Syntax to Check for Not Null and an Empty String in PHP
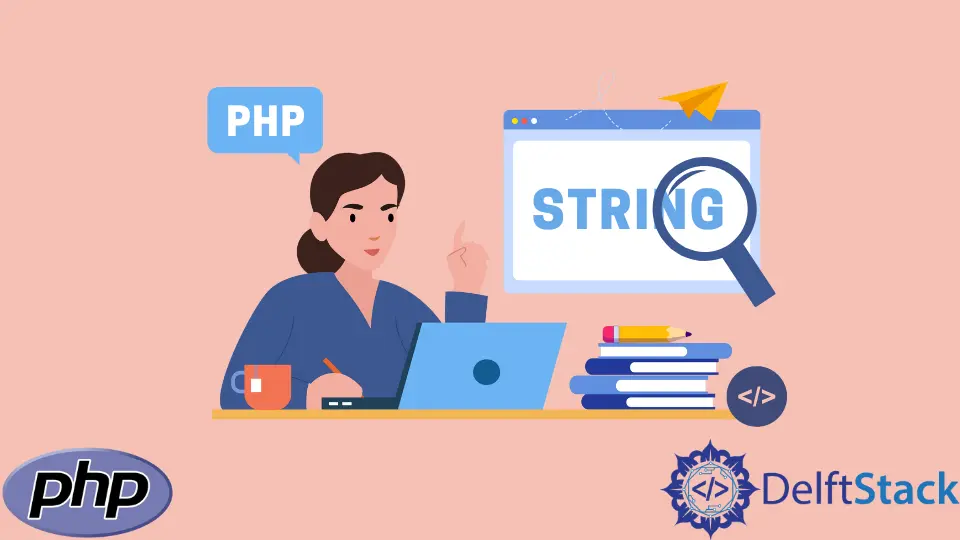
This article teaches you how to check for not null and empty strings in PHP. We’ll use PHP empty()
and is_null()
functions along with the negation operator.
Check for Not Null Using is_null()
in PHP
PHP is_null
function will check whether a variable is null or not. Meanwhile, you can append it with the negation operator, and it’ll check if the variable is not null.
In PHP, the negation operator is the exclamation mark (!
). We present an example below where we check whether a string is not null.
<?php
// Define a simple string
$sample_string = "I am a string";
// Check if it's not null. We use PHP is_null
// function, but we've added the negation
// sign before it.
if (!is_null($sample_string)) {
echo "Your variable <b>" . $sample_string . "</b> is not null.";
} else {
echo "Your variable is null.";
}
?>
Output:
Your variable <b>I am a string</b> is not null.
Check for an Empty String Using empty()
in PHP
PHP empty()
function allows you to check for an empty string. Also, the empty()
function can check for other values that PHP evaluates as empty.
In the following example, we use the empty()
function to test for an empty string among other values.
<?php
$empty_string = "";
$integer_zero = 0;
$decimal_zero = 0.0;
$string_zero = "0";
$null_keyword = NULL;
$boolean_false = FALSE;
$array_with_no_data = [];
$uninitialized_variable;
if (empty($empty_string)) {
echo "This message means the argument to function empty() was an empty string. <br />";
}
if (empty($integer_zero)) {
echo $integer_zero . " is empty. <br />";
}
if (empty($decimal_zero)) {
echo number_format($decimal_zero, 1) . " is empty. <br />";
}
if (empty($string_zero)) {
echo $string_zero . " as a string is empty. <br />";
}
if (empty($null_keyword)) {
echo "NULL is empty. <br />";
}
if (empty($boolean_false)) {
echo"FALSE is empty. <br />";
}
if (empty($array_with_no_data)) {
echo "Your array is empty. <br />";
}
if (empty($uninitialized_variable)) {
echo "Yes, your uninitialized variable is empty.";
}
?>
Output:
This message means the argument to function empty() was an empty string. <br />
0 is empty. <br />
0.0 is empty. <br />
0 as a string is empty. <br />
NULL is empty. <br />
FALSE is empty. <br />
Your array is empty. <br />
Yes, your uninitialized variable is empty.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn