Boolean Values in PowerShell
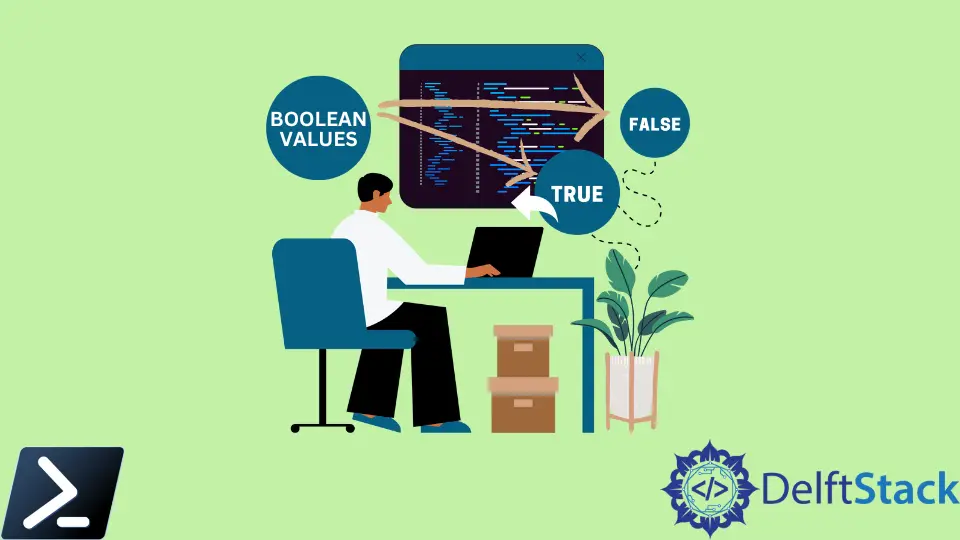
In Windows PowerShell scripts, we often use logic based on something true.
But there may be situations where you need to handle the inverse. That is a situation where you need to know when something does not meet some criteria.
So, while writing and debugging, PowerShell takes a more positive approach. It is essential to understand negation.
In this article, we will explore the usage of boolean values in PowerShell through native commands and operators, demonstrating their versatility and significance in script development.
Definition of Boolean in Windows PowerShell
When deciding if something is or isn’t in PowerShell, we talk about Boolean values represented as $True
or $False
.
The basic syntax shown below explains how Boolean works. Boolean type values are forms of output that return either True
or False
.
Still, the syntax uses comparison and conditional operators to compare the two or multiple values.
Example Code:
# Using -eq operator
"yes" -eq "yes"
# Using -ne operator
"no" -ne "no"
In the provided code, we utilize the -eq
operator to ascertain whether the string "yes"
matches "yes"
. Given that both strings are identical, this comparison yields $true
.
Conversely, employing the -ne
operator, we examine whether the string "no"
differs from "no"
. However, since both strings are identical, the comparison returns $false
.
Output:
When evaluating the Boolean expression, it compares the left side of the value to the right side of the value. If the value of the left side is equal to the value of the right side, then it is evaluated as True
; otherwise, False
, as shown above.
While -eq
and -ne
are valuable for comparing individual values, we can achieve more complex evaluations by using the and
and or
operators.
In PowerShell, the and
and or
operators are used to combine multiple conditions and evaluate them together.
Example Code:
$condition1 = $true
$condition2 = $false
# Using 'and' operator
$result1 = $condition1 -and $condition2
# Using 'or' operator
$result2 = $condition1 -or $condition2
Write-Host "Result of condition1 AND condition2: $result1"
Write-Host "Result of condition1 OR condition2: $result2"
In the provided code, we first set $condition1
to $true
and $condition2
to $false
. We then use the and
operator to combine these conditions.
Since $condition1
is true and $condition2
is false, the result of $result1
will be $false
. Next, we use the or
operator to combine the same conditions.
Since at least one condition ($condition1
) is true, the result of $result2
will be $true
.
Output:
There are multiple ways to output a Boolean value, and we will discuss them in the next section of the article.
Using Comparison Operators
We can use multiple conditional operators to compare values and output a Boolean result as our first example.
Example Code:
10 -eq 10 # equal
10 -gt 20 # greater than
10 -lt 20 # less than
10 -le 11 # less than or equal
10 -ge 8 # greater than or equal
In this script, we utilize different comparison operators to evaluate the relationship between distinct values. The -eq
operator assesses whether 10
equals 10
, which is true and thus results in $true
.
However, the -gt
operator scrutinizes whether 10
is greater than 20
, which is false and therefore yields false
. Similarly, -lt
, -le
, and -ge
operators are employed to examine if 10
is less than, less than or equal to, and greater than or equal to certain values, respectively, providing insightful comparisons between the values.
Output:
Using PowerShell Commands
Some native Windows PowerShell commands return Boolean values. One example of this is the Test-Path
cmdlet.
The Test-Path
cmdlet checks if the directory path exists inside our local machine.
Example Code:
# Check if the directory C:\Windows\temp exists
$exists = Test-Path -Path "C:\Windows\temp"
# Print the result
Write-Host "Directory exists: $exists"
In this script, we use the Test-Path
command to check if the directory C:\Windows\temp
exists on the system. The result of the Test-Path
command is stored in the variable $exists
, which will be $true
if the directory exists and $false
if it does not.
Output:
Some native commands will require a parameter to output a Boolean value. For example, the Test-Connection
command uses the -Quiet
parameter to return a Boolean value.
Example Code:
# Check if we can reach www.google.com with 2 echo request packets and suppress output
$reachable = Test-Connection -ComputerName "www.google.com" -Count 2 -Quiet
# Print the result
Write-Host "Host is reachable: $reachable"
In this script, we utilize the Test-Connection
command to send two echo request packets to www.google.com
and determine if the host is reachable. By specifying the -Quiet
parameter, we suppress all output except for the final result, which is stored in the variable $reachable
.
If www.google.com
is reachable, the value of $reachable
will be true
; otherwise, it will be false
.
Output:
Conclusion
Boolean values are fundamental in PowerShell scripting, enabling the evaluation of conditions and the control of program flow. While $True
and $False
are native boolean representations in PowerShell, alternatives such as 1
and 0
can also be employed in certain scenarios, particularly when interacting with external systems or APIs.
The use of comparison operators like -eq
and -ne
facilitates direct comparisons between values, returning boolean outcomes. Moreover, PowerShell commands like Test-Path
and Test-Connection
further expand the utility of boolean values, providing methods to assess the existence of directories or the reachability of hosts.
Understanding and effectively utilizing boolean values in PowerShell enhances script functionality and efficiency, empowering users to create robust and reliable automation solutions.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn