How to Add Column With a Constant Value in Pandas
-
Use an
in-place
Assignment to Add a Column With Constant Value in Pandas -
Use the
loc
Method to Add a Column With Constant Value in Pandas -
Use the
assign()
Function to Add a Column With Constant Value in Pandas -
Use the
fromkeys()
Method to Add a Column With Constant Value in Pandas -
Use the
series()
Method to Add a Column With Constant Value in Pandas -
Use the
apply()
Function to Add a Column With a Constant Value in Pandas
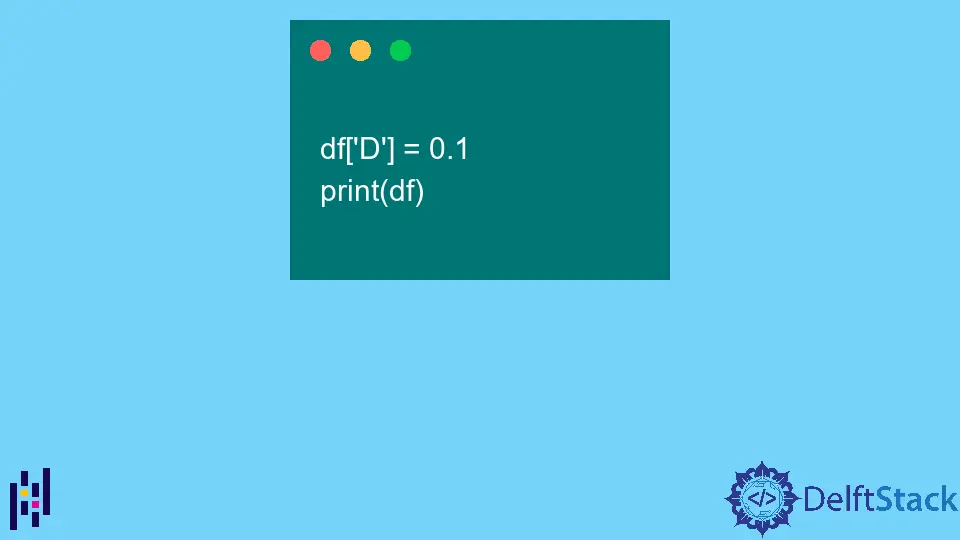
Often, we load data from external sources such as CSV
, JSON
, or populate based on the numpy
library. But more than that, we need to create a new data column with values to work with.
We can use different approaches with varying complexities for specific use cases to achieve this. This article will discuss six(6) ways to add columns with a constant value in Pandas.
Use an in-place
Assignment to Add a Column With Constant Value in Pandas
The simplest way to add a column with a constant value in pandas is to use the typical assignment operator (=
). In using it, we assign the constant value to a new column name using the square bracket
notation as below.
DataFrame["column name"] = value
Let’s illustrate this by creating a randomized data frame (with three columns - A
, B
, and C
) using numpy
and then adding a new column (named D
) with a constant value of 0.1
.
Code:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.randn(3, 3), columns=list("ABC"), index=[1, 2, 3])
print(df)
Output:
A B C
1 0.466388 -0.626593 0.127939
2 -0.523171 0.778552 -1.056575
3 1.669556 -0.254253 0.855463
Now, to add the new D
column with the 0.1
constant value, the code below is sufficient.
Code:
df["D"] = 0.1
print(df)
Output:
A B C D
1 -0.099164 0.100860 0.070556 0.1
2 -0.693606 -0.226484 0.032315 0.1
3 0.831928 -0.545919 1.759017 0.1
Use the loc
Method to Add a Column With Constant Value in Pandas
The loc
method is another approach we can use to add a column with a constant value in pandas. The loc
method allows us to index a part of the DataFrame by row and column names, so we can select a new column (like we did with square notation
in the previous section) using the loc
method and assign a new column name.
Let’s add a new column name - E
- with a constant value of 0.2
using the loc
method.
Code:
df.loc[:, "E"] = 0.2
print(df)
Output:
A B C E
1 -0.233729 -0.343784 -0.354388 0.2
2 -0.529278 -0.239034 0.791784 0.2
3 -0.498778 0.165311 2.983666 0.2
The :
specifies that all the rows are indexed, the E
column is specified, and then the value 0.2
is assigned to it.
Use the assign()
Function to Add a Column With Constant Value in Pandas
Another approach to achieve the operation to add a column with a constant value in Pandas is the assign()
function. The assign()
function is a specific function designed to assign new columns to a Pandas DataFrame where the column’s name is passed as the argument of the function.
Using this, we will create a new column - F
- with an assigned value - 0.3
in the df
DataFrame we have used in the article.
Code:
df = df.assign(F=0.3)
print(df)
Output:
A B C D E F
1 1.474679 -0.369649 0.258509 0.1 0.2 0.3
2 -0.887326 -0.097165 0.394489 0.1 0.2 0.3
3 -1.763712 0.631679 -0.667194 0.1 0.2 0.3
Use the fromkeys()
Method to Add a Column With Constant Value in Pandas
Still, on the assign()
function, if we want to add multiple columns with a constant value in Pandas, we can use the assign()
function and the dict.fromkeys()
functions. The fromkeys()
function takes two arguments.
The first argument is the list that contains the column names, and the second argument is the constant value we want in the columns we passed. The fromkeys()
returns a dictionary created based on the first argument (list) and the second argument(value) for a key-pair relationship.
Afterward, the dictionary is parsed to the assign()
function, and the **
serves as an idiom that allows an arbitrary number of arguments(the dictionary) to the function.
Code:
newColumns = ["G", "H", "I"]
df = df.assign(**dict.fromkeys(newColumns, 0.4))
print(df)
Output:
A B C D E F G H I
1 1.474679 -0.369649 0.258509 0.1 0.2 0.3 0.4 0.4 0.4
2 -0.887326 -0.097165 0.394489 0.1 0.2 0.3 0.4 0.4 0.4
3 -1.763712 0.631679 -0.667194 0.1 0.2 0.3 0.4 0.4 0.4
Instead of using the list and the fromkeys()
function, we can specify which constant value we want each column to have by creating a dictionary directly and using the assign()
function. This way, we have different constant values for each column.
Code:
newDictColumns = {"J": 0.5, "K": 0.6, "L": 0.7}
df = df.assign(**newDictColumns)
print(df)
Output:
A B C D E F G H I J K L
1 1.474679 -0.369649 0.258509 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
2 -0.887326 -0.097165 0.394489 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
3 -1.763712 0.631679 -0.667194 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
Use the series()
Method to Add a Column With Constant Value in Pandas
The series()
function allows us to create a one-dimensional array with axis labels. To add a new column, we use the list comprehension to loop through the DataFrame index
and add the constant value.
Let’s add an M
column by passing the list comprehension to the series()
function.
Code:
df["M"] = pd.Series([0.8 for x in range(len(df.index) + 1)])
print(df)
Output:
A B C D E F G H I J K L \
1 1.474679 -0.369649 0.258509 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
2 -0.887326 -0.097165 0.394489 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
3 -1.763712 0.631679 -0.667194 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
M
1 0.8
2 0.8
3 0.8
Use the apply()
Function to Add a Column With a Constant Value in Pandas
We can use the apply()
function to add a column with a constant value in Pandas by passing a lambda
function which assigns the value to each cell. Afterward, the result of the apply()
function is assigned to the square notation
of the DataFrame column.
Let’s see this in action by creating an N
column with a constant value of 0.9
using a lambda
function within the apply()
function.
Code:
df["N"] = df.apply(lambda x: 0.9, axis=1)
print(df)
Output:
A B C D E F G H I J K L \
1 1.474679 -0.369649 0.258509 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
2 -0.887326 -0.097165 0.394489 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
3 -1.763712 0.631679 -0.667194 0.1 0.2 0.3 0.4 0.4 0.4 0.5 0.6 0.7
M N
1 0.8 0.9
2 0.8 0.9
3 0.8 0.9
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn