How to Validate Email in PHP
-
Use the
filter_var()
Function and theFILTER_VALIDATE_EMAIL
to Validate the Email in PHP -
Use the
FILTER_VALIDATE_EMAIL
,FILTER_SANITIZE_EMAIL
andfilter_var()
Functions to Validate the Email in PHP -
Use the
preg_match()
Function to Validate the Email According to the Regular Expression
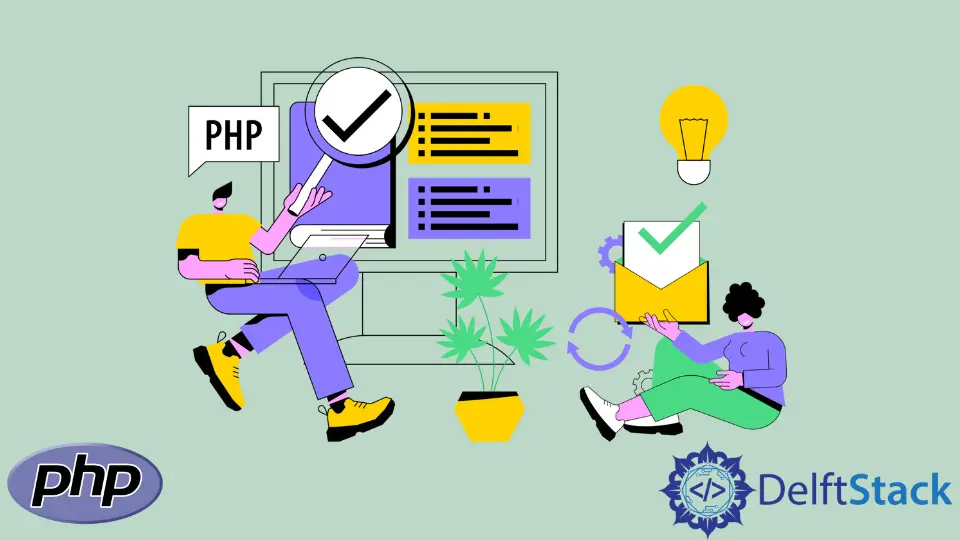
We will introduce a method to validate an email address in PHP using the filter_var()
function and the FILTER_VALIDATE_EMAIL
filter name id. The filter_var()
function takes the email as the first argument and the filter name FILTER_VALIDATE_EMAIL
to validate the email against the syntax in RFC 822. This method checks the valid email format rather than the valid email.
We will also demonstrate another method to validate email addresses in PHP using the FILTER_SANITIZE_EMAIL
and FILTER_VALIDATE_EMAIL
filter name ids along with the fiter_var()
function. This method first sanitizes the email address and then validates the email address.
We will introduce another method to validate email in PHP using the regular expression. This method uses the preg_match()
function to check if the email is valid according to the provided regular expression.
Use the filter_var()
Function and the FILTER_VALIDATE_EMAIL
to Validate the Email in PHP
We can use the filter_var()
function to filter a variable with a particular filter name. The FILTER_VALIDATE_EMAIL
filter name specifies that the email needs to verify. The function takes the email address as a string as the first parameter and the above-specified filter id as the second parameter. Thus, we can check the email provided whether is valid. The function returns the filtered data if the function succeeds or returns false. The email is said valid, not in the sense that the email exists. The filter id validates the email against the syntax in RFC 822. We can test the validation of email using a valid and an invalid email.
For example, create a function validateEmail()
that takes a parameter $email
. Use the filter_var()
function on the $email
variable and specify the filter id FILTER_VALIDATE_EMAIL
as the second parameter. Apply the if-else
condition on the filter_var()
function. In the if
block, display the message saying the email is valid, and in the else
condition, display that email is invalid. Outside the function, call the function two times. In the first function call supply the argument, peter.piper@iana.org
and first.last@example.123
in the second call.
We can assume that the email address provided in the example is accessed from a form using the $_POST
variable. The function in the example below gets invoked two times. The first invocation passes a valid email address and the second address passes an invalid email. The second email address is invalid as it contains numbers in the top-level domain. The result is as obvious.
Example Code:
#php 7.x
<?php
function validateEmail($email) {
if(filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "{$email}: A valid email"."<br>";
}
else {
echo "{$email}: Not a valid email"."<br>";
}
}
validateEmail('peter.piper@iana.org');
validateEmail('first.last@example.123');
?>
Output:
phppeter.piper@iana.org: A valid email
first.last@example.123:Not a valid email
Use the FILTER_VALIDATE_EMAIL
, FILTER_SANITIZE_EMAIL
and filter_var()
Functions to Validate the Email in PHP
We can use the additional FILTER_SANITIZE_EMAIL
filter name id in the first method to remove all the illegal characters from an email address. The filter name id is the second argument in the filter_var()
function, where the email address is the first argument. The function returns the sanitized email. We can again use the function to check for the validity of the email address after the sanitization. For this, we can follow the first method using the FILTER_VALIDATE_EMAIL
filter name id.
For example, create a variable $email
and store an email address with illegal characters in it. Store the email ram(.mugu)@exa//mple.org
as a string in the variable. Use the filter_var()
function on the variable and use FILTER_SANITIZE_EMAIL
id as the second parameter. Store the function on the same $email
variable. Then, apply the if-else
statement as in the first method. This time, use the FILTER_VALIDATE_EMAIL
email as the filter name in the function. Similarly, display the messages.
An email address with an illegal character is taken in the example below, and the filter_var()
function filters those characters and sanitizes the provided email. The email address provided in the example contains illegal characters such as ()
and //
. The function first removes these characters from the email and then validates the email.
Example Code:
#php 7.x
<?php
$email = "ram(.mugu)@exa//mple.org";
$email = filter_var($email, FILTER_SANITIZE_EMAIL);
if(filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "{$email}: A valid email"."<br>";
}
else{
echo "{$email}:Not a valid email"."<br>";
}
?>
Output:
ram.mugu@example.org: A valid email
Use the preg_match()
Function to Validate the Email According to the Regular Expression
We can use the preg_match()
function to validate the email address in PHP. This method uses the regular expression for the validation rule of the email. We can create a regular expression ourselves and define the rules for a valid email. The preg_match()
function takes two parameters where the first one is the regular expression, and the second one is the email to be checked. We can use the ternary operator to check the validity of the email along with the function.
For example, create two variables, $email_first
and $email_secon
, and store two email addresses in those variables. Store a valid email firstlast11@gmail.com
first and store an invalid one firstlast@11gmail,com
in the second. Write a function validateEmail()
that takes one parameter. Name the parameter $email
. Inside the function, write a regular expression in the $regex
variable as in the example code. Then write a ternary operator where the condition to be checked is the preg_match()
function. Take the $regex
as the first parameter and the $email
as the second parameter. Print the message saying the email is valid when the condition is true and the message saying the email is invalid when the condition is false. Echo the whole ternary expression. Outside the function, call the validateEmail()
function two times. Use the $email_first
variable in the first function call and use the $email_second
variable in the second function call.
In the example below, we have written a regular expression that creates a rule for validating the email. A valid email contains a recipient name, @
symbol, a domain, and a top-level domain. The above-created regex expression accepts the recipient name as alphanumeric values. The alphabet consists of both the uppercase and the lowercase. It also accepts a period. The email must have the @
symbol. The domain contains the alphabets only. The email should have a period then. The top-level domain should only consist of the alphabets and should have a length of two or three. The regex expression is created based on this rule. The first email is valid since it satisfies all the rules, but the second email is invalid. It is invalid because there is a number in the domain name, and there is no period before the top-level domain.
Example Code:
# php 7.x
<?php
$email_first = 'firstlast11@gmail.com';
$email_second ='firstlast@11gmail,com';
function validateEmail($email) {
$regex = "/^([a-zA-Z0-9\.]+@+[a-zA-Z]+(\.)+[a-zA-Z]{2,3})$/";
echo preg_match($regex, $email) ? "The email is valid"."<br>" :"The email is not valid";
}
validateEmail($email_first);
validateEmail($email_second);
?>
Output:
The email is valid
The email is not valid
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn