How to Redirect in Python Flask
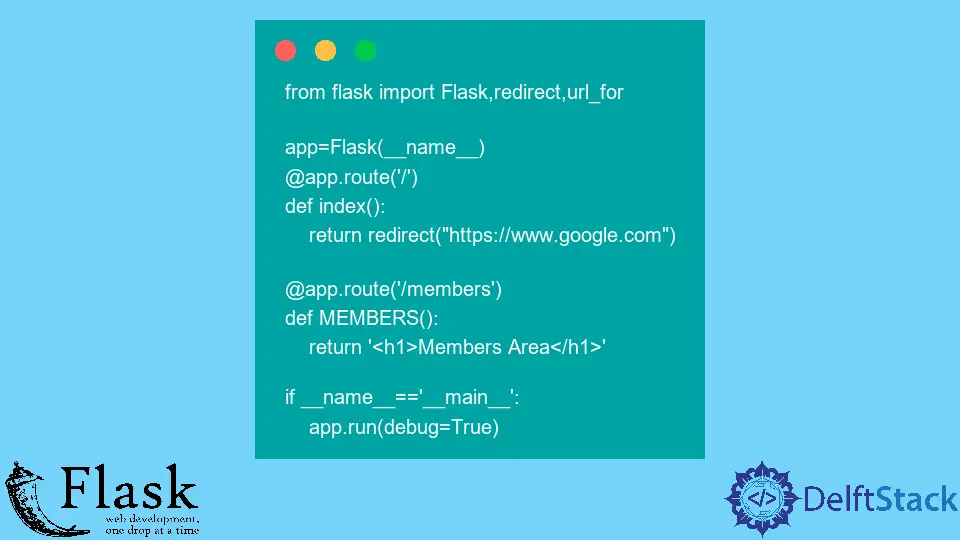
We will learn, with this explanation, how to use the redirect()
function to redirect the user and see how we can use it for multiple purposes in Flask.
Redirect the User With the Help of the redirect()
Function in Flask
In Flask, redirecting is fairly simple. It simply redirects a user or someone on your site by using a request or an API.
There are different ways to redirect the user. For example, if they land on your home page and they log in to your site, then you can redirect them to either the membership area, or you can redirect them to the password recovery page, wherever you like.
In general, a redirect is a way to send people to the next page from the server-side. So they don’t have to initiate the request on that page.
Before we start writing the code, we’ll show you the redirect()
function syntax here.
Syntax:
flask.redirect(location, code=302, Response=None)
We will only use the first two parameters, location
and code
. The location
is a URL, and the code
is the HTTP status code that gets sent with the request or the redirect()
response.
Let us demonstrate that with some code. Here, we will set up a Flask app quickly and start with importing Flask.
We will set up a couple of routes: one is creating an index()
function with header
tags, and another is a MEMBERS()
function which also uses a header
tag with a specific endpoint.
The first example is redirecting between pages in our app. We created two views or two pages, and we want someone who navigates to the index to be automatically redirected to the members’ page.
So, we will use the redirect()
function along with the url_for()
function. The redirect()
function is going to take a location for the first parameter, and this location
parameter needs to be the url_for()
.
We only need to type the name of the function inside the url_for()
which we want, the MEMBERS()
function generates the URL for members, and then this index()
function will redirect the user to the members’ page.
from flask import Flask, redirect, url_for
app = Flask(__name__)
@app.route("/")
def index():
return redirect(url_for("MEMBERS"))
@app.route("/members")
def MEMBERS():
return "<h1>Members Area</h1>"
if __name__ == "__main__":
app.run(debug=True)
After saving it, if we run this app using the index page and when we navigate there, it redirects us to the members’ page.
Open up Developer Tools, and let’s see what the network is saying when we run this page. We requested the index here, which gives us a status of 302
.
302
is typically used when we redirect someone; it sends us to the members’ page, but we didn’t request the server to do this.
Output:
We can also change the second parameter into 404
as the redirect, which would be strange, but we can use it in any condition. We will go to the index, which tells us that You should be automatically redirected to target URL. If not, click the link
.
It is because typically, a 404
is used for errors and not for redirects, but if we click on that link generated by Flask, it sends us to the members’ area. You do not need to modify the status code unless you have a specific reason; keep that in mind.
from flask import Flask, redirect, url_for
app = Flask(__name__)
@app.route("/")
def index():
return redirect(url_for("MEMBERS"), 404)
@app.route("/members")
def MEMBERS():
return "<h1>Members Area</h1>"
if __name__ == "__main__":
app.run(debug=True)
Output:
Suppose you want to redirect to any URL instead of the members’ area; let’s say you want to redirect to something that is off your site for whatever reason. In our case, we want them to be automatically redirected to google.com
.
from flask import Flask, redirect, url_for
app = Flask(__name__)
@app.route("/")
def index():
return redirect("https://www.google.com")
@app.route("/members")
def MEMBERS():
return "<h1>Members Area</h1>"
if __name__ == "__main__":
app.run(debug=True)
Output:
Depending on the result of that process, you will redirect them somewhere. For example, you get the post data for their login information if you figure out the username and password are valid.
If the password is correct, you would redirect them to a member’s area or anywhere you want to show the user. Or you can redirect them to a password recovery page if the password is wrong; that is completely up to you but know that the redirect typically takes place after some processing in the view.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn