How to Pass Boolean Parameters to a PowerShell Script From a Command Prompt
- Use Boolean Parameter to Pass Boolean Values to a PowerShell Script From a Command Prompt
- Use String Values to Pass Boolean Values to a PowerShell Script from the Command Prompt
- Conclusion
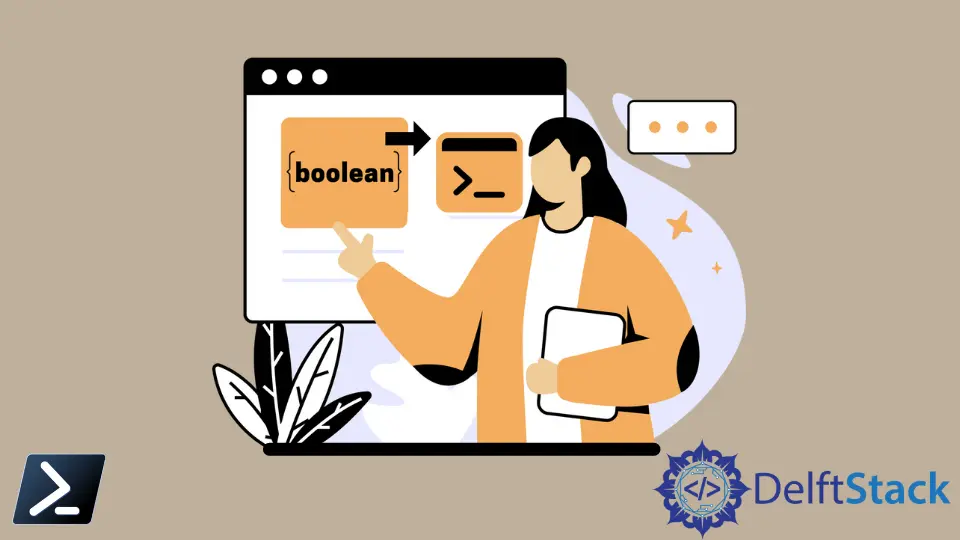
A PowerShell script is a structured sequence of commands stored in a text file with the .ps1
extension. When executed, PowerShell processes these commands one by one, allowing users to automate various tasks.
To make scripts more versatile and adaptable, you can define parameters using the param
statement within your PowerShell code. When you define a parameter in your script, you create an entry point for passing data to the script when it is executed.
Let’s illustrate this with a straightforward example. Consider a PowerShell script named YourScript.ps1
, which takes user-input values.
param (
$a, $b
)
if ($a -lt $b) {
Write-Host "$a is less than $b"
}
else {
Write-Host "$a is not less than $b"
}
To execute this script and pass values from the command prompt, you can use the following command:
powershell.exe -NoProfile -Command .\YourScript.ps1 -a 4 -b 5
Upon running this, you’ll receive the following output:
4 is less than 5
This showcases how to pass values to a PowerShell script efficiently, providing users with the capability to customize the script’s behavior.
Now, let’s delve into the topic of passing Boolean
values to a PowerShell script from the command prompt. A Boolean
value can represent either TRUE
or FALSE
, and incorporating this into your scripts can be immensely valuable for decision-making and conditional execution.
Use Boolean Parameter to Pass Boolean Values to a PowerShell Script From a Command Prompt
To pass Boolean values to a PowerShell script from the command prompt using parameters, first, declare a parameter that will accept Boolean values. You can use the [bool]
data type to specify that the parameter should accept Boolean values.
For example:
param (
[bool]$MyBooleanParameter
)
Now that you have set up your parameter, you can employ the $MyBooleanParameter
variable in your script to make decisions based on the Boolean value.
For instance, consider the following example:
if ($MyBooleanParameter) {
Write-Host "The Boolean parameter is true."
}
else {
Write-Host "The Boolean parameter is false."
}
Now that you have defined the parameter in your PowerShell script, you can pass Boolean values to it from the command prompt when executing the script.
To do this, follow these steps:
-
Open the Command Prompt
Press Win+R, type
cmd
, and press Enter to open the Command Prompt. -
Navigate to the Script Directory
Use the
cd
command to go to the directory where your PowerShell script is located. For example:cd C:\Path\To\Your\Script
-
Execute the Script
To execute your PowerShell script and pass a Boolean value to the parameter, use the
PowerShell.exe
command. Provide the script file’s name and use the-MyBooleanParameter
parameter to pass the Boolean value.For example:
PowerShell.exe -File "YourScript.ps1" -MyBooleanParameter $true
In the above example, we are passing the value
$true
to the-MyBooleanParameter
parameter.To pass a
false
Boolean value to the parameter, also provide the script file’s name and use the-MyBooleanParameter
parameter with the$false
value.For example:
PowerShell.exe -File "YourScript.ps1" -MyBooleanParameter $false
In the above command, we’re passing the value
$false
to the-MyBooleanParameter
parameter, which means that the Boolean parameter within your script will receive afalse
value when executed. Your script will then act accordingly based on thisfalse
value.
Use the switch
Parameter to Pass Boolean Values to a PowerShell Script From a Command Prompt
The switch
parameters in PowerShell are a special type of parameter used for specifying Boolean options. They are used when a parameter is either present (true
) or absent (false
).
In other words, you don’t need to provide a value for a switch
parameter; its mere presence indicates a true
value, while its absence indicates a false
value.
To pass Boolean values to a PowerShell script from the command prompt using switch
parameters, first, declare a parameter as a switch
to accept Boolean values. You can use the [switch]
data type to specify that the parameter is a switch.
Here’s an example of how to define a switch
parameter:
param (
[switch]$MySwitchParameter
)
Then, once you’ve defined a switch
parameter, you can utilize the $MySwitchParameter
variable in your script to control its behavior based on whether the switch is present (true
) or absent (false
).
For example, you can use an if
statement to check the status of the switch
parameter:
if ($MySwitchParameter) {
Write-Host "The switch is present, so the Boolean value is true."
}
else {
Write-Host "The switch is absent, so the Boolean value is false."
}
With the switch
parameter defined in your script, you can easily pass Boolean values to it from the command prompt when running the script.
Here are the steps:
-
Open the Command Prompt
Launch the Command Prompt by pressing Win+R, typing
cmd
, and pressing Enter. -
Navigate to the Script Directory
Use the
cd
command to go to the directory where your PowerShell script is located. For example:cd C:\Path\To\Your\Script
-
Execute the Script
To execute your PowerShell script and pass a Boolean value using a
switch
parameter, use thePowerShell.exe
command. Provide the script file’s name and simply include the-MySwitchParameter
switch to enable it.For example:
PowerShell.exe -File "YourScript.ps1" -MySwitchParameter
In this example, the
switch
is present, indicating atrue
Boolean value.To pass a
false
Boolean value, simply run your script without including theswitch
parameter. Here’s an example:PowerShell.exe -File "YourScript.ps1"
By not including the
switch
parameter when executing the script, you are essentially passing afalse
Boolean value, and your script can take the appropriate action based on this absence.
Passing Boolean values to a PowerShell script from the command prompt using switch
parameters is an efficient and straightforward way to control the script’s behavior. The switch
parameters are ideal for scenarios where you want to enable or disable specific features or behaviors within your script without the need for additional arguments or values.
Use String Values to Pass Boolean Values to a PowerShell Script from the Command Prompt
When it comes to passing Boolean values to a PowerShell script from the command prompt, another approach is to use string values. This method allows for greater flexibility, as it lets you pass values such as true
or false
as strings and convert them into Boolean values within the script.
To pass Boolean values to a PowerShell script from the command prompt using string values, declare a parameter to accept string values that represent Boolean states. You can use the [string]
data type for this parameter.
For example:
param (
[string]$MyBooleanString
)
In your script, convert the string value to a Boolean value. PowerShell provides a way to perform this conversion.
For example:
$MyBoolean = [System.Management.Automation.LanguagePrimitives]::ConvertTo([bool], $MyBooleanString)
You can now use the $MyBoolean
variable in your script to work with the Boolean value as needed. For example:
if ($MyBoolean) {
Write-Host "The Boolean value is true."
}
else {
Write-Host "The Boolean value is false."
}
With the parameter for string values defined in your script, you can pass Boolean values from the command prompt when executing the script. Here’s how:
-
Open the Command Prompt
Launch the Command Prompt by pressing Win+R, typing
cmd
, and pressing Enter. -
Navigate to the Script Directory
Use the
cd
command to go to the directory where your PowerShell script is located. For example:cd C:\Path\To\Your\Script
-
Execute the Script
To run your PowerShell script and pass a string value representing a Boolean state, use the
PowerShell.exe
command. Provide the script file’s name and include the-MyBooleanString
parameter with a string value, such as"true"
or"false"
:PowerShell.exe -File "YourScript.ps1" -MyBooleanString "true"
Conclusion
Passing Boolean values to a PowerShell script from the command prompt using string values is a flexible and effective method, especially when dealing with user inputs or configuration files that may contain string representations of Boolean states. By converting these string values to Boolean within the script, you can seamlessly work with them and create scripts that are more adaptable and user-friendly.
Understanding how to convert string values to Boolean and use them in your PowerShell scripts is a valuable skill for script developers, as it allows for greater versatility and ease of use in a wide range of scenarios.