How to Use the Flask redirect() Function With Parameters
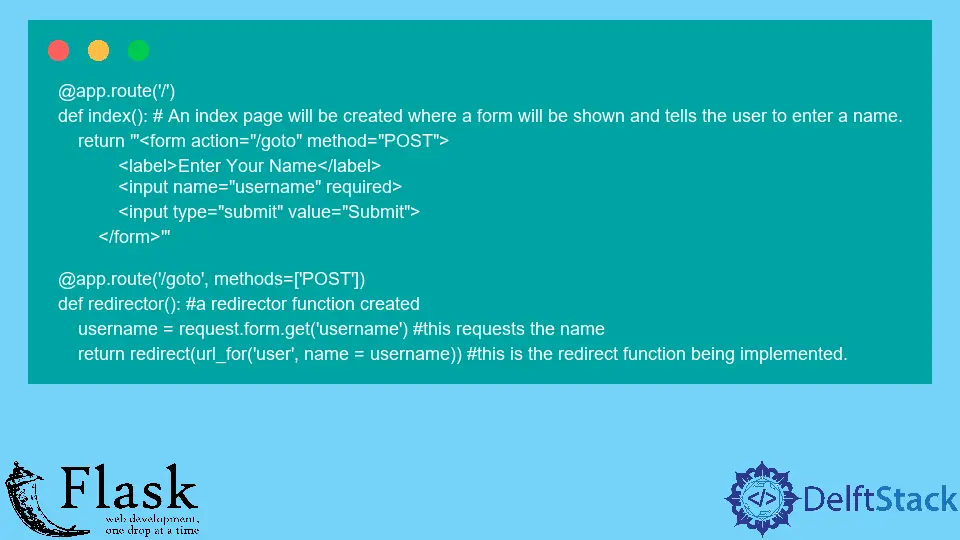
Here, we will discuss Flask’s redirect()
function. What is its parameter, how is it used, and what is needed to execute it? So, let us start learning about it.
Use the Flask redirect()
Function With Parameters
redirect()
is a Flask function or utility used explicitly for the users to redirect to a specific URL and then assign a status code, known as an Error. Error and redirect()
go together, but we won’t look into them in this guide.
The redirection occurs to the target location, and a response object is returned with the status code when this function is called.
An HTTP code is generated when a website receives a request and responds with a three-digit code after it processes it.
Let us look into an example to further understand the working of the redirect()
function. Here, we are showing a code snippet for an easy explanation; the full code will be provided at the end of this guide.
@app.route("/")
def index(): # An index page will be created where a form will be shown and tells the user to enter a name.
return """<form action="/goto" method="POST">
<label>Enter Your Name</label>
<input name="username" required>
<input type="submit" value="Submit">
</form>"""
@app.route("/goto", methods=["POST"])
def redirector(): # a redirector function created
username = request.form.get("username") # this requests the name
return redirect(
url_for("user", name=username)
) # this is the redirect function being implemented.
Let us look at the code’s functions, index
and redirector
.
The index()
function is created for one purpose, which is to create a form. Using the form, we can enter any name which will be used as the URL for the new website.
The redirector()
function includes two parts: the username
and the redirect
function. The username
here is used to request a name for the form; the one entered here is linked to the index()
function as the form is created there.
The second part, redirect()
, will redirect to the website mentioned in its parameters. In this case, the website is created using the url_for()
function.
The function url_for()
is used here to create a URL for us to redirect. We are not using any officially created URLs in this example; instead, we are using our own URLs.
Several keyword arguments represent variables in the URL rule, with the first argument being the function’s name. As query parameters, URLs with unknown variables are appended.
Now, let us understand this example by looking at the output. In this example, we have created an index
page where the system asks the user to enter a name for it to redirect it to that page.
Here, we will enter any name, and it will redirect it to a page created by that name. For example, we type in the name Aria
.
As you can see, we have been successfully redirected to a page named Aria
.
Now, let us look at another example that will redirect us to google.com
when asked to.
@app.route("/goto1") # \goto1 will be used as a keyword to redirect.
def redirector_1():
return redirect("https://www.google.com") # will redirect to google
We must type in the URL \goto1
for this example. That is all we would have to do to be redirected to google.com
.
Here is how to type in the URL.
The http address provided by the system/goto1
http://127.0.0.1:5000/goto1 #accoridng to example
The full code below includes both ways the redirect
function is used.
from flask import Flask, request, redirect, url_for
app = Flask(__name__)
@app.route("/")
def index():
return """<form action="/goto" method="POST">
<label>Enter Your Name</label>
<input name="username" required>
<input type="submit" value="Submit">
</form>"""
@app.route("/goto", methods=["POST"])
def redirector():
username = request.form.get("username")
return redirect(url_for("user", name=username))
@app.route("/goto1")
def redirector_1():
return redirect("https://www.google.com")
@app.route("/user/<name>")
def user(name):
return f"Hello {name}"
if __name__ == "__main__":
app.run(debug=True)
We must import the above libraries, Flask
, request
, redirect
, and url_for
. Without importing these, we won’t be able to use any of the functions that we have used in this code.
According to this code, the request
will help us request a name to create the new website, and the redirect
will allow us to redirect to the specific website we mentioned in the parameters.
Last but not the least, the url_for
will allow us to use the name created with the help of the form from the index
function to create a new website.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn