How to Minify HTML Output of the PHP Page
- Minify HTML Output of the PHP Page
- Use GZip Compression in Apache to Minify HTML Output of the PHP Page
-
Use the
ob_start()
Function With a Callback to Minify HTML Output of the PHP Page - Use a HTML Minifier Plugin to Minify HTML Output of the PHP Page
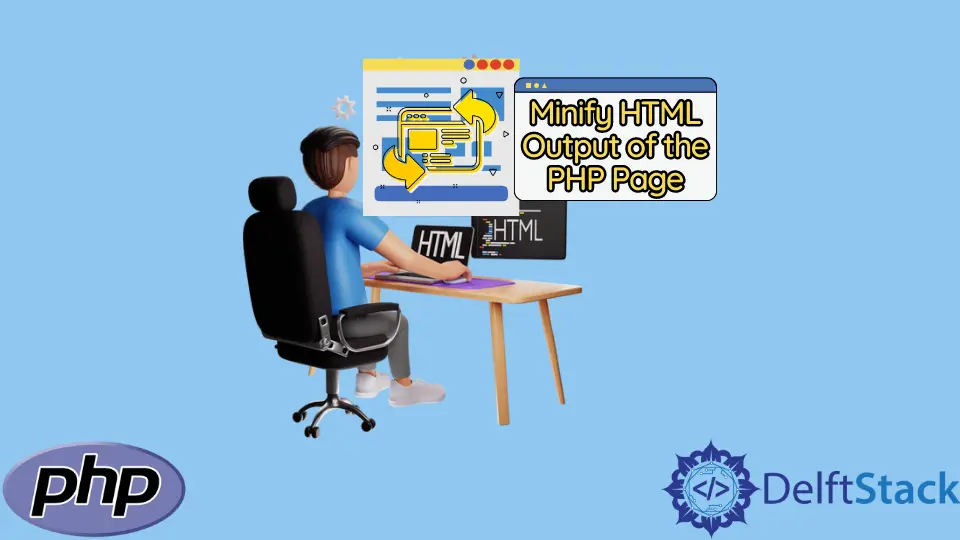
This article will discuss minifying your HTML output for PHP pages.
Minify HTML Output of the PHP Page
We minify the output to improve the overall website performance and user experience. The process involves removing unnecessary files for website users and reducing the page size and load times for website pages.
Additionally, website users can minimize resource or data usage through minified pages. The minifying process eliminates unnecessary details, newlines, comments, and excessive whitespaces.
The downside of the process is reducing code readability. When minifying, you can reduce your file size by 70%.
You can minify HTML output manually or automatically.
There are several tools available that can restore whitespaces in your code. However, you cannot revert changes done to the comments present on your script. Let us look at an example.
<html>
<head>
<!-- This content will show on the browser -->
<title>Title Page</title>
</head>
<body>
<!-- This is a comment. -->
<h1>Delftstack Tutorials!</h1>
</body>
</html>
The above file has a lot of whitespaces, newlines, and two comments. If we were to minify the file, it would look like this.
<html><head><title>Title Page</title></head><body><h1>Delftstack Tutorials!</h1></body></html>
Let us look at the different methods to minify your HTML output for PHP pages.
Use GZip Compression in Apache to Minify HTML Output of the PHP Page
You can minify your output by enabling GZip compression in Apache. Follow these steps below.
-
Locate and open your Apache configuration file. You can use Notepad to make the edits below.
Directories may defer depending on your system but make sure you open the
httpd.conf
file.
vim /etc/httpd/conf/httpd.conf
-
In the configuration file, check the line below by adding a
#
.LoadModule deflate_module modules/mod_deflate.so
-
Copy and paste the lines below to the end of the configuration file.
AddOutputFilterByType DEFLATE text/plain AddOutputFilterByType DEFLATE text/html AddOutputFilterByType DEFLATE text/xml AddOutputFilterByType DEFLATE text/css AddOutputFilterByType DEFLATE application/xml AddOutputFilterByType DEFLATE application/xhtml+xml AddOutputFilterByType DEFLATE application/rss+xml AddOutputFilterByType DEFLATE application/javascript AddOutputFilterByType DEFLATE application/x-javascript
-
Restart your Apache server.
sudo service httpd restart
Use the ob_start()
Function With a Callback to Minify HTML Output of the PHP Page
You can use the ob_start()
function with a callback to remove whitespaces before and after the tags, comments, and whitespace sequences.
Let us look at an example code.
<?php
ob_start("minifier");
function minifier($code) {
$search = array(
// Remove whitespaces after tags
'/\>[^\S ]+/s',
// Remove whitespaces before tags
'/[^\S ]+\</s',
// Remove multiple whitespace sequences
'/(\s)+/s',
// Removes comments
'/<!--(.|\s)*?-->/'
);
$replace = array('>', '<', '\\1');
$code = preg_replace($search, $replace, $code);
return $code;
}
?>
<!DOCTYPE html>
<html>
<head>
<!-- Page Title -->
<title>Sample Minifier</title>
</head>
<body>
<!-- page body -->
<h1>Delftstack Tutorials!</h1>
</body>
</html>
<?php
ob_end_flush();
?>
After running the code above, we got the output below.
<!DOCTYPE html><html><head><title>Sample minifier</title></head><body><h1>Delftstack Tutorials!</h1></body></html>
Use a HTML Minifier Plugin to Minify HTML Output of the PHP Page
We use an HTML Minifier on the server-side as a source code to optimize the output on the client’s side. The plugin removes unnecessary whitespaces, newlines, and comments.
You can choose from a pool of optimization choices based on your needs. Follow these steps to set up your plugin.
-
Use the link below to download the HTML Minifier file on your computer.
https://www.terresquall.com/download/HTMLMinifier.php
-
Copy and paste the code below into your PHP file.
<?php // Import the HTMLMinifier require_once 'myfolder/HTMLMinifier.php'; // HTML source to be minified $htmlpage = file_get_contents('./mypage.html'); // Minified version of the page echo HTMLMinifier::process($htmlpage); ?>
-
Run your PHP file.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn