How to Generate Random String in PHP
- Generate Random String in PHP
-
Generate Random String With the
uniqid()
Function -
Generate Random String With the
str_shuffle($string)
Function in PHP - Generate Random Hexadecimal Strings in PHP
- Generate Cryptographically Secure Random Strings in PHP
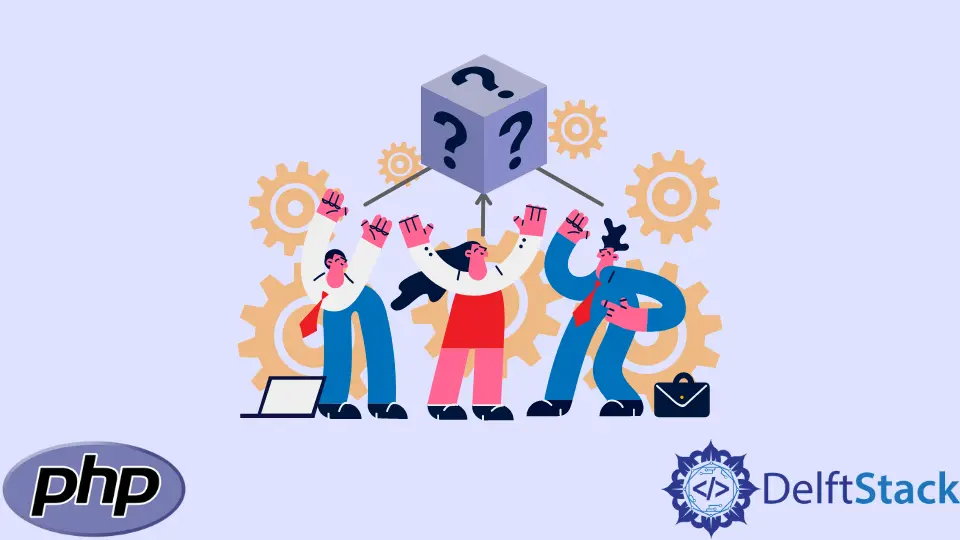
In this tutorial article, we will discuss how to generate the random string in PHP.
Generate Random String in PHP
we are going to define the random string generator function in PHP:
<?php
function random_str_generator ($len_of_gen_str){
$chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
$var_size = strlen($chars);
echo "Random string =";
for( $x = 0; $x < $len_of_gen_str; $x++ ) {
$random_str= $chars[ rand( 0, $var_size - 1 ) ];
echo $random_str;
}
echo "\n";
}
random_str_generator (8)
?>
Output:
Random string =ExmIixDb
It is unnecessary to take only alphabets in the declared variable; you can also add numerics, symbols, e.t.c., anything you want in your randomly generating string.
In the fourth line, we take the declared variable’s length using the strlen()
function. It helps rand( 0, $var_size - 1 )
to generate index from the specific given range and then add that value to newly created variable and the loop helps the rand( 0, $var_size - 1 )
to generate specific length of string.
Generate Random String With the uniqid()
Function
This is another way for the generation of random string by using the built-in function uniqid()
. It generates the 13 characters’ long unique identifier, which is based on the current timestamp. Here is the solution:
<?php
$Random_str = uniqid();
echo "Random String:", $Random_str, "\n";
?>
Output:
Random String:606208975a59f
The uniqid()
function also accepts parameters that may increase the uniqueness of string or identifier. It gives you more described value. Here are some of its solutions:
<?php
echo uniqid('user_');
?>
Output:
user_60620a2b23235
Generate Random String With the str_shuffle($string)
Function in PHP
str_shuffle($string)
is the built-in function, which works as a random string generator function like we have seen above that we use a loop and the rand()
built-in function to generate a random string, but it is also different. We use only the built-in function for generating a unique string which may help us to form a unique password of your demanding length by specifying the range to (str_shuffle($string),0,x(any number))
.
<?php
$x = 0;
$y = 10;
$Strings = '0123456789abcdefghijklmnopqrstuvwxyz';
echo "Gen_rand_str: ",substr(str_shuffle($Strings), $x, $y), "\n";
$a = 0;
$b = 20;
$Strings='0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
echo "Gen_My_Pass: ",'hello.'.substr(str_shuffle($Strings), $a, $b).'.World',"\n";
?>
Output:
Gen_rand_str: b38aenwpkh
Gen_My_Pass: hello.EUMm4TB26CltW8qYnApF.World
It may also help us to generate URLs, random file names e.t.c. It is more secure than the above generator and complex in predicting.
This method is more precise but has some issues like it cannot give the same characters in your random string twice and the random output is long as you gives the input string.
Generate Random Hexadecimal Strings in PHP
md5($string, $raw_output)
and sha1($string,$raw_output)
functions help us to generate random hexadecimal string. In these functions, we use time()
as the input for achieving output, and if we want a 32-character hexadecimal string, then we use md5()
, and for 40-character, we use sha1()
. Finally, we can also extract a random string of our specified length by the substr()
function.
<?php
echo "Out1: ",substr(md5(time()), 0, 16),"\n";
echo "Out2: ",substr(sha1(time()), 0, 16),"\n";
echo "Out3: ",md5(time()),"\n";
echo "Out4: ",sha1(time()),"\n";
?>
Output:
Out1: fcc78a076a5b743d
Out2: ae03e817c0dc6048
Out3: fcc78a076a5b743d8916038b48a6b0ee
Out4: ae03e817c0dc6048c19b708ce411a058294425b1
Generate Cryptographically Secure Random Strings in PHP
This generator is the most important and helpful feature of PHP. All generators which we will discuss above are not cryptographically secure. Cryptographically secure means it can also generate a random string, but it is hard for a third party to generate the same random number. That’s why we can say it cryptographically secure.
random_bytes($length)
is the function that can generate a cryptographically secure random string. The user specifies the length parameter. See the example below.
<?php
echo "Output-1: ",bin2hex(random_bytes(10)),"\n";
echo "Output-2: ",bin2hex(random_bytes(20)),"\n";
echo "Output-3: ",bin2hex(random_bytes(24)),"\n";
?>
Output:
Output-1: 911fc716798ce464c7c3
Output-2: 182fedf65a90b66139d80dbfc0f82912b73f37cd
Output-3: c3408d3a0dea41251dce940fe550452ca180cf5effaad235
As you have seen in the above code, we have used the bin2hex()
function. When random_bytes($length)
gives a specific output, then the bin2hex()
converts it into hexadecimal values. Which may double the value of the function random_bytes($length).
The openssl_random_pseudo_bytes($length, &$crypto_strong)
function is also used for generating randomly cryptographically string. The crypto_strong
parameter is used for analyzing that †he string is generated from a cryptographically secure algorithm or not.
If we want a cryptographically secure random string that utilizes all strings from a to z, we use the below codes.
<?php
function secure_random_string($length) {
$rand_string = '';
for($i = 0; $i < $length; $i++) {
$number = random_int(0, 36);
$character = base_convert($number, 10, 36);
$rand_string .= $character;
}
return $rand_string;
}
echo "Sec_Out_1: ",secure_random_string(10),"\n";
echo "Sec_Out_2: ",secure_random_string(10),"\n";
echo "Sec_Out_3: ",secure_random_string(10),"\n";
?>
Output:
Sec_Out_1: znxtaqynoi
Sec_Out_2: 10k10zkddntm
Sec_Out_3: ook8gfck6u
We specify the length of the random string in the secure_random_string()
function. In this function, we use a loop in which random_int()
generates random integers from the given specific range. After it, we convert the integer from base 10 to 36 with the base_convert()
function. Eventually, our resulting character is from any digit from 0-9 and any character from a-z. It is one more way to generate a randomly cryptographical string.