How to Apply Lambda Function to Pandas DataFrame
- Lambda Function
-
Applying Lambda Function on a Single Column Using
DataFrame.assign()
Method -
Applying Lambda Function on Multiple Columns Using
DataFrame.assign()
Method -
Applying Lambda Function on a Single Row Using
DataFrame.apply()
Method - Filtering Data by Applying Lambda Function
-
Use the
map()
Function by Applying Lambda Function -
Use
if-else
Statement by Applying Lambda Function - Conclusion
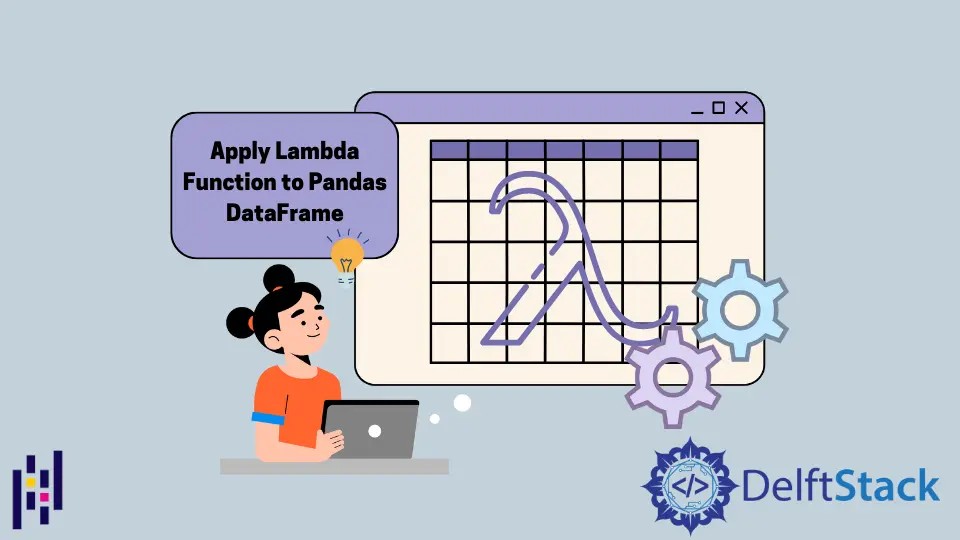
The lambda
function solves various data science problems in Pandas python. We can apply the lambda function on both rows and columns in the pandas DataFrame
.
We will explore in this article how to apply the lambda functions to pandas DataFrame
.
There are several applications of lambda function on pandas DataFrame
such as filter()
, map()
, and conditional statements
that we will explain with the help of some examples in this article.
Lambda Function
Lambda function contains a single expression.
The Lambda
function is a small function that can also use as an anonymous function means it doesn’t require any name. The lambda
function is useful to solve small problems with less code.
The following syntax is used to apply a lambda function on pandas DataFrame
:
dataframe.apply(lambda x: x + 2)
Applying Lambda Function on a Single Column Using DataFrame.assign()
Method
The dataframe.assign()
method applies the Lambda function on a single column. Let’s take an example.
In the following example, we have applied a lambda function on the column Students Marks
. After applying the Lambda function, the student percentages are calculated and stored in a new Percentage
column.
See the following implementation to apply a lambda function on a single column in Pandas DataFrame
.
Example Code:
import pandas as pd
# initialization of list
students_record = [
["Samreena", 900],
["Mehwish", 750],
["Asif", 895],
["Mirha", 800],
["Affan", 850],
["Raees", 950],
]
# pandas dataframe creation
dataframe = pd.DataFrame(students_record, columns=["Student Names", "Student Marks"])
# using Lambda function
dataframe1 = dataframe.assign(Percentage=lambda x: (x["Student Marks"] / 1000 * 100))
# display dataframe
print(dataframe1)
Output:
Student Names Student Marks Percentage
0 Samreena 900 90.0
1 Mehwish 750 75.0
2 Asif 895 89.5
3 Mirha 800 80.0
4 Affan 850 85.0
5 Raees 950 95.0
Applying Lambda Function on Multiple Columns Using DataFrame.assign()
Method
We can also apply the Lambda function on multiple columns using the dataframe.assign()
method in Pandas DataFrame
.
For example, we have four columns Student Names
, Computer
, Math
, and Physics
. We applied a Lambda function on multiple subjects columns such as Computer
, Math
, and Physics
to calculate the obtained marks stored in the Marks_Obtained
column.
Implement the following example.
Example Code:
import pandas as pd
# nested list initialization
values_list = [
["Samreena", 85, 75, 100],
["Mehwish", 90, 75, 90],
["Asif", 95, 82, 80],
["Mirha", 75, 88, 68],
["Affan", 80, 63, 70],
["Raees", 91, 64, 90],
]
# pandas dataframe creation
df = pd.DataFrame(values_list, columns=["Student Names", "Computer", "Math", "Physics"])
# applying Lambda function
dataframe = df.assign(
Marks_Obtained=lambda x: (x["Computer"] + x["Math"] + x["Physics"])
)
# display dataframe
print(dataframe)
Output:
Student Names Computer Math Physics Marks_Obtained
0 Samreena 85 75 100 260
1 Mehwish 90 75 90 255
2 Asif 95 82 80 257
3 Mirha 75 88 68 231
4 Affan 80 63 70 213
5 Raees 91 64 90 245
Applying Lambda Function on a Single Row Using DataFrame.apply()
Method
The dataframe.apply()
method applies the Lambda function on a single row.
For example, we applied the lambda function a single row axis=1
. Using the lambda function, we incremented each person’s Monthly Income
by 1000.
Example Code:
import pandas as pd
df = pd.DataFrame(
{
"ID": [1, 2, 3, 4, 5],
"Names": ["Samreena", "Asif", "Mirha", "Affan", "Mahwish"],
"Age": [20, 25, 15, 10, 30],
"Monthly Income": [4000, 6000, 5000, 2000, 8000],
}
)
df["Monthly Income"] = df.apply(lambda x: x["Monthly Income"] + 1000, axis=1)
print(df)
Output:
ID Names Age Monthly Income
0 1 Samreena 20 5000
1 2 Asif 25 7000
2 3 Mirha 15 6000
3 4 Affan 10 3000
4 5 Mahwish 30 9000
Filtering Data by Applying Lambda Function
We can also filter the desired data by applying the Lambda function.
The filter()
function takes pandas series and a lambda function. The Lambda function applies to the pandas series that returns the specific results after filtering the given series.
In the following example, we have applied the lambda function on the Age
column and filtered the age of people under 25 years.
Example Code:
import pandas as pd
df = pd.DataFrame(
{
"ID": [1, 2, 3, 4, 5],
"Names": ["Samreena", "Asif", "Mirha", "Affan", "Mahwish"],
"Age": [20, 25, 15, 10, 30],
"Monthly Income": [4000, 6000, 5000, 2000, 8000],
}
)
print(list(filter(lambda x: x < 25, df["Age"])))
Output:
[20, 15, 10]
Use the map()
Function by Applying Lambda Function
We can use the map()
and lambda functions.
The lambda function applies on series to map the series based on the input correspondence. This function is useful to substitute or replace a series with other values.
When we use the map()
function, the input size will equal the output size. To understand the concept of the map()
function, see the following source code implementation.
Example Code:
import pandas as pd
df = pd.DataFrame(
{
"ID": [1, 2, 3, 4, 5],
"Names": ["Samreena", "Asif", "Mirha", "Affan", "Mahwish"],
"Age": [20, 25, 15, 10, 30],
"Monthly Income": [4000, 6000, 5000, 2000, 8000],
}
)
df["Monthly Income"] = list(map(lambda x: int(x + x * 0.5), df["Monthly Income"]))
print(df)
Output:
ID Names Age Monthly Income
0 1 Samreena 20 6000
1 2 Asif 25 9000
2 3 Mirha 15 7500
3 4 Affan 10 3000
4 5 Mahwish 30 12000
Use if-else
Statement by Applying Lambda Function
We can also apply the conditional statements on pandas dataframes
using the lambda function.
We used the conditional statement inside the lambda function in the following example. We applied the condition on the Monthly Income
column.
If the monthly income is greater and equal to 5000, add Stable
inside the Category
column; otherwise, add UnStable
.
Example Code:
import pandas as pd
df = pd.DataFrame(
{
"ID": [1, 2, 3, 4, 5],
"Names": ["Samreena", "Asif", "Mirha", "Affan", "Mahwish"],
"Age": [20, 25, 15, 10, 30],
"Monthly Income": [4000, 6000, 5000, 2000, 8000],
}
)
df["Category"] = df["Monthly Income"].apply(
lambda x: "Stable" if x >= 5000 else "UnStable"
)
print(df)
Output:
ID Names Age Monthly Income Category
0 1 Samreena 20 4000 UnStable
1 2 Asif 25 6000 Stable
2 3 Mirha 15 5000 Stable
3 4 Affan 10 2000 UnStable
4 5 Mahwish 30 8000 Stable
Conclusion
We implemented various methods for applying the Lambda function on Pandas DataFrame
. We have seen how to apply the lambda function on rows and columns using the dataframe.assign()
and dataframe.apply()
methods.
We demonstrated the different applications of the lambda function on pandas DataFrame
series, such as the filter()
function, map()
function, conditional statements, and more.