How to Display Message Box in PowerShell
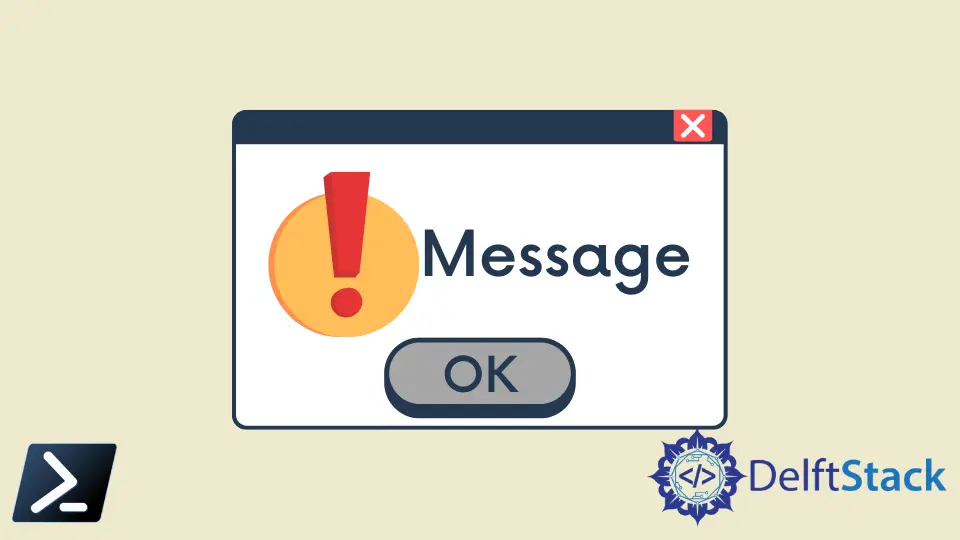
Some people think PowerShell is a command-line language. They believe that PowerShell can output text to a dull console screen.
However, Windows PowerShell is built on top of the .NET framework. Therefore, it can use graphical user interfaces (GUIs) for your scripts.
This article will discuss the valuable functions of PowerShell GUI called Message Boxes
, and we will learn how to write and output them using PowerShell.
Introduction to PowerShell GUI
A couple of ways exist to transform our scripts into GUI form. If we need to create simple GUI tools to interact with the user in Windows PowerShell, we will probably choose Windows Forms (WinForms) or Windows Presentation Foundation (WPF).
However, there are times when we don’t need to go to the extent of building fully-featured GUI tools.
For example, we wouldn’t want to figure out how to make forms and place controls on your forms when we only desire to display a message to your user for general information or get some simple input.
In this case, there’s another more straightforward method that only requires a single line of code; [System.Windows.MessageBox]
.
the MessageBox
Class in PowerShell
The MessageBox
class within the System.Windows
namespace has many options that allow us to show the user some text with an OK
button to ask for input and give them options like Yes
, No
, or Cancel
.
Firstly, this namespace doesn’t exist by default in Windows PowerShell. So, to use it, we will need to add the assembly into our Windows PowerShell session.
We will do this by adding the PresentationFramework
assembly using the Add-Type
cmdlet.
Add-Type -AssemblyName PresentationFramework
Once this is added, we can now use the MessageBox
class.
The MessageBox
class has a primary method called Show
. This method allows us to pass different parameters to it to behave differently.
For example, you can use the Show()
method to display a simple message in its simplest form.
[System.Windows.MessageBox]::Show('Hello')
When the message box comes up, we will notice that it will stop your console input until it is closed. When we click OK
, the console will then show you what the Show()
method returned, which, in this case, is simple 'OK'
.
The Show()
method has many constructors to customize things like the message box title, the type of buttons displayed, etc. Let’s go over one example.
First, we’ll add a title and icon, and we’ll add Yes
, No
, or Cancel
buttons to get input from the user.
We will add a few parameters to the Show()
method.
[System.Windows.MessageBox]::Show('Do you want to proceed?', 'Confirm', 'YesNoCancel', 'Error')
We can see that as long as we place the message to be shown, the title of the message box, the button set, and the type of icon to be displayed in the proper parameter order, we can offer different kinds of message boxes.
Then, depending on the button that we press, it will return a separate output that we can then use to decide on the future flow of your script.
$msgBoxInput = [System.Windows.MessageBox]::Show('Would you like to proceed?', 'Confirmation', 'YesNoCancel', 'Error')
switch ($msgBoxInput) {
'Yes' {
## Action Here
}
'No' {
## Action Here
}
'Cancel' {
## Action Here
}
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn