インデックスを使用して Pandas DataFrame の特定のセルに値を設定する方法
Puneet Dobhal
2023年1月30日
-
pandas.dataframe.at
メソッドを使用して、pandas DataFrame の特定のセルに値を設定する -
Dataframe.set_value()
メソッドを使用して、pandas DataFrame の特定のセルの値を設定する -
Dataframe.loc
メソッドを使用して、pandas DataFrame の特定のセルに値を設定する
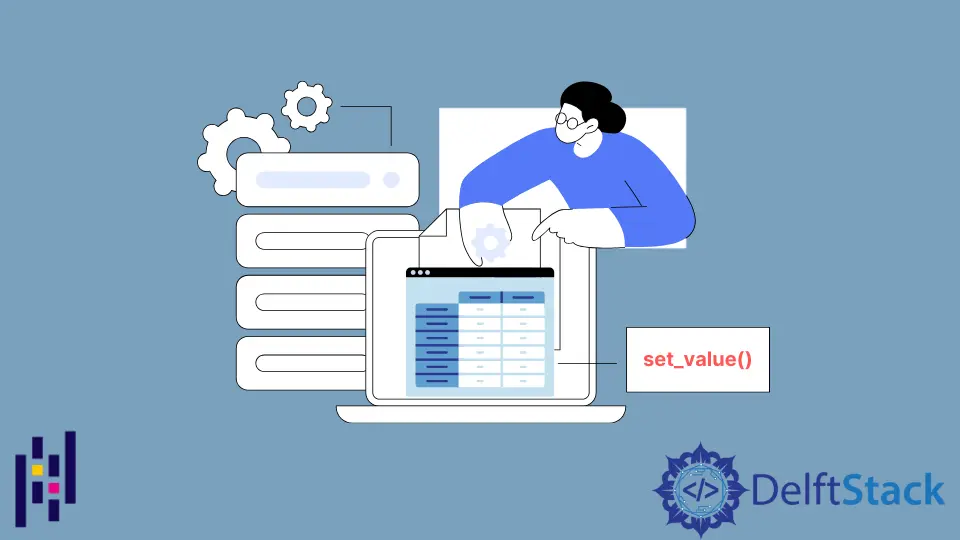
Pandas は、Python でのデータ分析を簡単かつ一貫性のあるものにするデータ中心の Python パッケージです。この記事では、インデックスを使用して Pandas の DataFrame
データ構造内の特定のセルにアクセスして値を設定するさまざまな方法について説明します。
pandas.dataframe.at
メソッドを使用して、pandas DataFrame の特定のセルに値を設定する
pandas.dataframe.at
メソッドは、DataFrame
に単一の値を設定する必要があるときに主に使用されます。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.at[0, "Col1"] = 99
sample_df.at[1, "Col2"] = 99
sample_df.at[2, "Col3"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
出力:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
お気づきかもしれませんが、セルにアクセスするときに、インデックスと列を .at[0, 'Col1']
として指定しました。最初のパラメータはインデックスで、2 番目のパラメータは列です。
列を残してインデックスのみを指定すると、そのインデックスのすべての値が変更されます。
Dataframe.set_value()
メソッドを使用して、pandas DataFrame の特定のセルの値を設定する
もう一つの選択肢は Dataframe.set_value()
メソッドです。これは前の方法とよく似ており、一度に 1つの値にアクセスしますが、構文が少し異なります。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.set_value(0, "Col1", 99)
sample_df.set_value(1, "Col2", 99)
sample_df.set_value(2, "Col3", 99)
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
出力:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 99 20 30
1 11 99 31
2 15 25 99
Dataframe.loc
メソッドを使用して、pandas DataFrame の特定のセルに値を設定する
構文にわずかな違いがある特定のセルを設定するもう 1つの実行可能な方法は、dataframe.loc
メソッドです。
import pandas as pd
sample_df = pd.DataFrame(
[[10, 20, 30], [11, 21, 31], [15, 25, 35]],
index=[0, 1, 2],
columns=["Col1", "Col2", "Col3"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(sample_df))
sample_df.loc[0, "Col3"] = 99
sample_df.loc[1, "Col2"] = 99
sample_df.loc[2, "Col1"] = 99
print "\nModified DataFrame"
print (pd.DataFrame(sample_df))
出力:
Original DataFrame
Col1 Col2 Col3
0 10 20 30
1 11 21 31
2 15 25 35
Modified DataFrame
Col1 Col2 Col3
0 10 20 99
1 11 99 31
2 99 25 35
記事で説明した上記のすべてのメソッドは、Pandas DataFrame
の特定のセルを変更または設定する便利な方法ですが、構文と仕様にわずかな違いがあります。