How to Combine Multiple Conditions in if Statement
-
the
if
Statement in PowerShell -
Use Logical Operators to Combine Multiple Conditions in the
if
Statement in PowerShell -
Use Multiple Operators to Combine Multiple Conditions in the
if
Statement in PowerShell -
Nesting
if
Statements to Combine Multiple Conditions in PowerShell - Conclusion
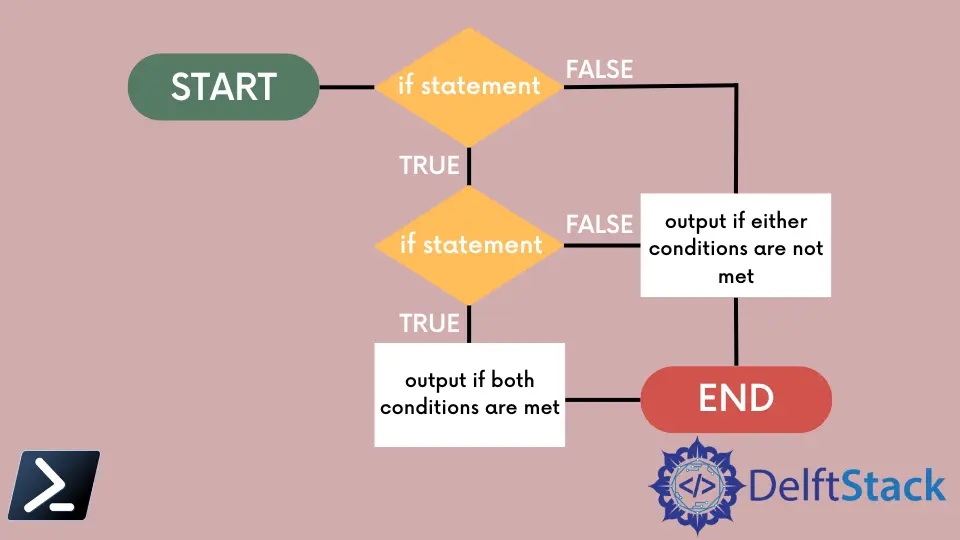
In PowerShell, the if
statement is a powerful tool for decision-making. However, many tasks require you to combine multiple conditions for more precise control.
We will look at in this article the various methods on how to combine multiple conditions using logical operators in PowerShell’s if
statement.
the if
Statement in PowerShell
Here is a simple example of an if
statement. If the condition inside the parentheses evaluates to true, it will execute the command Write-Host "2 is equal to 2."
.
Code:
if (2 -eq 2) {
Write-Host "2 is equal to 2."
}
If the specified condition in parentheses ()
evaluates to $true
, then it runs the command in the braces {}
.
Output:
2 is equal to 2.
If the condition is $false
, it will skip that code block. The above example uses one condition, but you can also evaluate multiple conditions in the if
statement.
Use Logical Operators to Combine Multiple Conditions in the if
Statement in PowerShell
The logical operators connect statements and expressions in PowerShell. You can use a single expression to test for multiple conditions by using them.
The supported logical operators in the PowerShell are -and
, -or
, -not
, -xor
, and !
. We will only discuss in this article how to use the -and
,-or
,-not
, and -xor
operators to combine multiple conditions in the if
statement in PowerShell.
Use the -and
Operator to Combine Multiple Conditions in the if
Statement in PowerShell
The following example uses the -and
operator to connect two statements combined in the if
statement. If the first condition 5 is less than 10
is true and the second condition 7 is greater than 5
is true, the Write-Host
command will be executed.
Code:
if ((5 -lt 10) -and (7 -gt 5)) {
Write-Host "The above conditions are true."
}
Output:
The above conditions are true.
Since both conditions are true in this case, the code block is executed, and the message "The above conditions are true."
is printed in the output.
Use the -or
Operator to Combine Multiple Conditions in the if
Statement in PowerShell
In this code, we use the -lt
(less than) and -gt
(greater than) operators to check if 5 is less than 10 or 7 is greater than 5. Then, we use the -or
operator to check if either (5 -lt 10)
or (7 -gt 5)
is true.
Code:
if ((5 -lt 10) -or (7 -gt 5)) {
Write-Host "At least one of the above conditions is true."
}
else {
Write-Host "Neither of the above conditions is true."
}
Output:
At least one of the above conditions is true.
Since both conditions are true in the original code, it prints that "At least one of the conditions is true."
.
Use the -not
Operator to Combine Multiple Conditions in the if
Statement in PowerShell
In the following code, we have a variable $number
representing a numeric value. We use the -lt
(less than) and -gt
(greater than) operators to check if the number is less than 5 or greater than 10.
The -or
operator is used to combine the two conditions on its left and right sides. It returns true if at least one of the conditions is true. Since both conditions are false, the -or
expression evaluates to false.
The -not
operator is applied to the result of the -or
expression. It negates the result since the -or
expression is false; applying -not
makes it true.
Code:
$number = 7
if (-not ($number -lt 5 -or $number -gt 10)) {
Write-Host "The number is between 5 and 10 (inclusive)."
}
else {
Write-Host "The number is outside the range of 5 to 10."
}
Output:
The number is between 5 and 10 (inclusive).
Because the -not
operator makes the combined condition true, the code inside the if
block is executed. The output indicates that the value of $number = 7
falls within the specified range of 5 to 10 (inclusive).
Use the -xor
Operator to Combine Multiple Conditions in the if
Statement in PowerShell
The following code checks two conditions and uses the -xor
operator to determine their combined truth value. Inside the if
statement, there are two conditions: The first condition is if 5 is less than 10, and the second condition is if 7 is greater than 5.
The -xor
operator is an exclusive OR
operator, which means it returns true if exactly one of its operands is true and false if both operands are true or both are false.
In this case, both conditions are true, so the -xor
expression evaluates to false, and the code inside the else
block is executed.
Code:
if ((5 -lt 10) -xor (7 -gt 5)) {
Write-Host "Exactly one of the above conditions is true."
}
else {
Write-Host "Neither or both of the above conditions are true."
}
Output:
Neither or both of the above conditions are true.
The output prints "Neither or both of the above conditions are true."
since it indicates that neither of the conditions is exclusively true; both conditions are either true or false together.
Use Multiple Operators to Combine Multiple Conditions in the if
Statement in PowerShell
Similarly, you can combine multiple conditions using logical operators in PowerShell’s if
statement. In this example, we execute different commands based on whether the combined conditions are true
or false
.
The code below combines multiple conditions using logical operators -and
, and -or
. If any of these conditions is true, it will execute the first command ( Write-Host "It is true."
) else, the second command (Write-Host "It is false."
) will execute.
Code:
if (((10 -lt 20) -and (10 -eq 10)) -or ((15 -gt 5) -and (12 -lt 6))) {
Write-Host "It is true."
}
else {
Write-Host "It is false."
}
Output:
It is true.
Here, the if
statement checks the combined conditions and executes the appropriate command block based on the evaluation of those conditions. Since the condition is true, it printed the message "It is true."
.
Remember to enclose each set of conditions within parentheses ()
and use the logical operators that suit your specific requirements.
Nesting if
Statements to Combine Multiple Conditions in PowerShell
Nesting if
statements can be valuable when you have complex conditions that require multiple layers of evaluation. For instance, when you need to check a condition within a specific context or perform different actions based on varying conditions, nesting if
statements allow for a more structured approach.
In this code, we declare a variable $number = 15
. Then, we create the outer if
statement that checks if the $number is greater than or equal to 10
.
If the outer condition is true, the nested if
statement checks if the $number is less than or equal to 20
.
Code:
$number = 15
if ($number -ge 10) {
if ($number -le 20) {
Write-Host "The number is between 10 and 20."
}
}
Output:
The number is between 10 and 20.
In this code, the outer if
statement checks if $number
is greater than or equal to 10
. If this condition is met, it proceeds to the nested if
statement, which checks if $number
is less than or equal to 20
.
Only when both conditions are true does it execute the Write-Host
command. In the output, both conditions are met, and printed out the message "The number is between 10 and 20."
.
Conclusion
In conclusion, combining multiple conditions in PowerShell’s if
statement is essential for making efficient scripts. With the logical operators -and
, -or
, -not
, and -xor
, you can create complex conditional logic to tackle a wide range of scripting challenges.
Whether it’s simple conditions or nested if
statements, PowerShell offers powerful tools to help you make informed decisions and execute the right actions in your scripts.