How to Convert Python Dictionary to Pandas DataFrame
-
Method to Convert
dictionary
to PandasDataFame
-
Method to Convert
keys
to Be thecolumns
and thevalues
to Be therow
Values in PandasDataFrame
-
pandas.DataFrame().from_dict()
Method to Convertdict
IntoDataFrame
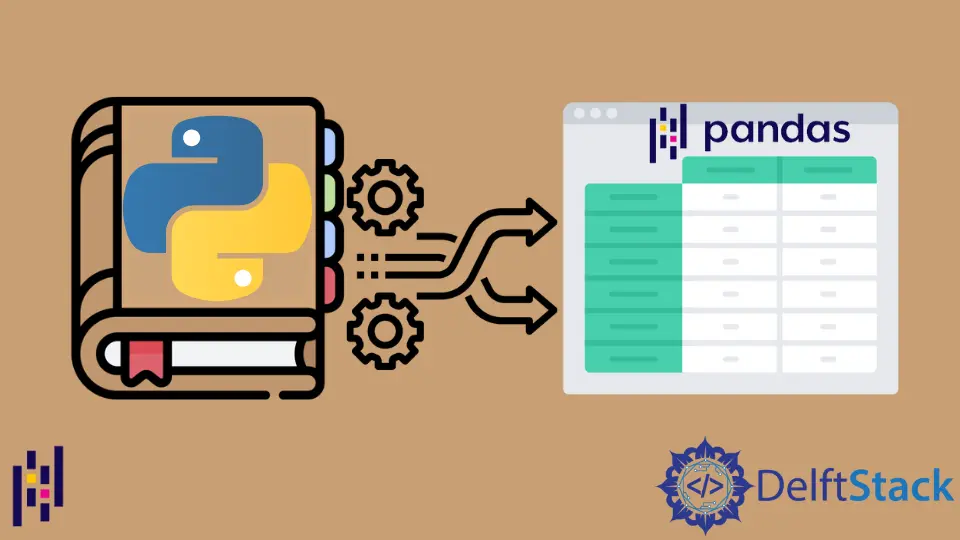
We will introduce the method to convert the Python dictionary
to Pandas datafarme
, and options like having keys
to be the columns
and the values
to be the row
values. We could also convert the nested dictionary
to DataFrame
.
We will also introduce another approach using pandas.DataFrame.from_dict
, and we will chain this with any rename
method to set both the index and columns’ names in one go.
Method to Convert dictionary
to Pandas DataFame
Pandas DataFrame
constructor pd.DataFrame()
converts the dictionary to DataFrame
if the items
of the dictionary is given as the constructor’s argument, but not the dictionary itself.
# python 3.x
import pandas as pd
fruit_dict = {3: "apple", 2: "banana", 6: "mango", 4: "apricot", 1: "kiwi", 8: "orange"}
print(pd.DataFrame(list(fruit_dict.items()), columns=["Quantity", "FruitName"]))
The keys
and values
of the dictionary are converted to two columns of the DataFrame
with the column names given in the options columns
.
Quantity FruitName
0 3 apple
1 2 banana
2 6 mango
3 4 apricot
4 1 kiwi
5 8 orange
Method to Convert keys
to Be the columns
and the values
to Be the row
Values in Pandas DataFrame
We can simply put brackets around the dictionary and remove the column name from the above code like this:
import pandas as pd
fruit_dict = {1: "apple", 2: "banana", 3: "mango", 4: "apricot", 5: "kiwi", 6: "orange"}
print(pd.DataFrame([fruit_dict]))
Output:
1 2 3 4 5 6
0 apple banana mango apricot kiwi orange
We will use pandas dictionary comprehension with concat
to combine all dictionaries
and then pass the list to give new column
names.
Consider the following code,
import pandas as pd
data = {"1": {"apple": 11, "banana": 18}, "2": {"apple": 16, "banana": 12}}
df = pd.concat({k: pd.Series(v) for k, v in data.items()}).reset_index()
df.columns = ["dict_index", "name", "quantity"]
print(df)
Output:
dict_index name quantity
0 1 apple 11
1 1 banana 18
2 2 apple 16
3 2 banana 12
pandas.DataFrame().from_dict()
Method to Convert dict
Into DataFrame
We will use from_dict
to convert dict
into DataFrame
, here we set orient='index'
for using dictionary keys as rows and applying rename()
method to change the column name.
Consider the following code,
import pandas as pd
print(
pd.DataFrame.from_dict(
{"apple": 3, "banana": 5, "mango": 7, "apricot": 1, "kiwi": 8, "orange": 3},
orient="index",
).rename(columns={0: "Qunatity"})
)
Output:
Quantity
apple 3
banana 5
mango 7
apricot 1
kiwi 8
orange 3