How to Set Value for Particular Cell in Pandas DataFrame Using Index
-
Set Value for Particular Cell in Pandas DataFrame Using
pandas.dataframe.at
Method -
Set Value for Particular Cell in Pandas DataFrame Using
Dataframe.set_value()
Method -
Set Value for Particular Cell in Pandas DataFrame Using
Dataframe.loc
Method
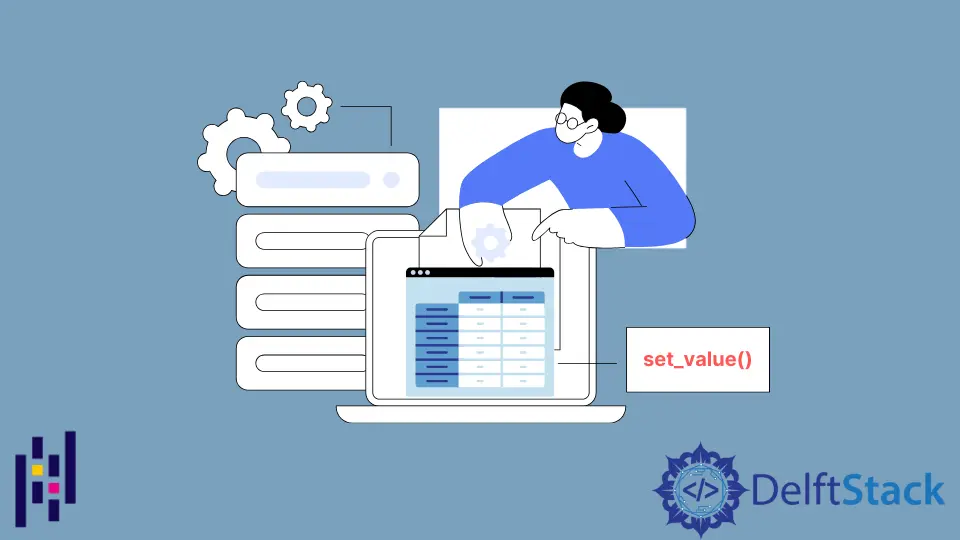
Pandas is a data-centric python package that makes data analysis in Python easy and coherent.
In this article, we will look into different methods of accessing and setting values for a particular cell in pandas DataFrame
data structure using an index.
Set Value for Particular Cell in Pandas DataFrame Using pandas.dataframe.at
Method
pandas.dataframe.at
method is primarily used when we need to set a single value in a DataFrame
.
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.at[0, "Col1"] = 99
sample_df.at[1, "Col2"] = 99
sample_df.at[2, "Col3"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
Output:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
As you might notice, while accessing the cell we have specified index and column as .at[0, 'Col1']
among which the first parameter is the index, and the second is the column.
If you leave the column and only specify the index, all values for that index will be modified.
Set Value for Particular Cell in Pandas DataFrame Using Dataframe.set_value()
Method
Another alternative is the Dataframe.set_value()
method. This is much similar to the previous method and accesses one value at a time, but with a slight difference in syntax.
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.set_value(0, "Col1", 99)
sample_df.set_value(1, "Col2", 99)
sample_df.set_value(2, "Col3", 99)
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
Output:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
Set Value for Particular Cell in Pandas DataFrame Using Dataframe.loc
Method
Another viable method to set a particular cell with a slight difference in syntax is the dataframe.loc
method.
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.loc[0, "Col3"] = 99
sample_df.loc[1, "Col2"] = 99
sample_df.loc[2, "Col1"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
Output:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 10 20 99
1 11 99 31
2 99 25 35
All the above-mentioned methods in the article are convenient ways to modify or set a particular cell in pandas DataFrame
, with minute differences in syntax and specification.