PowerShell With Robocopy and Arguments Passing
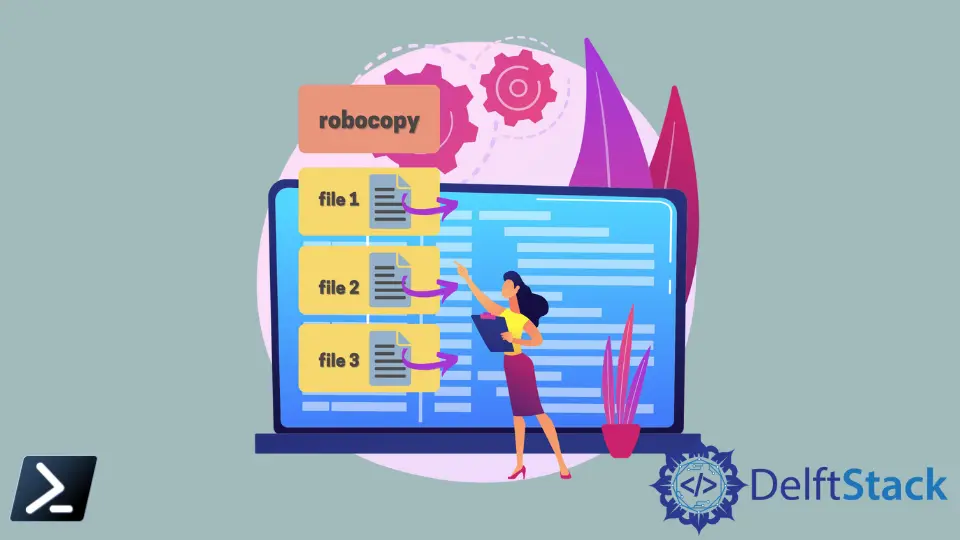
In the expansive landscape of Windows PowerShell, mastering the robocopy
command unveils a wealth of capabilities for proficient file management. As an indispensable tool in the arsenal of IT professionals, developers, and system administrators alike, Robocopy offers unparalleled versatility and efficiency in copying files and directories.
Its robust feature set, coupled with the flexibility of PowerShell scripting, empowers users to execute complex file transfer operations with precision and ease. This tutorial will teach you to use robocopy
in PowerShell.
Use the robocopy
Command to Copy Files in PowerShell
Robocopy, short for “Robust File Copy”, is a command-line utility native to Windows operating systems, renowned for its efficiency and versatility in copying files and directories. Developed by Microsoft, Robocopy offers a wide range of features that surpass the capabilities of traditional file copying methods, making it a preferred choice for system administrators, IT professionals, and power users alike.
The general syntax of the robocopy
command is:
robocopy <Source> <Destination> [<File(s)>] [<Options>]
<Source>
: Specifies the path to the source directory.<Destination>
: Specifies the path to the destination directory.[<File(s)>]
(optional): Specifies one or more files to be copied.[<Options>]
(optional): Specifies various options and parameters for the copy operation.
The following is a simple robocopy
command example.
robocopy "C:\SourceFolder" "D:\DestinationFolder" example.txt /njh /njs
In this code, we use the robocopy
command followed by the source and destination paths. We also specify the file to be copied and two options, /njh
and /njs
, which suppress the display of the job header and job summary information in the output, respectively.
Output:
For more information on robocopy
options, read this article.
Let’s have a look at another robocopy
command example. The following variables store the value for the robocopy
arguments.
$source = "C:\SourceFolder"
$destination = "C:\DestinationFolder"
$robocopyOptions = "/njh"
$file = "example.txt"
robocopy $source $destination $file $robocopyOptions
In the provided code snippet, we utilize variables to store the source and destination paths, as well as the desired Robocopy options and the file to be copied. By passing these variables to the robocopy
command, we initiate the copying process, allowing for the efficient transfer of the specified file from the source to the destination directory.
Output:
The above command will not work when you store multiple options in the $robocopyOptions
variable.
$robocopyOptions = "/njh /njs"
You have to split the strings in the command like this.
$source = "C:\SourceFolder"
$destination = "C:\DestinationFolder"
$robocopyOptions = "/njh /njs"
$file = "example.txt"
robocopy $source $destination $file $robocopyOptions.split(' ')
In the provided code snippet, we utilize variables to store the source and destination paths, along with the desired Robocopy options. By splitting the $robocopyOptions
string into an array using the .split(' ')
method, we ensure that each option is treated as a separate element.
This array is then passed as arguments to the robocopy
command, enabling the execution of the copy operation with the specified parameters.
Output:
Conclusion
Delving into the intricacies of the robocopy
command within PowerShell unlocks a world of possibilities for optimizing file management workflows. By comprehending its syntax, exploring diverse parameters, and harnessing the power of variables, users can streamline file transfer operations to meet the demands of diverse scenarios.
Whether it’s replicating files across network shares, synchronizing directories between servers, or backing up critical data, Robocopy stands as a stalwart ally, facilitating seamless and efficient file management within the Windows environment. As you embark on your journey with PowerShell Robocopy, embrace its capabilities to elevate your file management practices to new heights of efficiency and productivity.