How to Use PowerShell Functions
- Difference Between Functions and Cmdlets in PowerShell
- Introduction to Functions in PowerShell
- Add Parameters to Functions in PowerShell
- Create a Simple Parameter on a Function in PowerShell
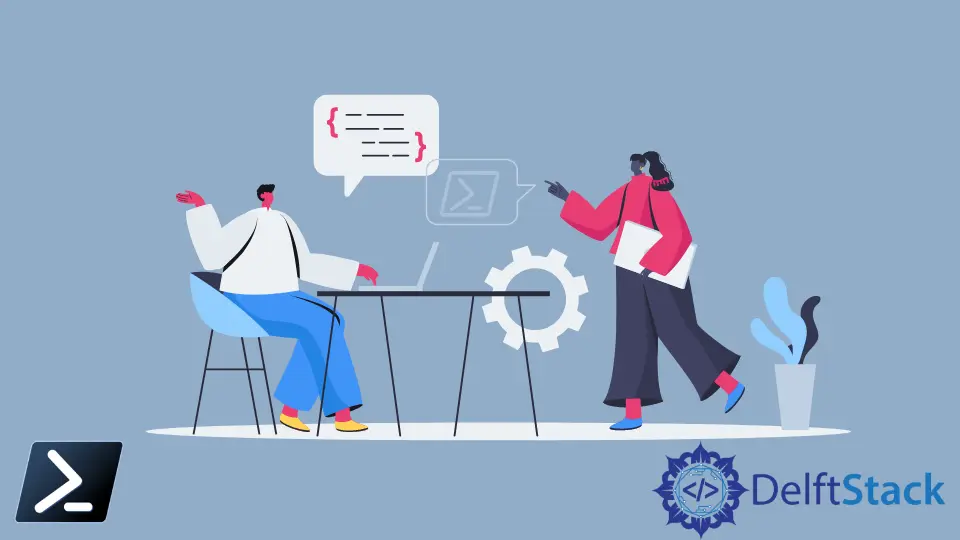
When we write a PowerShell script, we have many options. For example, we could write a thousand lines of code for tasks, all in a single block of code.
However, that would be complicated. So instead, we should write PowerShell functions.
PowerShell functions dramatically increase the usability and readability of our code, making it much easier to work with. In this article, we will learn to write functions, add and manage our functions’ parameters, and set up functions to accept pipeline input.
Difference Between Functions and Cmdlets in PowerShell
The concept of a PowerShell function might sound familiar because it sounds like the native PowerShell cmdlets. Commands like Write-Host
and Start-Service
, for example, are very similar to functions.
However, cmdlets are named pieces of code that solve a single problem and are not easily customizable. The difference between a cmdlet and a function is how these constructs are made.
We can see which commands are cmdlets and functions using the Get-Command
cmdlet and its -CommandType
parameter.
Get-Command -CommandType Function
The command above returns all the functions currently loaded into our PowerShell session or inside modules available to PowerShell.
Introduction to Functions in PowerShell
We use the function
keyword to define a function, followed by a descriptive, user-defined name and a set of curly braces. Inside the curly braces is a script block that we want PowerShell to execute.
Below we can see a primary function and execution of that function. The function, called Install-Software
, uses Write-Host
to display an output message in the console (primarily for simulating installation).
Once defined, we can use this function’s name to execute the code inside its script block.
Example Code:
function Install-Software {
Write-Host 'The software has been installed.'
}
Install-Software
Output:
The software has been installed.
Function-Naming Convention Best Practice with Verb-Noun Syntax in PowerShell
A function’s name is essential. We can name our functions whatever we want, but the name should always describe what the function does.
The function-naming convention best practice in PowerShell is the Verb-Noun syntax.
We should always start a function name with a verb followed by a dash and a noun. Use the Get-Verb
cmdlet to find the approved
verbs list.
Define an Advanced Function in PowerShell
The tutorial assumes we copied and pasted the previous code directly into the PowerShell console in this section. We can also define functions in a script.
We were working with a tiny function in the previous section, so defining it in the console wasn’t much of a problem. Most of the time, though, we will have much bigger functions.
So it’ll be easier to define those functions in a script or a module and then call that script or module to load the function into memory.
As we might imagine, retyping a more considerable function every time we want to tweak its functionality could be confusing.
Add Parameters to Functions in PowerShell
Functions can have any number of parameters. When we create our functions, we will have the option to include parameters and decide how those parameters work.
The parameters can be either optional or mandatory, and they can either be forced to accept one of a limited list of arguments or accept anything.
For example, the fictional software we install via the Install-Software
function might have many possible versions earlier. But currently, the Install-Software
function offers the user no way to specify which version they want to install.
If we were the only one using the function, we could change the code inside it each time we wanted a specific version, but that would consume a lot of time. This method would also be prone to potential errors, and we want others to be able to use our code.
Introducing parameters into our function allows it to have variability. Just as variables allowed us to write scripts that could handle many versions of the same situation, parameters will enable us to write a single function that does one thing in many ways.
In this case, we want it to install versions of the same software and do so on many computers.
First, let’s add a parameter to the function that enables a user or us to specify the version to install.
Create a Simple Parameter on a Function in PowerShell
Defining a parameter on a function requires a parameter block. The parameter block holds all the parameters for the function.
Define a parameter block with the param
keyword followed by parentheses, as shown below.
Example Code:
function Install-Software {
[CmdletBinding()]
param()
Write-Host 'The software has been installed.'
}
At this point, our function’s actual functionality hasn’t changed a bit. We have been just installed the plumbing, preparing the function for a parameter.
Once we have added the param
block, we can create the parameter by putting it within its parentheses, as shown below.
Example Code:
function Install-Software {
[CmdletBinding()]
param(
[Parameter()]
[string] $Version
)
Write-Host "The software version $Version has been installed."
}
Inside the param
block above, we would first define the Parameter
block. Using the Parameter()
block will turn the parameter into an “advanced parameter”.
An empty param
block like the one here does nothing but is required; we’ll explain how to use it in the next section.
Let’s focus on the [string]
type in front of the parameter name. We cast the parameters by putting the parameter’s type between square brackets before the parameter variable name.
PowerShell will always default values to a string. Above, anything passed in the variable $Version
will always be treated as a string.
We also add $Version
into our Write-Host
statement. Then, when we run the Install-Software
function with the Version
parameter and pass it a version number, we should get a message showing so.
Example Code:
Install-Software -Version 2
Output:
The software version 2 has been installed.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn