How to Read Excel Sheet Data in PowerShell
- Method 1: Using COM Objects to Read Excel Data
- Method 2: Using Import-Excel Module
- Method 3: Reading Specific Ranges from Excel
- Conclusion
- FAQ
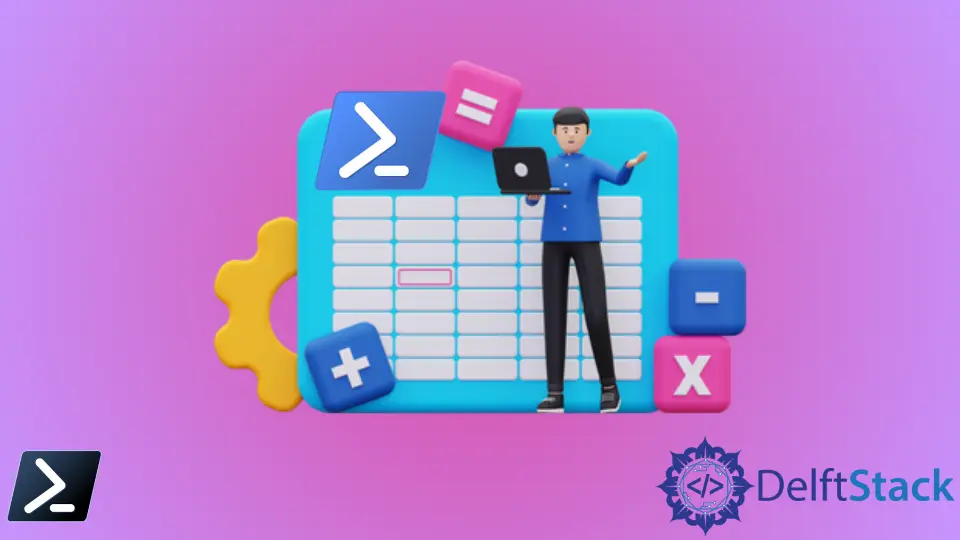
In today’s data-driven world, the ability to efficiently read and manipulate Excel sheet data is essential for many professionals. PowerShell, a powerful scripting language, provides an effective way to automate tasks, including reading data from Excel workbooks. Whether you’re managing reports, analyzing datasets, or just looking to streamline your workflow, knowing how to leverage PowerShell for Excel can save you time and effort.
In this article, we’ll explore the fundamentals of using PowerShell to read Excel sheet data, offering practical examples and clear explanations to help you get started. Let’s dive in!
Method 1: Using COM Objects to Read Excel Data
One of the most straightforward methods to read Excel data in PowerShell is by using COM objects. This approach allows you to interact with Excel directly, enabling you to open workbooks, read cell values, and even manipulate data if needed.
Here’s a simple example of how to read data from an Excel file:
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open("C:\path\to\your\file.xlsx")
$sheet = $workbook.Sheets.Item(1)
$data = $sheet.Cells.Item(1, 1).Value()
$excel.Quit()
$data
Output:
Your data from the first cell
In this script, we create a new instance of the Excel application using COM objects. We then open the specified Excel file and access the first sheet. The Cells.Item(1, 1).Value()
method retrieves the value from the first cell (A1). After obtaining the data, we ensure to quit the Excel application to free up resources. This method is particularly useful for quick data retrieval tasks, but keep in mind that it requires Excel to be installed on your machine.
Method 2: Using Import-Excel Module
For those who prefer a more user-friendly approach, the Import-Excel
module provides an excellent alternative. This method simplifies the process of reading Excel data without needing to interact with COM objects directly.
First, you need to install the Import-Excel
module if you haven’t already:
Install-Module -Name ImportExcel
Once installed, you can easily read data from an Excel file using the following command:
$data = Import-Excel -Path "C:\path\to\your\file.xlsx"
$data
Output:
Name Age
---- ---
Alice 30
Bob 25
In this example, we use the Import-Excel
command to read the entire contents of the specified Excel file. The result is automatically formatted into a PowerShell object, making it easy to access and manipulate the data. You can filter, sort, and analyze this data using standard PowerShell commands, which makes this method highly efficient for handling larger datasets. This approach is particularly beneficial for users who may not be as familiar with Excel’s COM objects but still need to work with Excel data regularly.
Method 3: Reading Specific Ranges from Excel
Sometimes, you may only want to read specific ranges from an Excel sheet rather than the entire dataset. Using the Range
property, you can target specific cells or ranges to retrieve only the data you need.
Here’s how to do it:
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open("C:\path\to\your\file.xlsx")
$sheet = $workbook.Sheets.Item(1)
$range = $sheet.Range("A1:B2").Value()
$excel.Quit()
$range
Output:
Name Age
---- ---
Alice 30
Bob 25
In this script, we specify the range of cells we want to read using the Range
property. In this case, we are retrieving data from cells A1 to B2. The output will contain only the specified range, which is particularly useful when working with large spreadsheets where you only need a subset of the data. As with the previous methods, remember to quit the Excel application to avoid keeping Excel running in the background.
Conclusion
Reading Excel sheet data in PowerShell can significantly enhance your productivity and streamline your workflow. Whether you choose to use COM objects for direct interaction or the Import-Excel
module for a more straightforward approach, PowerShell provides versatile options for managing Excel data. By mastering these techniques, you can automate repetitive tasks, analyze datasets efficiently, and make data-driven decisions with ease. Start experimenting with these methods today, and unlock the full potential of PowerShell for your data management needs!
FAQ
-
Can I use PowerShell to write data to Excel?
Yes, you can write data to Excel using both COM objects and theExport-Excel
command from theImport-Excel
module. -
Do I need Excel installed to use PowerShell for reading Excel files?
Yes, if you are using COM objects, Excel must be installed on your machine. However, theImport-Excel
module does not require Excel to be installed. -
What versions of Excel does PowerShell support?
PowerShell can interact with any version of Excel that supports COM automation, typically Excel 2007 and later. -
Is the
Import-Excel
module available for all versions of PowerShell?
TheImport-Excel
module is compatible with Windows PowerShell 5.1 and PowerShell Core. -
Can I read Excel files from SharePoint using PowerShell?
Yes, you can read Excel files stored in SharePoint using PowerShell by accessing the SharePoint API or using the SharePoint PnP PowerShell module.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn