How to Get Logged on Users in PowerShell
- Use the WMI Class to Get Logged on Users in PowerShell
- Use the .NET Environment Class to Get Logged on Users in PowerShell
-
Use the
query
Command to Get Logged on Users in PowerShell
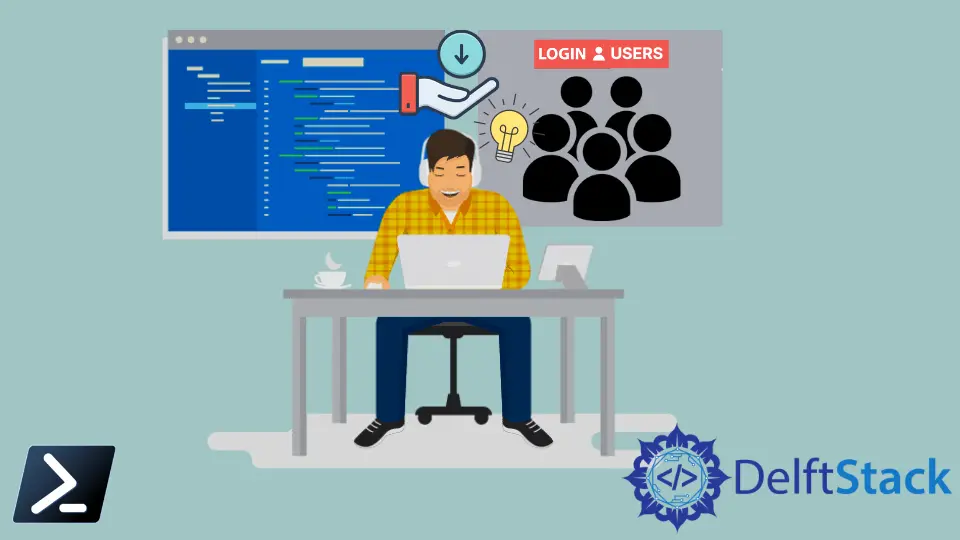
When we work with Windows, at some point, we’ll probably need to find out which users are currently logged on to a computer. Luckily, we can use PowerShell to get current users on local or remote computers.
This article will discuss several ways to use PowerShell to get current logged-in users on a computer. The methods we know will include native commands, .NET classes, and Windows Management Instrumentation (WMI) specific cmdlets.
Use the WMI Class to Get Logged on Users in PowerShell
Let us start with the PowerShell native cmdlets called Get-WMIObject
and Get-CimInstance
. These cmdlets allow us to fetch information, including the currently logged-on user, using the Windows Management Instrumentation or WMI classes on a local or remote computer.
Get-WMIObject
is the older (deprecated) cmdlet and is only available to Windows PowerShell version 5.1. Conversely, the Get-CIMInstance
command is the newer, more secure cmdlet and is open to the latest PowerShell versions.To use Windows PowerShell to get the current user, invoke either command targeting the Win32_ComputerSystem
class. The Win32_ComputerSystem
.NET class includes various properties, including the Username property.
To do so, open a Windows PowerShell window and run the commands below.
Example code:
# Use any of the commands
(Get-WMIObject -ClassName Win32_ComputerSystem).Username
(Get-CimInstance -ClassName Win32_ComputerSystem).Username
It is worth noting that querying the Win32_ComputerSystem
.NET class will only output the username of the currently logged-on user onto the computer (console session). Therefore, if the user logs on remotely (e.g., Remote Desktop), the username will be blank.
Both cmdlets return the same output, as shown below. If the currently logged-in user is a domain user account, the command will return the domain and the username (domain\username).
But for a local account, the output would be a local computer together with the username.
Output:
DOMAIN\User01
The Get-WMIObject
and Get-CimInstance
commands have a parameter called -ComputerName
, which accepts the computer’s name to query. Using this parameter means that we can query the same information from a remote machine in the network.
If we need to get only the user’s username without the domain, a solution is to separate the output using the split()
method.
The snippet below will split the username property into two using the backslash (\
) character as the delimiter. The [1]
part will use the cmdlet to output only the result at index [1].
It is worth noting that indexes start with 0; specifying index one will show the result at the second position.
Example code:
((Get-WMIObject -ClassName Win32_ComputerSystem).Username).Split('\')[1]
Windows PowerShell outputs the user part only, like the output below.
Output:
User01
Use the .NET Environment Class to Get Logged on Users in PowerShell
PowerShell is an object-oriented language and shell. Everything that we work on is an object.
When we run a command, the output is an object. If we declare a variable, that variable is an object.
These objects have a hierarchy that defines the proper data type, similar to blueprints. These said blueprints are called .NET classes or simply classes.
The class associated with it is the .NET Environment
class. So how do we use the Environment
class in PowerShell to get the current user?
The Environment class contains a Username
property, whose value is the currently logged-in user’s username. To retrieve the username property value, run the command below in PowerShell.
Example code:
[System.Environment]::UserName
Aside from the Username
property, the Environment
class also contains a process to get the value of an environment variable. The method’s name is GetEnvironmentVariable
function.
To retrieve the current user using this method, run the command below.
Example code:
[System.Environment]::GetEnvironmentVariable('username')
Output:
User01
Use the .NET WindowsIdentity Class to Get Logged on Users in PowerShell
The WindowsIdentity
.NET class is another .NET class we can invoke in Windows PowerShell to retrieve the current user. This class has a method called the GetCurrentName
function, which outputs an object that represents the current Windows user.
To retrieve the current user details, run the command below.
Example code:
[System.Security.Principal.WindowsIdentity]::GetCurrent()
The cmdlet returns the current user object properties, including the Name
property. The Name
property value follows the domain\user format.
Use the query
Command to Get Logged on Users in PowerShell
If we are a system admin or a domain user, we’ve logged on to a remote machine via the Remote Desktop Protocol or RDP at least once, perhaps to manage resources or use applications. Suppose we want to know who is currently logged on and using system resources.
Windows has a built-in command-line tool called the query
command to list all the currently logged-on users on a computer. The command also shows us if the user logged on via a remote desktop session or locally to the computer.
The query
legacy command contains two parameters pertinent to getting the logged-on users; session
and user
. The session
parameter displays all the computer sessions, while the user
parameter displays the users and their sessions.
For example, execute the command below to list the sessions on the current server.
Example code:
query user /server:$server
Output:
USERNAME SESSION NAME ID STATE IDLE TIME LOGON TIME
user01 console 1 Active none 3/27/2022 2:36 PM
After running the command, we’ll notice that quser
returns the sessions, but there are no blank sessions this time. The command also shows the users’ idle time and login timestamp.
Compared with other commands, the quser
is the more appropriate command in PowerShell to get current users.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn