How to Remove Special Character in PHP
-
Use the
preg_replace()
Function to Remove Special Character in PHP -
Use the
str_replace()
Function to Remove Special Character in PHP -
Use the
trim()
Function to Remove Special Character in PHP -
Use the
htmlspecialchars()
andstr_ireplace()
Functions to Remove Special Character in PHP -
Use the
substr()
Function to Remove Special Character in PHP -
Use the
strtr()
Function to Remove Special Character in PHP
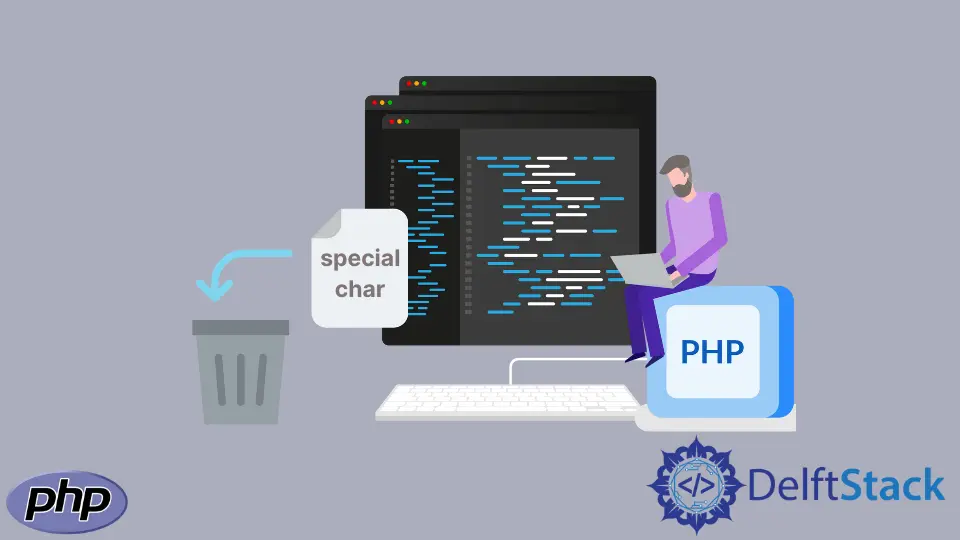
In programming, sometimes, we want to remove some special characters from the string. This tutorial shows how we will use different functions to remove the special character from a string.
Use the preg_replace()
Function to Remove Special Character in PHP
This function preg_replace()
contains few parameters as introduced below.
$pattern
: This parameter gives us a pattern for searching string or array of strings.$replaceWith
: In this, we give the character from which the$pattern
string or array is replaced. If the$pattern
parameter is an array and the$replaceWith
parameter is a string, then the all$pattern
parameter is replaced by the$replaceWith
string. On the other hand, if both$pattern
and$replaceWith
parameters are arrays, then it does the same as in the above condition but replace the specific indexed string of$pattern
with its responding counter index of$replaceWith
.$string
: This is the desirable string you want to filter with a special character.
See the example below.
<?php
function RemoveSpecialChar($str)
{
$res = preg_replace('/[0-9\@\.\;\" "]+/', '', $str);
return $res;
}
$str = "My name is hello and email hello.world598@gmail.com;";
$str1 = RemoveSpecialChar($str);
echo "My UpdatedString: ", $str1;
?>
Output:
My UpdatedString: Mynameishelloandemailhelloworldgmailcom
In this function, you can easily remove the special character, but before using this function, you need to havesome knowledge of regular expression.
Use the str_replace()
Function to Remove Special Character in PHP
This is also a very useful built-in function, which is used to replace the special character from the string. str_replace()
is also helps to replace that character with the removed character.
This function contains few parameters, as introduced below.
$search_str
: It contains such value which we want to search in the given string.$replace_str
: It stores a value that you want to replace, or you can also leave it empty if you only want the removal of a special character.$main_str
: It is the string we want to update.$count
: It represents the number of characters we want to replace or remove.
See the example code.
<?php
$mainstr = "This is a sim'ple text;";
echo "Text before remove: \n" . $mainstr, "\n";
$replacestr = remove_sp_chr($mainstr);
function remove_sp_chr($str)
{
$result = str_replace(array("#", "'", ";"), '', $str);
echo "\n\nText after remove: \n" . $result;
}
?>
Output:
Text before remove:
This is a sim'ple text;
Text after remove:
This is a simple text
Use the trim()
Function to Remove Special Character in PHP
This function only removes the character from the first and end of the string. It ignores the character who is in the middle of the string. If you want to remove only the starting and end of the character, then we use this function.
This function is easy to use and doesn’t take too many parameters. It demands only the main string from which you want to remove their first and last special character.
Here below we see how it works and what is its output:
<?php
$mainstr = "@@PHP@Programming!!!.";
echo "Text before remove:\n" . $mainstr;
echo "\n\nText after remove: \n" . trim($mainstr, '@!.');
?>
Output:
Text before remove:
@@PHP@Programming!!!.
Text after remove:
PHP@Programming
This function also has two variants.
ltrim()
rtrim()
the ltrim()
Function
It removes only the first characters of your string.
<?php
$str = "geeks";
$str = ltrim($str, 'g');
echo $str;
?>
Output:
eeks
the rtrim()
Function
It is the same as the above function, but it can remove your string’s last characters.
<?php
$string = "DelftStack is a best platform.....";
echo "Output: " . rtrim($string, ".");
?>
Output:
Output: DelftStack is a best platform
Use the htmlspecialchars()
and str_ireplace()
Functions to Remove Special Character in PHP
The htmlspecialchars()
and str_ireplace()
are used to remove the effect of predefined characters from the data. It converts all predefined elements of Html into special characters like it converts <
into <,&
then it will be converted into &
.
The str_ireplace()
is used for the removal of Html characters from the text. It works as str_replace()
, which is briefly explained above, and can perform case-insensitive searches. It means that when we scrap the code of Html in this function and after processing, it may remove all special characters of Html like <h2>
, <b>
e.t.c.
Lets see how it works:
<?php
$mainstr = "<h2>Welcome to <b>PHPWorld</b></h2>";
echo "Text before remove: \n" . $mainstr;
echo "\n\nText after remove: \n" .
str_ireplace(array('<b>', '</b>', '<h2>', '</h2>'), '',
htmlspecialchars($mainstr));
?>
Output:
Text before remove:
<h2>Welcome to <b>PHPWorld</b></h2>
Text after remove:
Welcome to PHPWorld
Use the substr()
Function to Remove Special Character in PHP
As we know, the string is a kind of array. The substr()
function removes up to the specific index of character.
<?php
$str = "@@HelloWorld";
$str1 = substr($str, 1);
echo $str1 . "\n\n";
$str1 = substr($str, 2);
echo $str1;
?>
Output:
@HelloWorld
HelloWorld
Use the strtr()
Function to Remove Special Character in PHP
This function is an amazing feature of PHP. It translates characters or replaces substrings. It takes three parameters, the first one is the string on which we apply this function after that the second parameter is that character which we want to replace from that string and the last parameter is that character from which we want to replace the value of the second parameter. Now we see through programming:
<?php
$str = "ei all, I said eello";
//$trans = array("h" => "-", "hello" => "hi", "hi" => "hello");
echo "Output: " . strtr($str, "e", "h");
?>
Output:
Output: hi all, I said hhllo
There is its good feature where str_replace()
become fail. Below is its example:
<?php
$strTemplate = "My name is :name, not :name2.";
$strParams = [
':name' => 'Dave',
'Dave' => ':name2 or :password',
':name2' => 'Steve',
':pass' => '7hf2348', ];
echo "\n" . strtr($strTemplate, $strParams) . "\n";
echo "\n" . str_replace(array_keys($strParams), array_values($strParams), $strTemplate) . "\n";
?>
Output:
My name is Dave, not Steve.
My name is Steve or 7hf2348word, not Steve or 7hf2348word2.