How to Check if User Exists in PowerShell
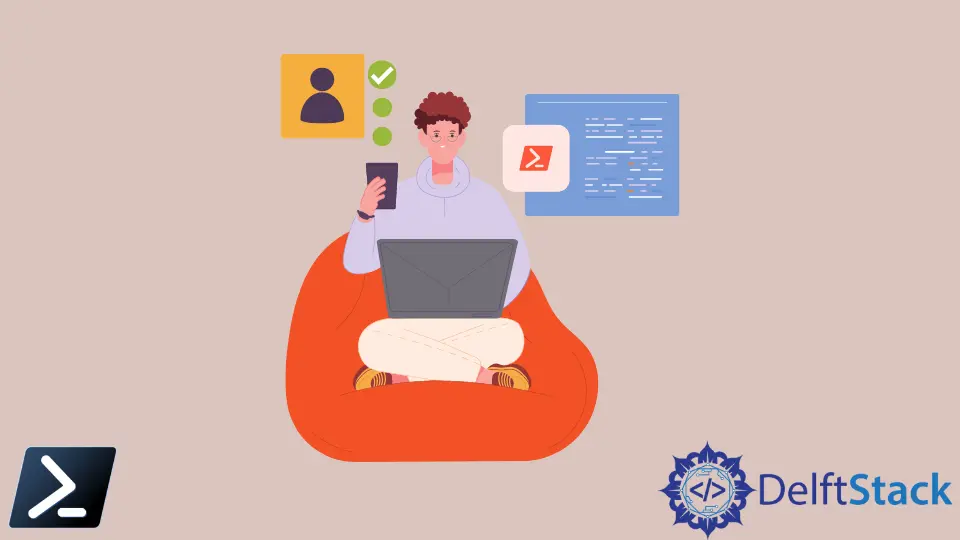
This tutorial demonstrates how to check if a user exists in PowerShell.
PowerShell Check if User Exists
PowerShell provides a command based on the active directory, which is used to check whether a user exists. To use this command, first, we need to import the module ActiveDirectory
; the syntax for this command is below.
Get-ADUser
Now follow the steps below to check if a user exists using PowerShell:
-
First, we need to import the module
ActiveDirectory
. Run the following command:Import-Module ActiveDirectory
-
Once the module is imported, we assign the user name to a variable.
-
Then, we create a user variable using the
Get-ADUser
in atry-catch
block. -
Finally, we check the user variable using the
if-else
condition to determine if it is null or has some value. -
Run the following commands for the above three steps:
$UserName = "Sheeraz"
$CheckUser = $(try { Get-ADUser $UserName } catch { $null })
If ($CheckUser -ne $Null) {
"User exists in the Active Directory"
}
Else {
"User not found in the Active Directory"
}
The above code will check for the user in the active directory and print the output based on the result. See the output:
User not found in the Active Directory
We can also check multiple users at once. Follow the steps below to check if multiple users exist in the active directory or not:
-
First of all, create an object of users that includes all users’ names.
-
Then run a
foreach
loop to check each user. -
Use
try-catch
blocks to check that each user is similar to the above method. -
Use the
if-else
condition to print the results. See the command based on these steps.
$CheckUsers = @("Sheeraz", "Jhon", "Mike")
foreach ($CheckUser in $CheckUsers) {
$UserObject = $(try { Get-ADUser $CheckUser } catch { $Null })
If ($UserObject -ne $Null) {
Write-Host "The user $CheckUser already exists" -ForegroundColor "green"
}
else {
Write-Host "The user $CheckUser does not exists " -ForegroundColor "red"
}
}
What if we have a large number of users to check? We can use a CSV file with the Get-ADUser
command to check many users.
Follow the steps below:
-
First of all, create an empty object of results.
-
Import the CSV with user names.
-
Then run a
foreach
loop to check each user. -
Use
try-catch
blocks to check that each user is similar to the above method. -
Use the
if-else
condition to show the results. See the command based on these steps.
$UsersResult = @()
Import-Csv 'C:\Users\Admin\demo.csv' | ForEach-Object {
$CheckUser = $_."UserName"
$UserObject = $(try { Get-ADUser $CheckUser } catch { $Null })
If ($UserObject -ne $Null) {
$ExistUser = $true
}
else {
$ExistUser = $false
}
$UsersResult += New-Object PSObject -Property @{
UserName = $CheckUser
ExistUser = $ExistUser
}
}
$UsersResult | select UserName, ExistUser
Our CSV file contains 20 names of different users. The code above will check for each user and print true
if the user exists and print false
if the user doesn’t exist.
See the output:
UserName ExistUser
-------- ---------
False
False
False
False
False
False
False
False
False
False
False
False
False
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook