How to Change Order of Columns Pandas Dataframe
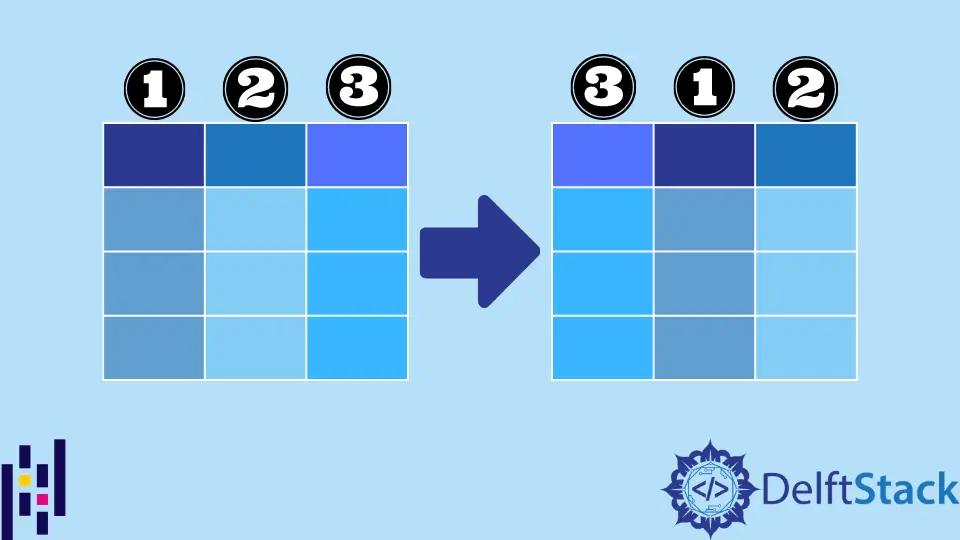
Pandas dataframes are two-dimensional data structures that store information in rows and columns.
Use the reindex()
Function to Change Order of Columns Python Pandas Dataframe
The reindex()
function in pandas can be used to reorder or rearrange the columns of a dataframe. We will create a new list of your columns in the desired order, then use data= data[cols]
to rearrange the columns in this new order.
First, we need to import python libraries numpy and pandas. Then declare a variable data in which we use an [np.random.rand(10, 5)
function](/api/numpy/python-numpy-random.rand-function/ creates a dataframe of 5 columns and 10 rows.
This function creates random values to make the dataframe have the following DataFrame:
import pandas as pd
import numpy as np
data = pd.DataFrame(np.random.rand(10, 5))
data
Output:
0 1 2 3 4
0 0.277764 0.778528 0.443376 0.838117 0.256161
1 0.986206 0.647985 0.061442 0.703383 0.415676
2 0.963891 0.477693 0.558834 0.616453 0.842086
3 0.746559 0.180196 0.038300 0.391343 0.877589
4 0.554592 0.958017 0.286683 0.526546 0.185318
5 0.370137 0.751773 0.594891 0.570358 0.779640
6 0.795137 0.271263 0.742291 0.615652 0.728313
7 0.912602 0.254319 0.455149 0.241939 0.250034
8 0.125905 0.300788 0.767852 0.265875 0.599287
9 0.400207 0.516693 0.345934 0.691878 0.088651
By assignment, add another column(s): use the following code, so the column calculates the mean
value of the dataframe created above.
data["mean"] = data.mean(1)
data
Output:
The above output shows the 6th column mean. How can we move the column mean to the front, i.e., make it the first column, while keeping the other columns in their original order?
One straightforward solution is to reassign the dataframe with a list of the columns, which can then be reconstructed as needed. The columns.tolist()
function lists the column’s name in a list.
columns_name = data.columns.tolist()
columns_names
Output:
[0, 1, 2, 3, 4, 'mean']
Rearrange the cols however you want. This is how we got the last element to go to the top:
columns = columns_name[-1:] + columns_name[:-1]
columns
Output:
['mean', 0, 1, 2, 3, 4]
The above output shows that the mean column moves to first. Similarly, we change the index values to first change the columns no 4 positions.
columns = columns_name[-2:] + columns_name[:-3]
columns
Output:
[4, 'mean', 0, 1, 2]
As you can see, the 4th column moves to the first and first column, which means move to the 2nd position. This is how we change the order of columns.
Now we use the reindex()
function to reorder the columns of the python dataframe. You can also use a list of column names and pass that list to the reindex()
method, as shown below.
Use reindex()
function to reorder. Columns are accepted as a list by the reindex()
method.
Single large brackets with column names are used to change column order by name.
column_names = [0, 2, 3, 1, 4, "mean"]
data = data.reindex(columns=column_names)
data
Output:
0 2 3 1 4 mean
0 0.277764 0.443376 0.838117 0.778528 0.256161 0.518789
1 0.986206 0.061442 0.703383 0.647985 0.415676 0.562938
2 0.963891 0.558834 0.616453 0.477693 0.842086 0.691791
3 0.746559 0.038300 0.391343 0.180196 0.877589 0.446797
4 0.554592 0.286683 0.526546 0.958017 0.185318 0.502231
5 0.370137 0.594891 0.570358 0.751773 0.779640 0.613360
6 0.795137 0.742291 0.615652 0.271263 0.728313 0.630531
7 0.912602 0.455149 0.241939 0.254319 0.250034 0.422809
8 0.125905 0.767852 0.265875 0.300788 0.599287 0.411942
9 0.400207 0.345934 0.691878 0.516693 0.088651 0.408673
The above output shows you can rearrange the columns by indexing the dataframe with the column names and creating a new dataframe.
Then use reindex()
by index value by index name. Use double brackets with index names.
data = data[[1, 0, 2, 3, 4, "mean"]]
data
Output:
1 0 2 3 4 mean
0 0.778528 0.277764 0.443376 0.838117 0.256161 0.518789
1 0.647985 0.986206 0.061442 0.703383 0.415676 0.562938
2 0.477693 0.963891 0.558834 0.616453 0.842086 0.691791
3 0.180196 0.746559 0.038300 0.391343 0.877589 0.446797
4 0.958017 0.554592 0.286683 0.526546 0.185318 0.502231
5 0.751773 0.370137 0.594891 0.570358 0.779640 0.613360
6 0.271263 0.795137 0.742291 0.615652 0.728313 0.630531
7 0.254319 0.912602 0.455149 0.241939 0.250034 0.422809
8 0.300788 0.125905 0.767852 0.265875 0.599287 0.411942
9 0.516693 0.400207 0.345934 0.691878 0.088651 0.408673
Conclusion
We already know how to reorder dataframe columns using the reindex()
method and dataframe indexing and sort the columns alphabetically in ascending or descending order.
Also, we have discovered how to move the column to the first, last, or specific position. These operations can be used in the pandas dataframe to perform various data manipulation operations.