How to Show a Number to Two Decimal Places in PHP
-
Use
number_format()
Function to Show a Number to Two Decimal Places in PHP -
Use
round()
Function to Show a Number to Two Decimal Places in PHP -
Use
sprintf()
Function to Show a Number to Two Decimal Places in PHP
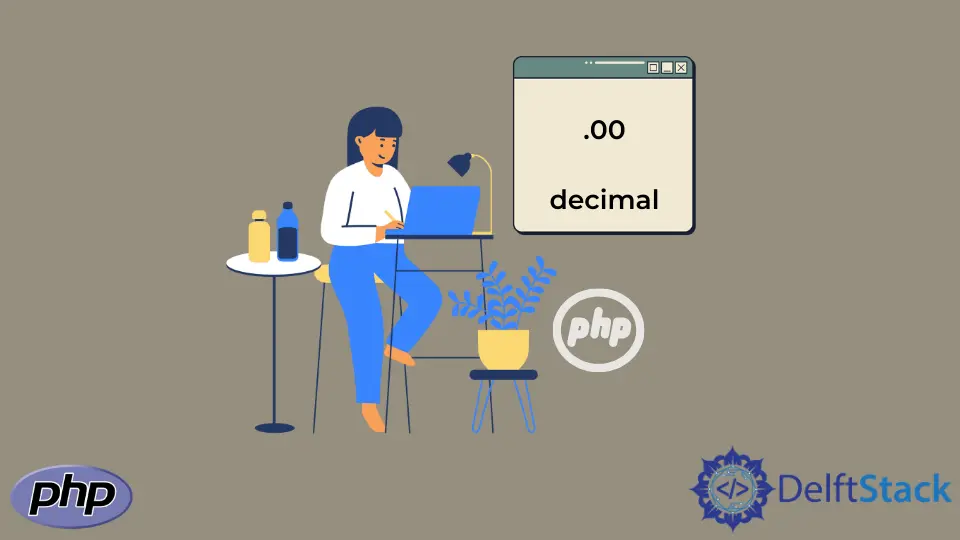
In this article, we will introduce methods to show a number
to two decimal places in PHP.
- Using
number_format()
function - Using
round()
function - Using
sprintf()
function
Use number_format()
Function to Show a Number to Two Decimal Places in PHP
The built-in function number_format()
is used to format a number. By formatting, we mean that the number is displayed with a decimal point and a thousand separator. It also rounds a number if needed. We can use this function to show a number to two decimal places. The correct syntax to use this function is as follows
number_format($number, $NumOfDecimals, $decimalIndicator, $thousandSeparator)
Parmater | Description | |
---|---|---|
$number |
mandatory | the number to be formatted |
$NumOfDecimals |
optional | the number of decimal values after decimal point |
$decimalIndicator |
optional | a custom decimal point for the number. If omitted, the decimal point by default is . |
$thousandSeparator |
optional | a custom thousand separator. If omitted, the thousand separator by default is "," |
Example Codes:
<?php
$number1 = 5;
$number2 = 34.600;
$number3 = 15439.093;
$format_number1 = number_format($number1, 2);
$format_number2 = number_format($number2, 2);
$format_number3 = number_format($number3, 2, "d", "@");
echo "The number $number1 after formating is: $format_number1 \n";
echo "The number $number2 after formating is: $format_number2 \n";
echo "The number $number3 after formating is: $format_number3 \n";
?>
This function has three optional parameters but it does not allow three parameters. It allows one, two, and four parameters to be passed. In this example, the $number1
and $number2
are formatted to two decimal places with the default decimal point. But $number3
is formatted with custom decimal point "d"
and thousand separator "@"
.
Output:
The number 5 after formating is: 5.00
The number 34.6 after formating is: 34.60
The number 15439.093 after formating is: 15@439d09
Use round()
Function to Show a Number to Two Decimal Places in PHP
The round()
function is used to round a number or float value. We can round a number to our desired decimal places. The correct syntax to use this function is as follows
round($number, $decimalPlaces, $mode);
Parmater | Description | |
---|---|---|
$number |
mandatory | the number to be formatted |
$decimalPlaces |
optional | the number of decimal values after decimal point |
$mode |
optional | rounding mode |
Example Codes:
<?php
$number1 = 5;
$number2 = 34.6;
$number3 = 15439.093;
$format_number1 = round($number1, 2);
$format_number2 = round($number2, 2);
$format_number3 = round($number3, 2);
echo "The number $number1 after rounding is: $format_number1 \n";
echo "The number $number2 after rounding is: $format_number2 \n";
echo "The number $number3 after rounding is: $format_number3 \n";
?>
The important thing to note here is that it does not affect a number or a float value with one decimal point if we want to round these to two decimal places.
Output:
The number 5 after rounding is: 5
The number 34.6 after rounding is: 34.6
The number 15439.093 after rounding is: 15439.09
When the original number has fewer decimal digits than the decimal places to be formatted, round()
function will not add zeros at the end of the number.
You should use the number_format()
method if two decimal places are needed for all numbers including integers.
Use sprintf()
Function to Show a Number to Two Decimal Places in PHP
The built-in function sprintf()
formats a string according to a given format. It can be used to show a number to two decimal places. The correct syntax to use this function is as follows
sprintf($format, $parameter1, $parameter2, ... , $parameterN);
The parameter $format
is the format that specifies how the variables will be in the string. The next parameter $parameter1
is the first variable whose value will be assigned to the first %
in the string. The parameter $parameter2
is the second variable whose value will be assigned to the second %
in the string. In this way, we can insert N variables for N %
signs.
<?php
$num = 67;
$number = sprintf('%.2f', $num);
echo "The number upto two decimal places is $number";
?>
Here, we have used %f
for a float value. %.2f
indicates that the float value will be up to two decimal places.
Output:
The number up to two decimal places is 67.00