How to Check Type and Value of Null in PHP
- Compare Null Value With Empty String Using Double and Triple Equals Operator in PHP
-
Compare Null Value With
0
Using Double and Triple Equals Operator in PHP -
Compare Null Value With
false
Using Double and Triple Equals Operator in PHP
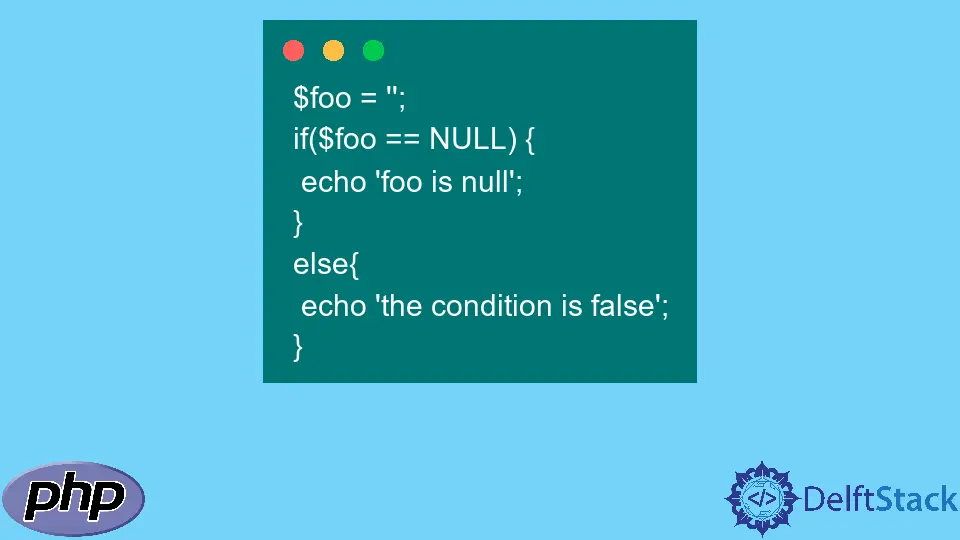
This article will compare and check the PHP null value with values like ''
, 0
, and false
. We will use the double and triple equals operator to demonstrate the differences.
Compare Null Value With Empty String Using Double and Triple Equals Operator in PHP
In PHP, there are various comparison operators. We use the comparison operators to compare the value between two entities. The double and triple equals operators in PHP are most commonly used. There is a simple difference between these two comparison operators. The double equals operator, ==
compares the value between the two entities. Whereas the triple equals operator, ===
compares the value, as well as the type between the two entities. We need to know these differences to compare the entities in PHP the way we want.
We can compare a null value with an empty string and watch the differences using these, both comparison operators. For example, create a variable $foo
and assign it to an empty string. Then, compare the variable with NULL
with the double equals operator using the if
condition. Display the message foo is null
, if the condition is true. Display the message the condition is false
, if the condition is false. Similarly, use the triple equals operator for the same piece of code.
We can see that the first code example returns the true value, and the second returns the false value. The double equals operator compares only the value of empty string and NULL
, and the value are equal. But, the triple equals operator compares the value as well as the type of these entities. As the empty string is of string
type and NULL
is of NULL
type, the value returned is false. We can use the gettype()
function to check the type.
Example Code:
$foo = '';
if($foo == NULL) {
echo 'foo is null';
}
else{
echo 'the condition is false';
}
Output:
foo is null
Example Code:
$foo = '';
if($foo === NULL) {
echo 'foo is null';
}
else{
echo 'the condition is false';
}
Output:
the condition is false
Compare Null Value With 0
Using Double and Triple Equals Operator in PHP
Here, we will compare NULL
with 0
using the double equals and triple equals operator. We know that 0
is an integer. When we compare it with NULL
using the double equals operator, the condition will be true as 0
is null. But, integer
and NULL
are different types. Therefore, using the triple equals operator will run the false condition.
Thus, we learned how the double and triple equals comparison operators work while comparing the null value with 0
.
Example Code:
$foo = 0;
if($foo == NULL) {
echo 'foo is null';
}
else{
echo 'the condition is false';
}
Output:
foo is null
Example Code:
$foo = 0;
if($foo === NULL) {
echo 'foo is null';
}
else{
echo 'the condition is false';
}
Output:
the condition is false
Compare Null Value With false
Using Double and Triple Equals Operator in PHP
We will compare NULL
with the false
boolean value using the double and triple equals comparison operators. The value of false
and NULL
are the same; therefore, the true condition is executed using the double equals operator. As false
is a boolean value, its type is not the same as NULL
, so the false condition is executed.
Example Code:
$foo = false;
if($foo == NULL) {
echo 'foo is null';
}
else{
echo 'the condition is false';
}
Output:
foo is null
Example Code:
$foo = false;
if($foo === NULL) {
echo 'foo is null';
}
else{
echo 'the condition is false';
}
Output:
the condition is false
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn