How to Use the Where-Object Command in PowerShell
-
the
Where-Object
Cmdlet in PowerShell - Creating Filter Conditions With Script Blocks in PowerShell
-
Use
Where-Object
to Filter Objects in PowerShell - Creating Filter Conditions With Parameters in PowerShell
- Using Multiple Conditional Statements
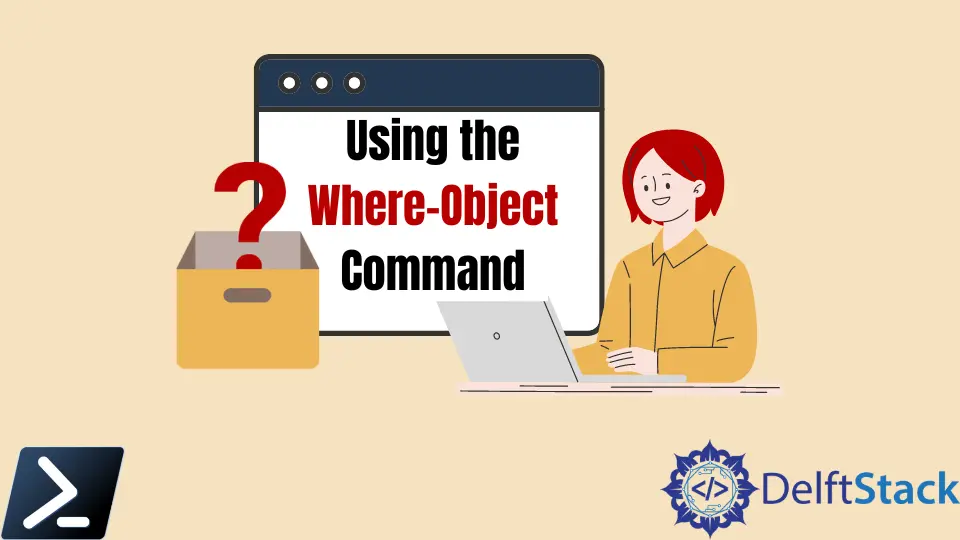
When you work with Windows PowerShell properties in a collection of objects, sometimes you will need a way to filter all the specific things you only need. Therefore, knowing how to use the Windows PowerShell Where-Object
cmdlet is a vital technique to have in your Windows PowerShell skillset.
The Where-Object
cmdlet is a valuable command to filter objects. In this article, we will learn several ways to construct a Where-Object
command, its syntax, and the use of multiple conditional statements.
the Where-Object
Cmdlet in PowerShell
The Windows PowerShell Where-Object
cmdlet’s only goal is to filter the output of a command and return specific information that we want to print out.
In summary, the Where-Object
cmdlet is a filter. It allows us to construct a condition that returns either True
or False
. Depending on the result of the situation, the cmdlet then either returns the output or not.
You can craft that condition in two ways: script blocks and parameters.
Creating Filter Conditions With Script Blocks in PowerShell
Script blocks are a vital component in Windows PowerShell. They’re used in hundreds of places throughout the scripting language. A script block is an anonymous function that categorizes code and executes it in various places.
Example Script Block:
{$_.StartType -EQ 'Automatic'}
Using the Windows PowerShell pipeline, you could then pipe those objects to the Where-Object
cmdlet and use the FilterScript
parameter. Since the FilterScript
parameter accepts a script block, we can create a condition to check whether or not the property of each object is equal to the specific value, like the example below.
Example Code:
Get-Service | Where-Object -FilterScript { $_.StartType -eq 'Automatic' }
Where-Object
cmdlet and don’t include the FilterScript
parameter name. Instead, they provide the script block alone like Where-Object {$_.StartType -eq 'Automatic'}
for a faster and cleaner way of scripting.While this type of syntax works for this particular scenario, the concept of a script block with the curly braces ({}
) makes the code less readable and more difficult for less experienced Windows PowerShell users. This readability issue caused the Windows PowerShell team to introduce parameters.
Use Where-Object
to Filter Objects in PowerShell
The Get-ChildItem
gets the list of all files and folders at the specified location. Let’s consider you only want to display the .csv
files in the directory.
The following example shows how to use the Where-Object
cmdlet to filter and select only .csv
files.
Get-ChildItem | Where-Object { $_.extension -eq ".csv" }
In the above script, the output of Get-ChildItem
is piped to the Where-Object
cmdlet. The Where-Object
filters the output where the file extension is .csv
.
Output:
Directory: C:\Users\rhntm
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 3/11/2022 7:57 AM 122 books.csv
-a---- 3/8/2022 2:07 PM 780199 house_data.csv
-a---- 3/8/2022 10:38 PM 421 play_tennis.csv
You can also use Where
as the alias for the Where-Object
cmdlet.
Below is another example that filters the list of processes with the ProcessName
property value. It displays only processes that have spotify
as the ProcessName
.
Get-Process | where { $_.ProcessName -in "spotify" }
Output:
Handles NPM(K) PM(K) WS(K) CPU(s) Id SI ProcessName
------- ------ ----- ----- ------ -- -- -----------
550 31 55036 71256 2.81 1820 3 Spotify
294 21 12328 18144 0.06 13536 3 Spotify
476 64 96012 141208 20.61 13980 3 Spotify
You can use the -notin
operator to select values, not in the collection of objects.
For example, the following example prints all the processes that do not have spotify
as the ProcessName
.
Get-Process | where { $_.ProcessName -notin "spotify" }
In this way, you can use the Where-Object
cmdlet to filter the objects from the collection of objects based on their property values.
Creating Filter Conditions With Parameters in PowerShell
Introduced in Windows PowerShell 3.0, parameters have more of a natural flow to how they are written. Using the same previous example, let’s use parameters to filter a specific output.
Example Code:
Get-Service | Where-Object -Property StartType -EQ 'Automatic'
Notice above that instead of using a script block, the command specifies the object property as a parameter value to the Property parameter. In addition, the -eq
operator is now a parameter, allowing you to pass the Automatic
value to it.
Using parameters in this manner now eliminates the need for a script block. However, though Microsoft has introduced parameters as a cleaner way of scripting, we have an excellent reason to use script blocks that we will discuss in the next section of the article.
Using Multiple Conditional Statements
You can combine two or more conditions utilizing an operator (like -and
or -or
) to evaluate them using a script block. However, we need to use a particular Windows PowerShell component called subexpressions to evaluate each condition successfully.
In Windows PowerShell, we can use subexpressions to run an expression within a filtering syntax like Where-Object
. We do this by enclosing whichever conditional statement we run with parentheses (()
).
Example Code:
Get-Process | Where-Object { ($_.CPU -gt 2.0) -and ($_.CPU -lt 10) }
Example Script Block:
Handles NPM(K) PM(K) WS(K) CPU(s) Id SI ProcessName
------ - ------ ---- - ---- - ------ -- -- ---------- -
334 21 18972 26384 5.23 3808 0 AnyDesk
635 34 13304 51264 9.56 4140 5 notepad
726 36 12820 51196 4.69 12088 5 notepad
802 46 18356 65088 7.98 10784 5 OneDrive
340 18 6472 26436 3.44 1252 5 RuntimeBroker
698 34 14672 44484 3.63 3284 5 RuntimeBroker
323 19 5732 23432 4.00 11200 5 RuntimeBroker
560 18 6440 27752 4.63 8644 5 sihost
632 31 21524 69972 2.81 6392 5 StartMenuExperienceHost
390 18 9756 30832 3.94 3084 5 svchost
523 24 8768 36312 2.17 6300 5 svchost
524 17 6416 21036 4.42 10932 5 SynTPEnh
525 22 15336 38904 3.41 2192 5 TextInputHost
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn