How to Multiply Columns by a Scalar in Pandas
- Use In-Place Assignment to Multiply Columns by a Scalar in Pandas
-
Use the
multiply
Function to Multiply Columns by a Scalar in Pandas -
Use the
apply
Function to Multiply Columns by a Scalar in Pandas -
Use the
loc
Operator to Multiply Columns by a Scalar in Pandas -
Use the
iloc
Operator to Multiply Columns by a Scalar in Pandas
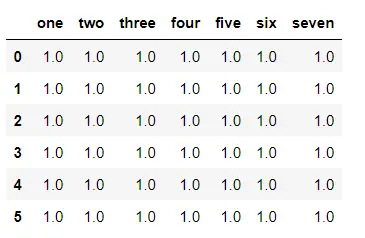
We use Pandas for many dataframe operations, from populating data to cleaning the said data. The Pandas dataframe offers methods for data and mathematical operations using Python.
Different mathematical operations might seem less intuitive to carry out, but with Python, they are a lot easier than you think. One such operation is multiplying Pandas columns by scalar values.
This article will show five ways to multiply columns by a scalar in Pandas within different complexities.
Use In-Place Assignment to Multiply Columns by a Scalar in Pandas
Before we go into how to approach multiplying columns by scalar values in pandas using in-place assignment, let’s showcase what we intend to achieve.
We have a dataframe with seven columns.
Now, we want to multiply a column (one
) by a scalar value (5).
So, how do we achieve that? The first approach is using in-place assignment, an operation where we, in addition, to assigning a value, operate on the value.
Using in-place assignment, we overwrite the value of the first operand variable with the result of the operation performed on the value of the operand. Visually, x *= 3
is equivalent to x = x * 3
where the value held by the variable x
is multiplied by 3
and then assigned to the same variable x
.
To illustrate this, we can create a Pandas dataframe of 6 rows and 7 columns populated with ones
which assigned column names and perform the in-place assignment on the one
column.
import pandas as pd
import numpy as np
df = pd.DataFrame(
np.ones((6, 7)), columns=["one", "two", "three", "four", "five", "six", "seven"]
)
print(df)
Output:
one two three four five six seven
0 1.0 1.0 1.0 1.0 1.0 1.0 1.0
1 1.0 1.0 1.0 1.0 1.0 1.0 1.0
2 1.0 1.0 1.0 1.0 1.0 1.0 1.0
3 1.0 1.0 1.0 1.0 1.0 1.0 1.0
4 1.0 1.0 1.0 1.0 1.0 1.0 1.0
5 1.0 1.0 1.0 1.0 1.0 1.0 1.0
To use in-place assignment, we have to select the Pandas column we want to multiply by the scalar value using either the dot notation or the bracket notation. Using the bracket notation, we select the one
column.
df["one"] *= 5
print(df)
Output:
one two three four five six seven
0 5.0 1.0 1.0 1.0 1.0 1.0 1.0
1 5.0 1.0 1.0 1.0 1.0 1.0 1.0
2 5.0 1.0 1.0 1.0 1.0 1.0 1.0
3 5.0 1.0 1.0 1.0 1.0 1.0 1.0
4 5.0 1.0 1.0 1.0 1.0 1.0 1.0
5 5.0 1.0 1.0 1.0 1.0 1.0 1.0
As you can see, the one
column has been multiplied by the scalar value (5).
Use the multiply
Function to Multiply Columns by a Scalar in Pandas
Rather than use the multiplication operator in an in-place assignment approach, you can use the multiply
method provided by Pandas. We apply the method in the column we want and pass the scalar value as the argument for the multiply
method.
To showcase the multiply
method, we will multiply the column two
by the scalar value of 7
(accessing the column two
using the dot notation).
df.two = df.two.multiply(7)
print(df)
Output:
one two three four five six seven
0 5.0 7.0 1.0 1.0 1.0 1.0 1.0
1 5.0 7.0 1.0 1.0 1.0 1.0 1.0
2 5.0 7.0 1.0 1.0 1.0 1.0 1.0
3 5.0 7.0 1.0 1.0 1.0 1.0 1.0
4 5.0 7.0 1.0 1.0 1.0 1.0 1.0
5 5.0 7.0 1.0 1.0 1.0 1.0 1.0
Use the apply
Function to Multiply Columns by a Scalar in Pandas
Similar to how we used the multiply
method, we can use the apply
method - a higher-order function - to multiply columns by a scalar in Pandas. The apply
method takes a lambda
function that will carry out an action on the cell elements of the column it’s applied on.
So, we can specify a multiplication action on the cell element using the lambda
function.
To illustrate how this works, we will multiply the three
column (from the dataframe used in earlier sections) by 9
using the apply
method.
df["three"] = df["three"].apply(lambda x: x * 9)
print(df)
Output:
one two three four five six seven
0 5.0 7.0 9.0 1.0 1.0 1.0 1.0
1 5.0 7.0 9.0 1.0 1.0 1.0 1.0
2 5.0 7.0 9.0 1.0 1.0 1.0 1.0
3 5.0 7.0 9.0 1.0 1.0 1.0 1.0
4 5.0 7.0 9.0 1.0 1.0 1.0 1.0
5 5.0 7.0 9.0 1.0 1.0 1.0 1.0
You can see that all the cells in the three
column have been multiplied by 9
based on the lambda
function. This approach can seem stressful, but it can be more functional when the value we want to multiply the column may vary based on the cell.
Use the loc
Operator to Multiply Columns by a Scalar in Pandas
Also, we can use the loc
operator to multiply the column by a scalar in Pandas. With the loc
operator, we can index a portion of a dataframe.
We can index based on rows and columns. For us, we need to index based on the column we need.
The typical syntax for the loc
operator is given below.
DataFrame.loc[rows, columns]
However, for us to access only the column, we can use the syntax below.
DataFrame.loc[:, columns]
The :
indicates that it wants all the rows. Together, the syntax above means to access all the rows in the specified column.
Now, we will use the above syntax to get the column four
and use the in-place assignment to multiply the values by 11
.
df.loc[:, "four"] *= 11
print(df)
Output:
one two three four five six seven
0 5.0 7.0 9.0 11.0 1.0 1.0 1.0
1 5.0 7.0 9.0 11.0 1.0 1.0 1.0
2 5.0 7.0 9.0 11.0 1.0 1.0 1.0
3 5.0 7.0 9.0 11.0 1.0 1.0 1.0
4 5.0 7.0 9.0 11.0 1.0 1.0 1.0
5 5.0 7.0 9.0 11.0 1.0 1.0 1.0
This seems unnecessarily long, but this approach is functional if we want to multiply multiple columns. For example, if we want to multiply columns five
and six
by 13
, we will pass a list containing the names of the column as the second argument to the loc
operator.
df.loc[:, ["five", "six"]] *= 13
print(df)
Output:
one two three four five six seven
0 5.0 7.0 9.0 11.0 13.0 13.0 1.0
1 5.0 7.0 9.0 11.0 13.0 13.0 1.0
2 5.0 7.0 9.0 11.0 13.0 13.0 1.0
3 5.0 7.0 9.0 11.0 13.0 13.0 1.0
4 5.0 7.0 9.0 11.0 13.0 13.0 1.0
5 5.0 7.0 9.0 11.0 13.0 13.0 1.0
Use the iloc
Operator to Multiply Columns by a Scalar in Pandas
Similar to the loc
operator, it accepts only integer values as the index for the values we intend to access. Unlike loc
, where we can use the column’s name, we use the column’s index value.
Also, remember that the index starts at 0 and not 1. Therefore, if we want to access column seven
and multiply by 15
, we will use the index value 6
.
df.iloc[:, 6] = df.iloc[:, 6] * 15
print(df)
Output:
one two three four five six seven
0 5.0 7.0 9.0 11.0 13.0 13.0 15.0
1 5.0 7.0 9.0 11.0 13.0 13.0 15.0
2 5.0 7.0 9.0 11.0 13.0 13.0 15.0
3 5.0 7.0 9.0 11.0 13.0 13.0 15.0
4 5.0 7.0 9.0 11.0 13.0 13.0 15.0
5 5.0 7.0 9.0 11.0 13.0 13.0 15.0
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn