How to Check for Numerical Characters in PHP
-
Use
ctype_digit
to Check for Numeric Characters in PHP - Check for Numeric Characters With Regular Expressions in PHP
- Check for Numeric Characters With the Exclusion of the Exponential Sign in PHP
-
Use the
filter_var
Function to Check for Numeric Characters in PHP
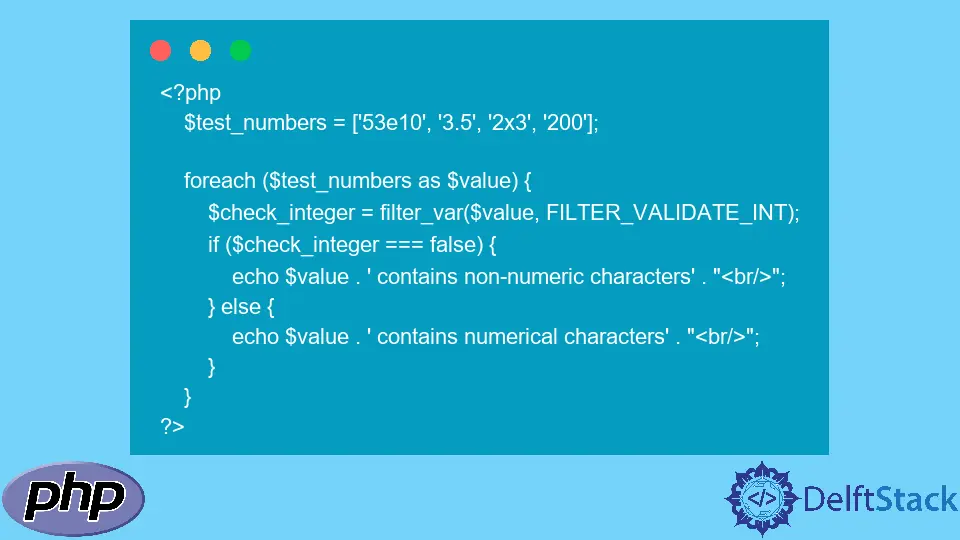
This tutorial will discuss the different methods to check for numeric characters in PHP. We’ll use Regular Expression and built-in functions in PHP.
The functions we’ll use are ctype_digit
, preg_match
, strpos
, is_numeric
, and filter_var
. These methods are useful because they allow you to exclude some non-numeric characters.
Use ctype_digit
to Check for Numeric Characters in PHP
The ctype_digit
function accepts a text string and checks if all the string characters are numerical. The ctype_digit
will not consider the exponential character as a number.
Example:
<?php
$number_with_exponent = '53e10';
if (ctype_digit('$number_with_exponent')) {
echo $number_with_exponent . ' contains numerical characters';
} else {
echo $number_with_exponent . ' contains non-numeric characters';
}
?>
Output:
53e10 contains non-numeric characters
The function will return false
because the string had an exponential character.
Check for Numeric Characters With Regular Expressions in PHP
Regular Expression can check if the characters in a string are all numeric. The first thing you’ll have to do is set up a matching pattern that exclusively matches numerical characters.
In the code block, we’ve set up a Regular Expression
matching pattern with PHP preg_match
function, and then we pass the string to check as the second argument to the preg_match
function.
Example:
<?php
$number_with_exponent = '53e10';
if (preg_match("/^\-?[0-9]*\.?[0-9]+\z/", $number_with_exponent)) {
echo $number_with_exponent . ' contains numerical characters';
} else {
echo $number_with_exponent . ' contains non-numeric characters';
}
?>
Output:
53e10 contains non-numeric characters
Check for Numeric Characters With the Exclusion of the Exponential Sign in PHP
You’ll find this approach useful if you use the if
statement with the is_numeric
function. When using the function is_numeric
on a string containing the exponential sign, the function will return true
because PHP considers the exponential sign a valid numerical character.
Example:
<?php
$number_with_exponent = '53e10';
$check_string_position = strpos($number_with_exponent, 'e');
$check_is_numeric = is_numeric($number_with_exponent);
if ($check_string_position === false && $check_is_numeric) {
echo $number_with_exponent . ' contains numerical characters';
} else {
echo $number_with_exponent . ' contains non-numeric characters';
}
?>
Output:
53e10 contains non-numeric characters
Use the filter_var
Function to Check for Numeric Characters in PHP
The PHP filter_var
function will filter a variable based on a filter. You supply this filter as the second argument to the filter_var
function.
To check for numeric characters, you’ll use a filter called FILTER_VALIDATE_INT
.
Example:
<?php
$test_numbers = ['53e10', '3.5', '2x3', '200'];
foreach ($test_numbers as $value) {
$check_integer = filter_var($value, FILTER_VALIDATE_INT);
if ($check_integer === false) {
echo $value . ' contains non-numeric characters' . "<br/>";
} else {
echo $value . ' contains numerical characters' . "<br/>";
}
}
?>
Output:
53e10 contains non-numeric characters <br />
3.5 contains non-numeric characters <br />
2x3 contains non-numeric characters <br />
200 contains numerical characters <br />
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn