How to Rename Columns in Pandas DataFrame
-
Rename Columns in Pandas
DataFrame
Using theDataFrame.columns
Method -
Rename Columns in Pandas
DataFrame
UsingDataFrame.rename()
Method -
Rename Columns in Pandas
DataFrame
UsingDataFrame.set_axis()
Method
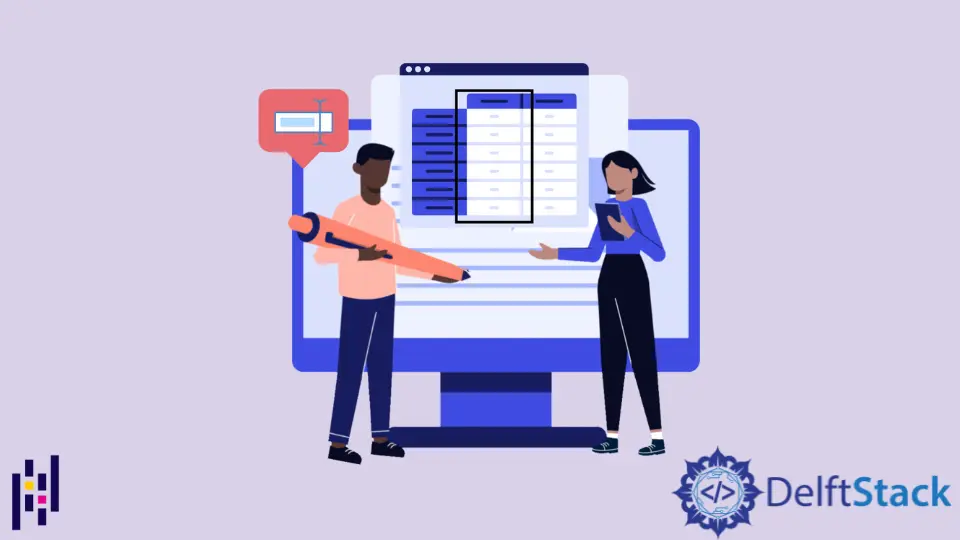
Often we need to rename a column in Pandas when performing data analysis. This article will introduce different methods to rename Pandas column names in Pandas DataFrame
.
Rename Columns in Pandas DataFrame
Using the DataFrame.columns
Method
This method is pretty straightforward and lets you rename columns directly. We can assign a list of new column names using DataFrame.columns
attribute as follows:
import pandas as pd
example_df = pd.DataFrame(
[["John", 20, 45], ["Peter", 21, 62], ["Scot", 25, 68]],
index=[0, 1, 2],
columns=["Name", "Age", "Marks"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(example_df))
example_df.columns = ["Name", "Age", "Roll_no"]
print "\nModified DataFrame"
print (pd.DataFrame(example_df))
Output:
Original DataFrame
Name Age Marks
0 John 20 45
1 Peter 21 62
2 Scot 25 68
Modified DataFrame
Name Age Roll_no
0 John 20 45
1 Peter 21 62
2 Scot 25 68
One drawback of this approach is that you have to list the entire column even if only one of the columns needs to be renamed. Specifying the entire column list becomes impractical when you have a large number of columns.
Rename Columns in Pandas DataFrame
Using DataFrame.rename()
Method
The alternative approach to the previous method is using DataFrame.rename()
method. This method is quite handy when we need not rename all columns.
We will need to specify the old column name as key and new names as values.
import pandas as pd
example_df = pd.DataFrame(
[["John", 20, 45, 78], ["Peter", 21, 62, 68], ["Scot", 25, 68, 95]],
index=[0, 1, 2],
columns=["Name", "Age", "Marks", "Roll_no"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(example_df))
example_df.rename(columns={"Marks": "Roll_no", "Roll_no": "Marks"}, inplace=True)
print "\nModified DataFrame"
print (pd.DataFrame(example_df))
Output:
Original DataFrame
Name Age Marks Roll_no
0 John 20 45 78
1 Peter 21 62 68
2 Scot 25 68 95
Modified DataFrame
Name Age Roll_no Marks
0 John 20 45 78
1 Peter 21 62 68
2 Scot 25 68 95
The most significant advantage of this method is that you can specify as many columns as you want. It is quite useful when you need to rename specific columns and unlike previous methods no need to list the entire column list for the DataFrame
.
You might notice that in the DataFrame.rename()
function call, we have specified the inplace
parameter as True
. inplace
parameter is by default False
and determines whether to return a new pandas DataFrame
or not.
Specifying it as True
means function call doesn’t return a new pandas DataFrame
but changes the existing DataFrame
in place.
Rename Columns in Pandas DataFrame
Using DataFrame.set_axis()
Method
Another convenient method to rename columns of pandas DataFrame
. We have to specify the entire column list while using this method.
import pandas as pd
example_df = pd.DataFrame(
[["John", 20, 45, 78], ["Peter", 21, 62, 68], ["Scot", 25, 68, 95]],
index=[0, 1, 2],
columns=["Name", "Age", "Marks", "Roll_no"],
)
print "\nOriginal DataFrame"
print (pd.DataFrame(example_df))
example_df.set_axis(["Name", "Age", "Roll_no", "Marks"], axis="columns", inplace=True)
print "\nModified DataFrame"
print (pd.DataFrame(example_df))
Output:
Original DataFrame
Name Age Marks Roll_no
0 John 20 45 78
1 Peter 21 62 68
2 Scot 25 68 95
Modified DataFrame
Name Age Roll_no Marks
0 John 20 45 78
1 Peter 21 62 68
2 Scot 25 68 95