How to Create an Input Box in PowerShell
-
Introduction to
Read-Host
Command in PowerShell - Use the Secure String Parameter in PowerShell
- Use the Visual Basic Library in PowerShell
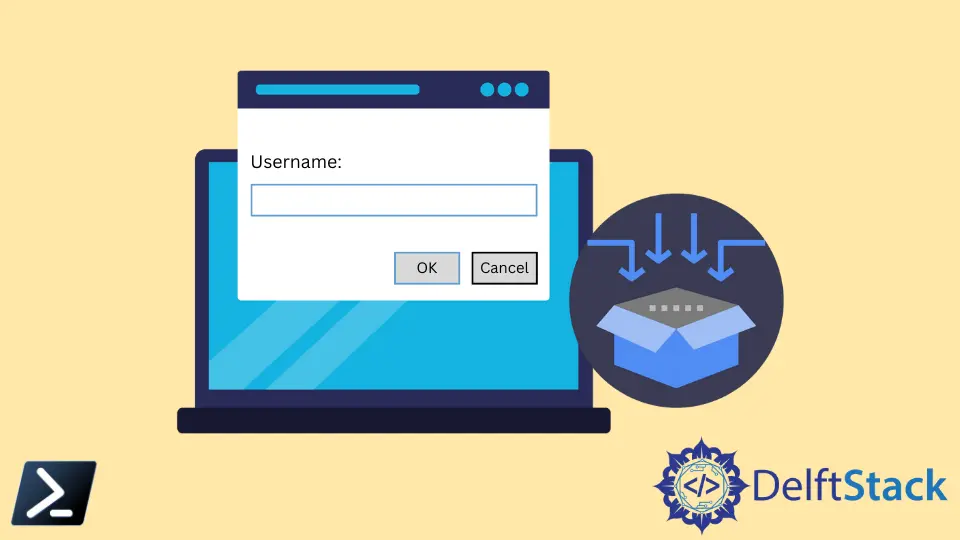
In PowerShell, the script can retrieve the input from the user by prompting them with the Read-Host
command. It acts like the stdin
command and reads the information supplied by the user from the command line.
Since the user’s input can also be stored as a secured string, passwords can also be prompted using this cmdlet.
In regular PowerShell or ISE, a colon is displayed at the end of the prompt requesting input in a more GUI enhanced ISE. In addition, a pop-up is displayed along with a few buttons.
This article will explain getting user input in PowerShell using the prompt and how to generate an input box using PowerShell GUI.
Introduction to Read-Host
Command in PowerShell
The Read-Host
command is the most basic PowerShell native command that we can use to ask for user input.
Example Code:
Read-Host
Basic Syntax:
Read-Host [[-Prompt] <Object>] [-AsSecureString] [<CommonParameters>] ALIASES
None
The Read-Host
command has three parameters that we can use.
-AsSecureString
- This parameter indicates the input typed by the user is hidden with *
. When this parameter is used, the output is a specific string object.
- The data type of the parameter value is
Switch
. - The parameter’s default value is
NULL
. - Wild card characters are not accepted.
-MaskInput
- This parameter is like the secure string parameter in functionality, except that the output is a string, not a secure string.
- The data type of the parameter value is
Switch
. - The parameter’s default value is
NULL
. - Wild card characters are not accepted.
-Prompt
- This parameter denotes the script’s prompt text to display to the user. This parameter value needs to be a string.
In the case of spaces, they should be enclosed within quotes.
- The data type of the parameter is
PSObject
. - The parameter’s default value is
NULL
. - Wild card characters are not accepted.
Use the Secure String Parameter in PowerShell
When executing the Read-Host
command, its normal behavior will ask for user input on the command line. However, using the AsSecureString
parameter is one of the easiest ways to generate a simple GUI that asks for a user’s input.
To do this, run the following snippet below.
$UserName = Read-Host 'Enter Your User Name:' -AsSecureString
Output:
The only downside of this is that the input is masked like a password. This method is perfect if we are asking for the user’s password.
However, what if we ask for something that we can show in plain text, like perhaps a username or an employee ID.
What if we want to customize our input box and fields that don’t need the AsSecureString
parameter. We will discuss the method to achieve this process in the article’s next section.
Use the Visual Basic Library in PowerShell
One of the newest ways of asking for input from the user with a GUI interface is by loading the Microsoft Visual Basic Interaction class with the Add-Type
cmdlet.
Example Code:
Add-Type -AssemblyName Microsoft.VisualBasic
$User = [Microsoft.VisualBasic.Interaction]::InputBox('Username:', 'User', "Enter username here")
The InputBox
function accepts three parameters, as we see in the snippet above.
- The first parameter is the label or the name of the text field.
- The second parameter is the variable’s name on where we will store the value of the provided user input.
- The third parameter is the default value of the text field.
Output:
Once the user fills in the text box with user input and presses the OK
button, the $User
variable will contain the value in the textbox.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn