How to List Local Users and Groups Using PowerShell
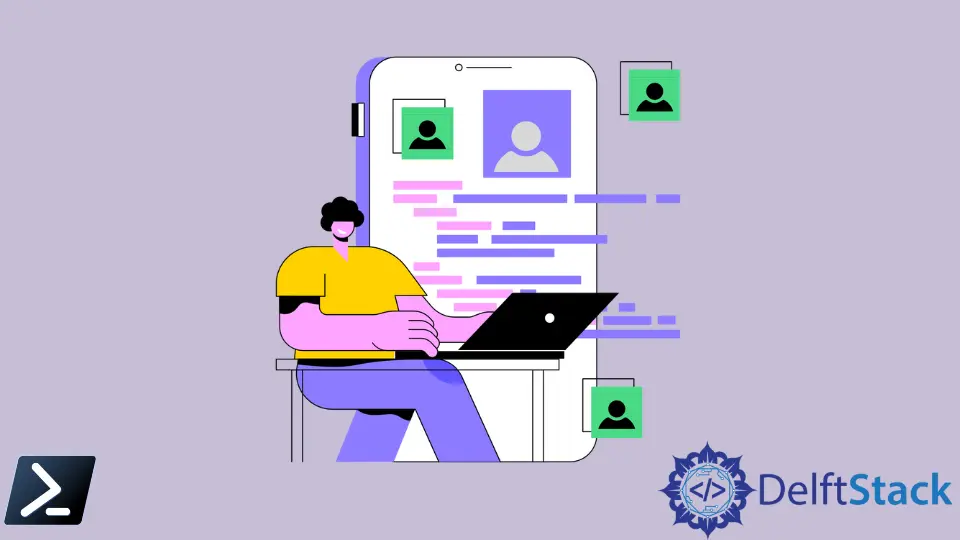
This article will discuss how to query local users and groups in our machine with PowerShell.
List Local Users and Groups Using PowerShell
When there’s a domain disconnection due to network issues, most of the time, our domain credentials will not work due to the unreachability and unavailability of the authentication server. Thus, as the best standard, we should have our local credentials handy, and as administrators, these credentials are part of the local administrator’s group.
We can use PowerShell scripting to check if we have administrator credentials, especially since we can also use these commands to process multiple machines in bulk. The following section will discuss several ways to query the local users and groups using PowerShell.
Get a User’s Local Group Membership With ADSI
According to Microsoft, Active Directory Service Interfaces (ADSI) are built-in COM interfaces used to access directory services. For example, within the ADSI library is the [ADSI]
class that we can run in Windows PowerShell to query a group membership of a current user.
Example Code:
$adsi = [ADSI]"WinNT://$env:COMPUTERNAME"
$adsi.Children | where { $_.SchemaClassName -eq 'user' } | ForEach-Object {
$groups = $_.Groups() | ForEach-Object { $_.GetType().InvokeMember("Name", 'GetProperty', $null, $_, $null) }
$_ | Select-Object @{Name = 'UserName'; Expression = { $_.Name } }, @{Name = 'Group'; Expression = { $groups -join ';' } }
}
Output:
UserName Groups
-------- ------
Administrator Administrators
DefaultAccount System Managed Accounts Group
Guest Guests
user Users
Get a User’s Local Group Membership With WMI
WMI is a collection of guidelines for streamlining hardware and software management across a network using Windows-based computers, and it’s pre-installed on Microsoft’s newest operating systems. The vendor provided a command-line interface (CLI) for WMI known as WMI Command Line (WMIC) in OSs before Windows 10.
WMIC is compatible with existing shells and utility commands in these previous versions of Windows.
Example Code:
Get-WmiObject -Class Win32_UserAccount -Filter "LocalAccount='True'"
Output:
AccountType : 512
Caption : DESKTOP-7GI1260\Administrator
Domain : DESKTOP-7GI1260
SID : S-1-5-21-3848050931-464278446-3624840-500
FullName :
Name : Administrator
AccountType : 512
Caption : DESKTOP-7GI1260\user
Domain : DESKTOP-7GI1260
SID : S-1-5-21-3848050931-464278446-3624840-1003
FullName :
Name : user
The -Filter
parameter is in place to only filter accounts that are created locally. Therefore, it is not formed through default account creation when the domain has been established.
Get a User’s Local Group Membership With Get-Local
Commands
PowerShell 5.1 can now use the Get-LocalGroupMember
, Get-LocalGroup
, Get-LocalUser
, and other Get-Local*
commands in the Microsoft.PowerShell.LocalAccounts
module to get and map local users and groups.
Using these new commands makes it more concise when writing our script.
Example Code:
Get-LocalGroupMember -name users
Output:
ObjectClass Name PrincipalSource
----------- ---- ---------------
User DESKTOP-7GI1260\kentm MicrosoftAccount
Group NT AUTHORITY\Authenticated Users Unknown
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn