How to Return Several Items From a PowerShell Function
- Use Array to Return Several Items From a PowerShell Function
-
Use
[PSCustomObject]
to Return Several Items From a PowerShell Function - Use Hash Tables to Return Several Items From a PowerShell Function
- Use Custom Objects to Return Several Items From a PowerShell Function
- Conclusion
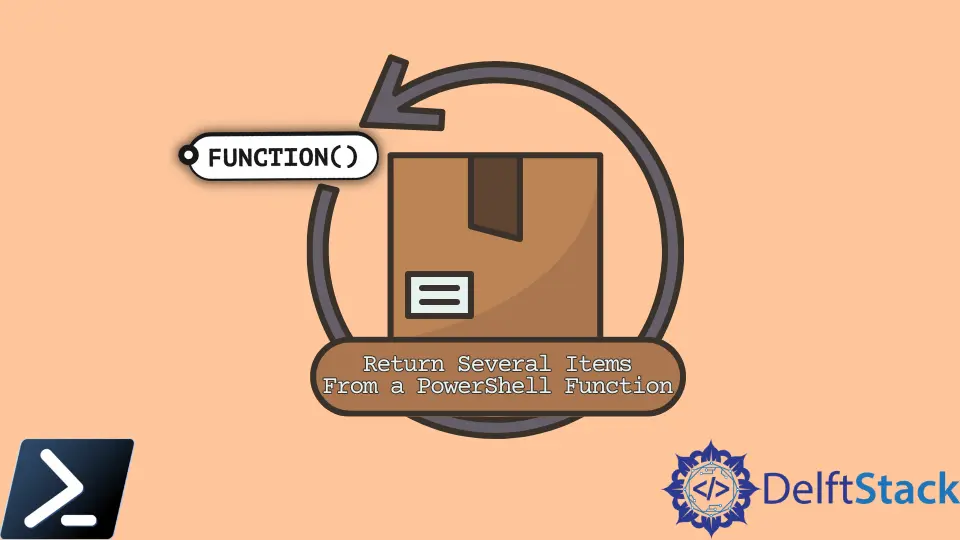
When working with PowerShell functions, there often arises a need to return multiple values efficiently. One effective approach is to utilize arrays, [PSCustomObject]
, hash tables or custom objects to encapsulate and present these values.
In this article, we’ll delve into how to use the methods mentioned to return multiple items from a PowerShell function. We’ll outline step-by-step procedures for each method and provide code examples to illustrate their implementation and showcase their outputs.
Use Array to Return Several Items From a PowerShell Function
To return multiple values from a PowerShell function using an array, follow these steps:
-
Declare an Array Variable: Start by declaring an array variable within the function to hold the multiple values you want to return.
-
Populate the Array: Populate the array with the desired values that you want to return. You can add elements to the array using indexing.
-
Return the Array: Return the populated array as the output of the function.
Let’s create a simple PowerShell function that returns multiple values using an array.
function Get-MultipleValues {
$values = @()
$values += "Value1"
$values += "Value2"
$values += "Value3"
return $values
}
$resultArray = Get-MultipleValues
Write-Output "Values returned from the function:"
foreach ($value in $resultArray) {
Write-Output $value
}
Output:
Values returned from the function:
Value1
Value2
Value3
In this example, we have a function named Get-MultipleValues
. Within the function, we declare an array variable $values
.
We then populate this array with three values: "Value1"
, "Value2"
, and "Value3"
. Finally, we return the populated array as the output of the function.
When calling the function, we store the result in a variable ($resultArray
) and display the values within the array using a loop.
Use [PSCustomObject]
to Return Several Items From a PowerShell Function
You can also use [PSCustomObject]
to create custom objects in PowerShell. It allows you to define properties and values for an object, making it a suitable choice for returning multiple values from a function.
To create a custom object using [PSCustomObject]
, you simply define the properties and their corresponding values like this:
$customObject = [PSCustomObject]@{
Property1 = 'Value1'
Property2 = 'Value2'
...
}
To return multiple values using [PSCustomObject]
in a PowerShell function, begin by defining the PowerShell function and creating an instance of [PSCustomObject]
. Define the properties and values you want to return within the custom object.
function Get-MultipleValues {
[CmdletBinding()]
param (
[string]$Value1,
[int]$Value2,
[string]$Value3
)
$resultObject = [PSCustomObject]@{
Name = $Value1
Age = $Value2
Address = $Value3
}
return $resultObject
}
Now, you can call the function and retrieve the custom object containing multiple values.
$result = Get-MultipleValues -Value1 'Rohan' -Value2 21 -Value3 'UK'
Write-Output "Values returned from the function:"
foreach ($value in $result) {
Write-Output $value
}
Output:
Values returned from the function:
Name Age Address
---- --- -------
Rohan 21 UK
As we can see, the PowerShell code above introduces a function named Get-MultipleValues
. This function, tagged with [CmdletBinding()]
, indicates its ability to utilize common cmdlet parameters.
Within the function, three parameters are defined: $Value1
(a string), $Value2
(an integer), and $Value3
(a string). The function constructs a custom object named $resultObject
using these parameters, assigning them to properties Name
, Age
, and Address
within the custom object.
The return $resultObject
statement ensures that this custom object is returned as the output of the Get-MultipleValues
function.
After that, the script calls the Get-MultipleValues
function, specifying values for each parameter: $Value1
is set to 'Rohan'
, $Value2
is set to 21
, and $Value3
is set to 'UK'
. The resulting custom object, incorporating the provided parameter values, is stored in the variable $result
.
Following this, the script employs Write-Output
to present a message on the console and initiates a loop, traversing through the properties of the custom object held in $result
. For each property, the script displays both the property name and its respective value on the console.
Use Hash Tables to Return Several Items From a PowerShell Function
A hash table in PowerShell is an unordered collection of key-value pairs. Each key must be unique, and the associated value can be of any data type.
It is also called a dictionary or associative array. Hash tables are particularly useful when you need to store and retrieve data based on specific keys, providing a structured way to organize information.
To return multiple values from a PowerShell function using hash tables, follow these steps:
-
Create a Hash Table: Instantiate a hash table within the function to store the key-value pairs representing the data you want to return.
You can create a hash table in PowerShell using
@{}
. The keys and values are placed in the{}
brackets.The syntax to create a hash table is as follows.
@{ <key> = <value>; [<key> = <value> ] ... }
-
Add Key-Value Pairs: Add the key-value pairs to the hash table, associating each value with a unique key.
-
Return the Hash Table: Return the populated hash table as the output of the function.
Let’s have an example that uses the hash table to return multiple values from a function named user
.
Code:
function user() {
$hash = @{ Name = 'Rohan'; Age = 21; Address = 'UK' }
return $hash
}
$a = user
Write-Host "$($a.Name) is $($a.Age) and lives in $($a.Address)."
Output:
Rohan is 21 and lives in UK.
As we can see, this code defines a function named user()
using the function
keyword. Within this function, a hash table named $hash
is created using the @{}
syntax, containing three key-value pairs: Name
with the value 'Rohan'
, Age
with the value 21
, and Address
with the value 'UK'
.
The return $hash
statement ensures that this hash table is the output of the user()
function.
Subsequently, the script calls the user()
function and assigns the resulting hash table to the variable $a
. This means that $a
now holds the hash table returned by the user()
function.
Finally, the Write-Host
cmdlet is used to display a message to the console. The message incorporates the values from the hash table stored in $a
, retrieved using $($a.Name)
, $($a.Age)
, and $($a.Address)
to access the Name
, Age
, and Address
values respectively.
Now, let’s create another PowerShell function that calculates the sum and product of two numbers and returns the results using a hash table.
function Calculate-SumAndProduct {
param(
[int]$num1,
[int]$num2
)
$resultHashTable = @{
Sum = $num1 + $num2
Product = $num1 * $num2
}
return $resultHashTable
}
$inputNum1 = 5
$inputNum2 = 7
$result = Calculate-SumAndProduct -num1 $inputNum1 -num2 $inputNum2
Write-Output "Sum: $($result['Sum'])"
Write-Output "Product: $($result['Product'])"
Output:
Sum: 12
Product: 35
This code begins by defining a function called Calculate-SumAndProduct
, which accepts two int
parameters, $num1
and $num2
. Inside the function, a hash table named $resultHashTable
is created using the @{}
syntax.
This hash table contains two key-value pairs: Sum
with a value equal to the sum of $num1
and $num2
, and Product
with a value equal to the product of $num1
and $num2
. The function then returns this hash table, making it the output of the Calculate-SumAndProduct
function.
Moving forward in the script, two input numbers are defined: $inputNum1
with a value of 5
and $inputNum2
with a value of 7
. The Calculate-SumAndProduct
function is called with these input numbers, and the resulting hash table is stored in a variable named $result
.
Finally, the script uses the Write-Output
cmdlet to display the sum and product values by accessing the corresponding values in the hash table stored in $result
.
Use Custom Objects to Return Several Items From a PowerShell Function
A custom object in PowerShell is an instance of the System.Management.Automation.PSCustomObject
class. It allows you to define properties and values associated with those properties, mimicking a real-world object.
Custom objects are flexible and enable the organization and retrieval of data in a structured manner.
To return multiple values from a PowerShell function using custom objects, follow these steps:
-
Define a Custom Object: Create a custom object within the function and define properties for the values you want to return.
-
Assign Property Values: Assign the values you want to return to the properties of the custom object.
-
Return the Custom Object: Return the populated custom object as the output of the function.
Let’s create a PowerShell function that calculates the sum and product of two numbers and returns the results using a custom object.
function Calculate-SumAndProduct {
param(
[int]$num1,
[int]$num2
)
$resultObject = New-Object PSObject
$resultObject | Add-Member -MemberType NoteProperty -Name "Sum" -Value ($num1 + $num2)
$resultObject | Add-Member -MemberType NoteProperty -Name "Product" -Value ($num1 * $num2)
return $resultObject
}
$inputNum1 = 5
$inputNum2 = 7
$result = Calculate-SumAndProduct -num1 $inputNum1 -num2 $inputNum2
Write-Output "Sum: $($result.Sum)"
Write-Output "Product: $($result.Product)"
Output:
Sum: 12
Product: 35
In this example, we defined a function named Calculate-SumAndProduct
that takes two input numbers ($num1
and $num2
). Inside the function, we created a custom object named $resultObject
and added properties (Sum
and Product
) to it, assigning the respective calculated values.
When calling the function with input numbers (5
and 7
), we store the result in a variable ($result
). We then displayed the sum and product by accessing the properties of the result custom object.
Conclusion
This article has explored various techniques to return multiple values from a function, including using arrays, [PSCustomObject]
, hash tables, and custom objects. Each method offers its advantages and can be selected based on the specific requirements of your script.
Arrays provide a straightforward way to return multiple values, especially when the data items share a similar type or structure. [PSCustomObject]
enables you to create custom objects with named properties, offering a structured approach for returning data.
Hash tables excel in scenarios where data retrieval is based on specific keys, providing an organized and efficient solution. Custom objects, built using the [System.Management.Automation.PSCustomObject]
class, offer flexibility and mimic real-world objects.