在 PHP 中检查数字字符
Habdul Hazeez
2023年1月30日
-
使用
ctype_digit
检查 PHP 中的数字字符 - 在 PHP 中使用正则表达式检查数字字符
- 在 PHP 中检查排除指数符号的数字字符
-
使用
filter_var
函数检查 PHP 中的数字字符
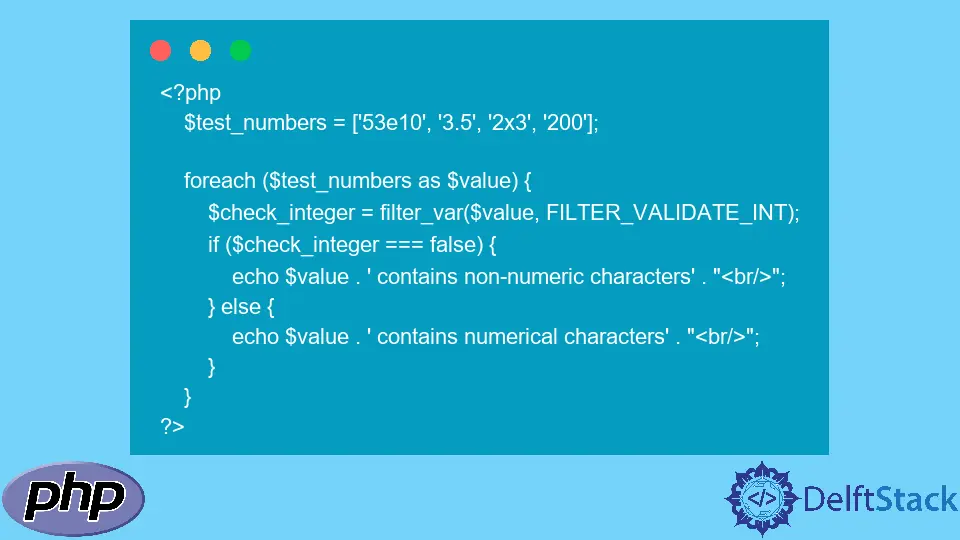
本教程将讨论在 PHP 中检查数字字符的不同方法。我们将在 PHP 中使用正则表达式和内置函数。
我们将使用的函数是 ctype_digit
、preg_match
、strpos
、is_numeric
和 filter_var
。这些方法很有用,因为它们允许你排除一些非数字字符。
使用 ctype_digit
检查 PHP 中的数字字符
ctype_digit
函数接受文本字符串并检查所有字符串字符是否都是数字。ctype_digit
不会将指数字符视为数字。
例子:
<?php
$number_with_exponent = '53e10';
if (ctype_digit('$number_with_exponent')) {
echo $number_with_exponent . ' contains numerical characters';
} else {
echo $number_with_exponent . ' contains non-numeric characters';
}
?>
输出:
53e10 contains non-numeric characters
该函数将返回 false
,因为字符串具有指数字符。
在 PHP 中使用正则表达式检查数字字符
正则表达式可以检查字符串中的字符是否都是数字。你必须做的第一件事是设置一个专门匹配数字字符的匹配模式。
在代码块中,我们使用 PHP preg_match
函数设置了正则表达式匹配模式,然后我们将要检查的字符串作为第二个参数传递给 preg_match
函数。
例子:
<?php
$number_with_exponent = '53e10';
if (preg_match("/^\-?[0-9]*\.?[0-9]+\z/", $number_with_exponent)) {
echo $number_with_exponent . ' contains numerical characters';
} else {
echo $number_with_exponent . ' contains non-numeric characters';
}
?>
输出:
53e10 contains non-numeric characters
在 PHP 中检查排除指数符号的数字字符
如果你将 if
语句与 is_numeric
函数一起使用,你会发现这种方法很有用。在包含指数符号的字符串上使用函数 is_numeric
时,该函数将返回 true
,因为 PHP 认为指数符号是有效的数字字符。
例子:
<?php
$number_with_exponent = '53e10';
$check_string_position = strpos($number_with_exponent, 'e');
$check_is_numeric = is_numeric($number_with_exponent);
if ($check_string_position === false && $check_is_numeric) {
echo $number_with_exponent . ' contains numerical characters';
} else {
echo $number_with_exponent . ' contains non-numeric characters';
}
?>
输出:
53e10 contains non-numeric characters
使用 filter_var
函数检查 PHP 中的数字字符
PHP filter_var
函数将根据过滤器过滤变量。你将此过滤器作为第二个参数提供给 filter_var
函数。
要检查数字字符,你将使用名为 FILTER_VALIDATE_INT
的过滤器。
例子:
<?php
$test_numbers = ['53e10', '3.5', '2x3', '200'];
foreach ($test_numbers as $value) {
$check_integer = filter_var($value, FILTER_VALIDATE_INT);
if ($check_integer === false) {
echo $value . ' contains non-numeric characters' . "<br/>";
} else {
echo $value . ' contains numerical characters' . "<br/>";
}
}
?>
输出:
53e10 contains non-numeric characters <br />
3.5 contains non-numeric characters <br />
2x3 contains non-numeric characters <br />
200 contains numerical characters <br />
作者: Habdul Hazeez
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn