How to Log Off Remote Sessions in PowerShell
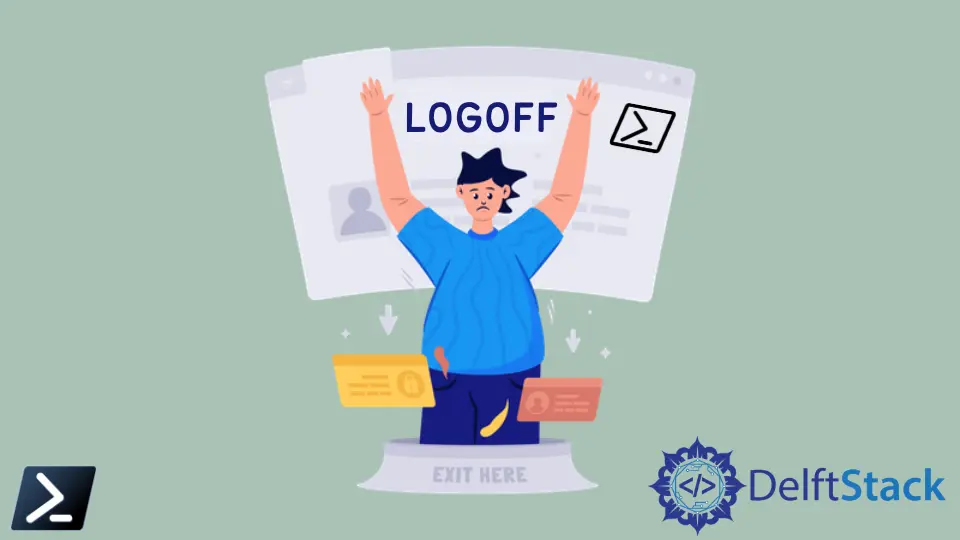
As an administrator of an organization, it is a common issue in a workplace where a specific server or workstation may experience degradation of performance.
This situation is usually caused by multiple users connecting remotely to the machine, especially if this is a shared workstation. However, most users remotely connecting do not log off properly and just hit the close button on their Remote Desktop Connection or RDP.
If the user doesn’t log off from the machine properly, their profile will remain active and take up resources. As an administrator, we can remediate this by performing log off operations.
This article will discuss how we can log off remote users using the command line and PowerShell.
Log Off Remote Sessions in PowerShell
Programmatically logging off remote sessions is easy. However, it is better to use legacy commands instead of the built-in PowerShell command modules in this use case.
For example, the PowerShell module Invoke-RDUserLogoff
command has its limitations, like being only executed on a domain-joined computer and operating systems Windows Server 2012 R2 or 8.1 and below.
For now, let us focus on a couple of legacy commands to log off remote users that it is still widely used up to this day.
the logoff
Command
The logoff
command will terminate a user’s current session in a local or remote server or workstation. If we run the help command with the code below, we can see that the logoff
cmdlet uses a few parameters like the session name or id and the server name, which are both critical.
Example Code:
logoff /?
Output:
LOGOFF [sessionname | sessionid] [/SERVER:servername] [/V] [/VM]
If we already know which server to target, how could we know the current session on that machine? We will now then use the query user
command or quser
.
Query for the User Session Using the quser
Command
For now, try running quser
on your local machine with the code snippet below.
Example Code:
quser
Output:
USERNAME SESSION NAME ID STATE IDLE TIME LOGON TIME
>marion console 1 Active none 6/10/2022 5:56 AM
Running the quser
command will display all of the current sessions on your computer. However, how can we isolate a specific user’s session id on a remote server without actually logging in to that server?
Remember that we can execute the quser
command locally while targeting a remote computer. All it needs is an administrator account that has the right to connect to computers remotely.
From this point is where we will need Windows PowerShell.
We will need PowerShell for the snippet below to perform string operations like -split
. In addition, the output below will display the user’s session id, which we need as a parameter for the logoff
command.
Example Code:
((quser /server:RDP01 | ? { $_ -match "marion" }) -split ' +')[3]
Output:
1
It is worth noting that we can run legacy commands in PowerShell like quser
and logoff
. So, from here on, we will now use the PowerShell terminal instead of the command prompt as our interpreter.
Log Off Users Using the quser
and logoff
Commands
Now that we know how to get a user’s session ID without establishing a remote session on the computer, we can combine all commands inside PowerShell. We may follow the snippet of code below as an example.
Set-Alias Query-User quser
Set-Alias Logoff-User logoff
$serverName = 'RDP01'
$userName = 'marion'
$sessionID = ((Query-User /server:$serverName | ? { $_ -match $userName }) -split ' +')[3]
Logoff-User $sessionID /server:$serverName
As you can notice, we have created new aliases for both quser
and logoff
commands. This step is optional, but we have done this to immerse ourselves with PowerShell scripting even more by using the Verb-Noun command format.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn