How to Round Floating-Point Numbers in PHP
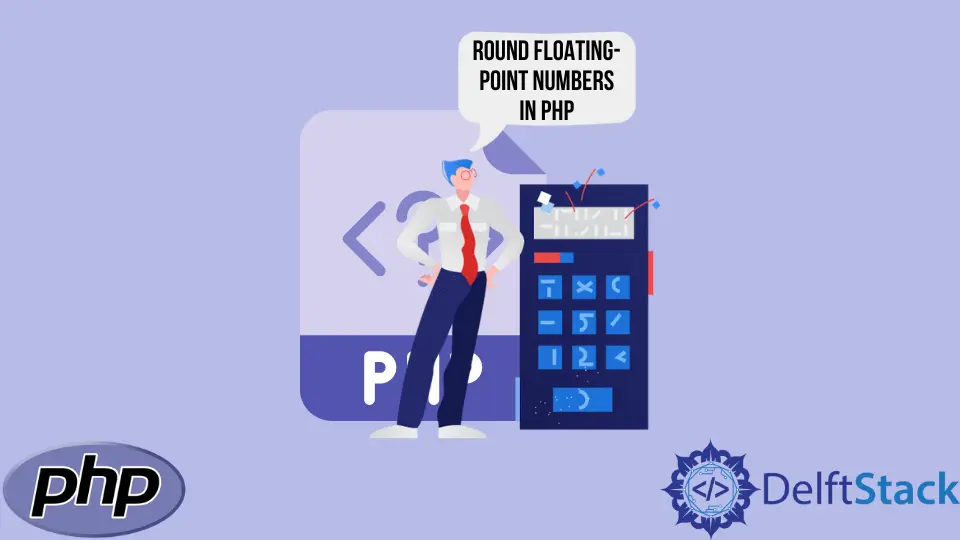
In PHP, the round()
function rounds the floating-point numbers.
This tutorial demonstrates how to use PHP’s round()
function.
Round the Floating-Point Numbers in PHP
The round()
function rounds a floating-point number in PHP. We can use it to define a specific precision value that will round the numbers, keeping the precision value in view.
This precision value can be zero or negative. The function has three parameters; the syntax for this function is:
float round(number, precision, mode);
Where:
-
The
number
is the floating-point number we want to round. -
The
precision
is an optional parameter that decides the number of decimal digits to round to. The defaultprecision
value is zero. -
The
mode
is an optional parameter to specify a constant, specifying the rounding mode. There are four types of constants for mode:3.1.
PHP_ROUND_HALF_UP
: This mode will tell the method to round the number away from zero.
3.2.PHP_ROUND_HALF_DOWN
: This mode will tell the method to round the number towards zero.
3.3.PHP_ROUND_HALF_EVEN
: This mode will tell the method to round the number towards the nearest even value.
3.4.PHP_ROUND_HALF_ODD
: This mode will tell the method to round the number towards the nearest odd value.
The return value for this method is the rounded number. Let’s try an example of this method.
<?php
$RoundValue1=round(10.3);
$RoundValue2=round(12.5);
$RoundValue3=round(13.6);
$RoundValue4=round(14.6,0);
$RoundValue5=round(6.97553,2);
$RoundValue6=round(7.76521,-3);
$RoundValue7=round(8.063,2);
$RoundValue8=round(9.076,2);
$RoundValue9=round(6.97553, PHP_ROUND_HALF_UP);
$RoundValue10=round(6.97553, PHP_ROUND_HALF_DOWN);
$RoundValue11=round(6.97553, PHP_ROUND_HALF_EVEN);
$RoundValue12=round(6.97553, PHP_ROUND_HALF_ODD) ;
echo "The Round Value for (10.3) is : ". $RoundValue1 . "<br /><br />" ;
echo "The Round Value for (12.5) is : ". $RoundValue2 . "<br /><br />" ;
echo "The Round Value for (13,6) is : ". $RoundValue3 . "<br /><br />" ;
echo "The Round Value for (14.6,0) is : ". $RoundValue4 . "<br /><br />" ;
echo "The Round Value for (6.97553,2) is : ". $RoundValue5 . "<br /><br />" ;
echo "The Round Value for (7.76521,-3) is : ". $RoundValue6 . "<br /><br />" ;
echo "The Round Value for (8.063,2) is : ". $RoundValue7 . "<br /><br />" ;
echo "The Round Value for (9.076,2) is : ". $RoundValue8 . "<br /><br />" ;
echo "The Round Value for (6.97553,2, PHP_ROUND_HALF_UP) is : ". $RoundValue9 . "<br /><br />" ;
echo "The Round Value for (6.97553,2, PHP_ROUND_HALF_DOWN) is : ". $RoundValue10 . "<br /><br />" ;
echo "The Round Value for (6.97553,2, PHP_ROUND_HALF_EVEN) is : ". $RoundValue11 . "<br /><br />" ;
echo "The Round Value for (6.97553,2, PHP_ROUND_HALF_ODD) is : ". $RoundValue12 . "<br /><br />" ;
?>
The code above shows 12 different versions of the usage of the round()
method. See the output:
The Round Value for (10.3) is : 10
The Round Value for (12.5) is : 13
The Round Value for (13,6) is : 14
The Round Value for (14.6,0) is : 15
The Round Value for (6.97553,2) is : 6.98
The Round Value for (7.76521,-3) is : 0
The Round Value for (8.063,2) is : 8.06
The Round Value for (9.076,2) is : 9.08
The Round Value for (6.97553,2, PHP_ROUND_HALF_UP) is : 7
The Round Value for (6.97553,2, PHP_ROUND_HALF_DOWN) is : 6.98
The Round Value for (6.97553,2, PHP_ROUND_HALF_EVEN) is : 6.976
The Round Value for (6.97553,2, PHP_ROUND_HALF_ODD) is : 6.9755
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook