How to Use HTML Image Tag Inside PHP
- Use the Image Tag Inside a PHP Variable
- Store Image URL Source in PHP
-
Convert URL Strings With
str_replace()
and Usingiframe
Tag in PHP
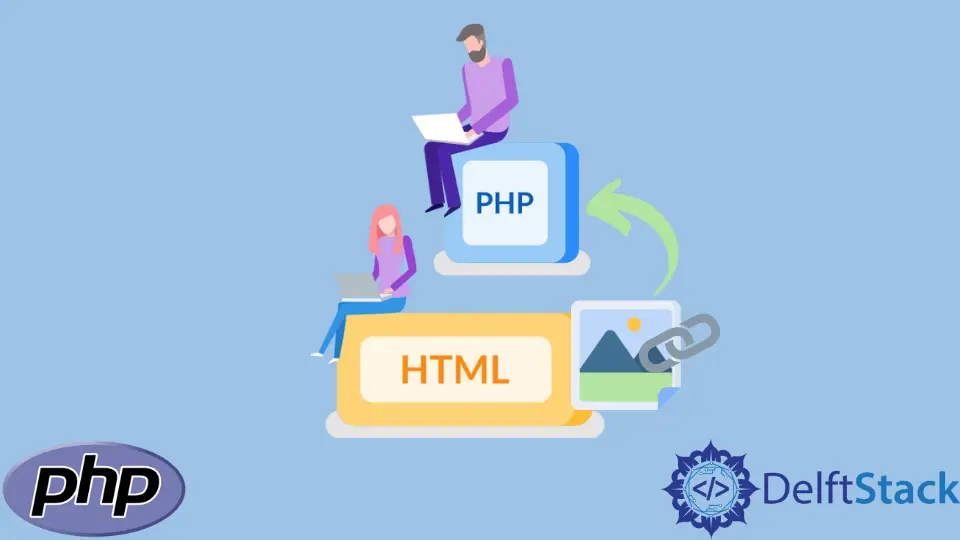
Using HTML tags inside PHP ensures the proper use of quotations. You only need to store your markup inside a PHP variable.
The HTML must remain within the quotations of the PHP strings whenever we substitute the PHP variable with the HTML tags. Hence, the actual quotes for your img
tag will not imply.
Single Quotes:
<?php
$demo = $demo = . '<img src= "\Your Source\" alt="" />';
?>
Double Quotes:
<?php
$demo2 = $demo2 . "<img src=\Your Image Source\" />";
?>
Now let’s have examples of how to use HTML tags inside PHP.
Use the Image Tag Inside a PHP Variable
HTML:
<!DOCTYPE html>
<head>
<title> Use Img Tag inside PHP Script </title>
<style>
img {
height: 400px;
width: 400px;
display: block;
margin-left: auto;
margin-right: auto
}
</style>
</head>
<body align='center'>
<form action='index.php' method='post'>
<input type='submit' name='image' value='Use HTML Image Tag inside PHP' />
<input type='submit' name='video' value='Use HTML Video Tag inside PHP' />
<input type='submit' name='method2' value='Use HTML Img Tag in PHP method 2' /> </form>
</body>
</html>
PHP script:
## Note: You do not need to reuse the HTML code above again. It contains markup for all three examples. (Also available in the code directory)
<?php
if(isset($_POST['image'])){
$use_img_tag_php = $use_img_tag_php . '<div>
<img src="demoimage.gif" alt="" /></div>';
echo $use_img_tag_php;
}
?>
Output:
We have used single quotes for the PHP and double for the HTML. With this method, there is no need to echo your variable inside the source of the image tag anymore.
Store Image URL Source in PHP
You only need to store your URLs in a PHP variable and echo the source inside the src
attribute of the img
tag.
Code:
<?php
if(isset($_POST['method2'])){
//Asign URL path to a variable
$imgurl = "demoimage.gif"; //Use image URL from your root directory
//Now it dynamic
//Close php tag
?>
<div>
<img src="<?php
echo $imgurl ?>" alt="" /></div>
<?php
}
//close php tag, the isset condition will only load HTML inside its body
?>
Output:
Convert URL Strings With str_replace()
and Using iframe
Tag in PHP
If you have copied any youtube URL and you want to use this in an HTML iframe
. At the same time, you want it to be dynamic.
We will put HTML tag inside PHP while also using str_replace()
.
Code:
<?php
if(isset($_POST['video'])){
$fetchyoutubevideo = "https://www.youtube.com/watch?v=/AkFi90lZmXA";
$embed_it = str_replace("watch?v=", "embed/", $fetchyoutubevideo);
echo $embed_it;
echo $use_video_tag_php = $use_video_tag_php.'<div>
<iframe width="560" height="315"
src="'.$embed_it.'" title="Video Title" frameborder="0" allow="accelerometer;
autoplay; clipboard-write;
encrypted-media; gyroscope; picture-in-picture" allowfullscreen >
</iframe></div>';
}
?>
Output:
In the above example, we stored the URL path into $fetchyoutubevideo
. Then we use str_replace();
and pass three parameters.
- First parameter - (
watch?v
) = string - Second parameter - (
/embed
) = string - Third parameter - (
$embed_it
) = PHP variable (URL String)
The str_replace
function has replaced the first parameter with the second and stored it in the third parameter.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn