How to Get the Value of a Registry Key Using PowerShell
- What Is a Registry Key
- Backing Up Registry Keys in PowerShell
-
Getting a Value of a Registry Key Using
Get-ItemProperty
Cmdlet in PowerShell -
Getting a Value of a Registry Key Using
Get-ItemPropertyValue
Cmdlet in PowerShell
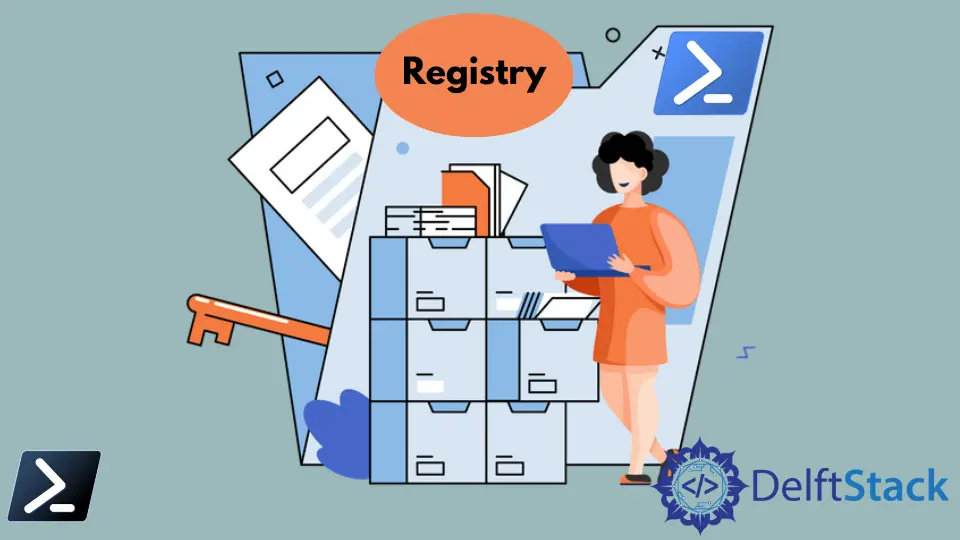
There are instances where fixing errors, tweaking Windows features, or completely uninstalling software or a product, requires you to edit the Windows registry.
While using the Registry Editor may be convenient, an error may still occur when we are not careful enough. Editing the registry is very delicate, and your local system is dependent on it.
This article will show you how to safely get registry key values using Windows PowerShell.
What Is a Registry Key
A registry key can be considered a bit like a structured file folder, but it exists only within the Windows Registry.
In addition, registry keys may also contain registry values, just like folders contain files. Registry keys can also include other registry keys, usually referred to as subkeys.
As mentioned, the Windows Registry is structured, with the topmost registry keys referred to as the registry hives. These are considered special registry keys with special permissions, but they still have the same essential function as a standard registry key.
HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft
If we take the example above, HKEY_LOCAL_MACHINE
or HKLM
for short, is the top of the path of the registry. The SOFTWARE
registry key then succeeds it, and as mentioned, registry keys can contain more keys in them which we call subkeys such as Microsoft
.
Backing Up Registry Keys in PowerShell
It’s wise to back up our registry before we make any changes. As mentioned, any accidental change within the registry may cause an error to your files, software, or even worse, the whole operating system.
However, with a copy of the keys you’re changing, you can feel comfortable knowing you can revert any changes that might result in problems in your operating system.
We may use the legacy commands reg export
to quickly backup your registry. For example, the syntax below will backup the whole HKLM
registry.
reg export HKLM C:\RegBack\HKLM.Reg /y
Your backed-up registry keys will export in a .REG
file format. Therefore, you can quickly restore backed-up registry keys by double-clicking the registry file and following the prompts.
Getting a Value of a Registry Key Using Get-ItemProperty
Cmdlet in PowerShell
The Get-ItemProperty
is a PowerShell cmdlet used to return registry entries in a more readable format than its relative command Get-Item
. We can also get the value of a specific registry key using the Get-ItemProperty
cmdlet.
Example Code:
$registryPath = "HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion"
Get-ItemProperty -Path $registryPath -Name ProgramFilesDir
Output:
ProgramFilesDir : C:\Program Files
PSPath : Microsoft.PowerShell.Core\Registry::HKEY_LOCAL_MACHINE\SOFTWARE\Micr
osoft\Windows\CurrentVersion
PSParentPath : Microsoft.PowerShell.Core\Registry::HKEY_LOCAL_MACHINE\SOFTWARE\Micr
osoft\Windows
PSChildName : CurrentVersion
PSDrive : HKLM
PSProvider : Microsoft.PowerShell.Core\Registry
""
) as some registry paths have spaces in them. Enclosing the path in double quotes will take the literal expression of the said path and will not result in an error.The command creates a PSCustomObject
object with PsPath
, PsParentPath
, PsChildname
, PSDrive
, PSProvider
, and another property that is named after the name of the key value.
So, to output just the actual value of the registry key, we can call the key value name property into our script.
Example Code:
$registryPath = "HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion"
$keyValue = Get-ItemProperty -Path $registryPath -Name ProgramFilesDir
$keyValue.ProgramFilesDir
Output:
C:\Program Files
The command Get-ItemProperty
works on PowerShell Versions 2 to 5. However, in Windows PowerShell 5, Microsoft introduced a new cmdlet that makes printing registry keys in the command-line much simpler.
Getting a Value of a Registry Key Using Get-ItemPropertyValue
Cmdlet in PowerShell
As mentioned, the Get-ItemPropertyValue
cmdlet is introduced in Windows PowerShell version 5 to address the query of getting the value of registry keys in a much shorter and more straightforward way.
The cmdlet only takes two parameters to work: the registry file path and the registry key that needs to be queried for a value.
Example Code:
Get-ItemPropertyValue 'HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion' 'ProgramFilesDir'
Output:
C:\Program Files
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn