it directly. It’s straightforward and efficient, making it ideal for adding static data or calculations based on existing columns.
Here’s how you can do it:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
df = pd.DataFrame(data)
df['City'] = ['New York', 'Los Angeles', 'Chicago']
print(df)
Output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
In this example, we first create a DataFrame named df
with two columns: ‘Name’ and ‘Age’. Next, we append a new column called ‘City’ by directly assigning a list of city names to it. This method is particularly useful when you have a predefined list of values that correspond to the DataFrame’s existing rows. It’s quick, simple, and effective for straightforward data additions.
Method 2: Using the assign()
Method
The assign()
method is another elegant way to append a column to a DataFrame. This method returns a new DataFrame with the added column while keeping the original DataFrame unchanged. This can be particularly useful when you want to maintain immutability in your data processing workflow.
Let’s look at an example:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
df = pd.DataFrame(data)
new_df = df.assign(Country=['USA', 'USA', 'USA'])
print(new_df)
Output:
Name Age Country
0 Alice 25 USA
1 Bob 30 USA
2 Charlie 35 USA
In this code, we again start by creating a DataFrame df
. We then use the assign()
method to create a new DataFrame new_df
, which includes an additional column called ‘Country’. The original DataFrame df
remains unchanged. This method is particularly useful for chaining operations in a clean and readable manner, making your code more modular and easier to maintain.
Method 3: Using insert()
Method
If you want to append a column at a specific position in the DataFrame rather than at the end, the insert()
method is the way to go. This method allows you to specify the index where the new column should be inserted, giving you more control over the DataFrame’s structure.
Here’s how to use it:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
df = pd.DataFrame(data)
df.insert(1, 'Gender', ['F', 'M', 'M'])
print(df)
Output:
Name Gender Age
0 Alice F 25
1 Bob M 30
2 Charlie M 35
In this example, we create a DataFrame df
once again. We then use the insert()
method to add a new column ‘Gender’ at index 1. This means that ‘Gender’ will be placed between the ‘Name’ and ‘Age’ columns. The flexibility of this method allows you to maintain a specific order in your DataFrame, which can be crucial for readability and data analysis.
Method 4: Using concat()
When you need to append a column from another DataFrame, the concat()
function is your best friend. This method is especially useful when you are merging datasets or when the new column is derived from a different DataFrame but still relates to the original one.
Let’s see it in action:
import pandas as pd
data1 = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
data2 = {
'City': ['New York', 'Los Angeles', 'Chicago']
}
df1 = pd.DataFrame(data1)
df2 = pd.DataFrame(data2)
df_combined = pd.concat([df1, df2], axis=1)
print(df_combined)
Output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
In this example, we create two DataFrames: df1
and df2
. The first DataFrame contains names and ages, while the second one contains city names. By using pd.concat()
, we can append the columns of df2
to df1
along the column axis (axis=1). This method is powerful for combining datasets and is particularly useful in data analysis where you often work with multiple DataFrames.
Conclusion
Appending a column to a Pandas DataFrame is a fundamental skill that can significantly enhance your data manipulation capabilities. Whether you choose to use direct assignment, the assign()
method, the insert()
method, or concat()
, each method offers unique advantages depending on your specific needs. By mastering these techniques, you can ensure that your data is well-organized and ready for analysis. So go ahead, experiment with these methods, and make your data work for you!
FAQ
-
How can I append multiple columns to a DataFrame?
You can append multiple columns by using direct assignment with a DataFrame or by using theassign()
method multiple times. -
Can I append a column with different lengths to a DataFrame?
No, all columns in a DataFrame must have the same number of rows. If you try to append a column with a different length, you will get an error. -
What happens to the original DataFrame when using the
assign()
method?
The original DataFrame remains unchanged. Theassign()
method returns a new DataFrame with the added column. -
Is it possible to append a column based on a condition?
Yes, you can create a new column based on conditions applied to existing columns using methods likenp.where()
orapply()
. -
How do I remove a column after appending it?
You can remove a column using thedrop()
method, specifying the column name and settingaxis=1
.