How to Use Echo Shorthand in PHP
-
Introduction to the
echo
Statement in PHP -
Replace the
echo
Statement With the<?= ?>
Shorthand Tag in PHP - Best Practices
- Performance Considerations
- Conclusion
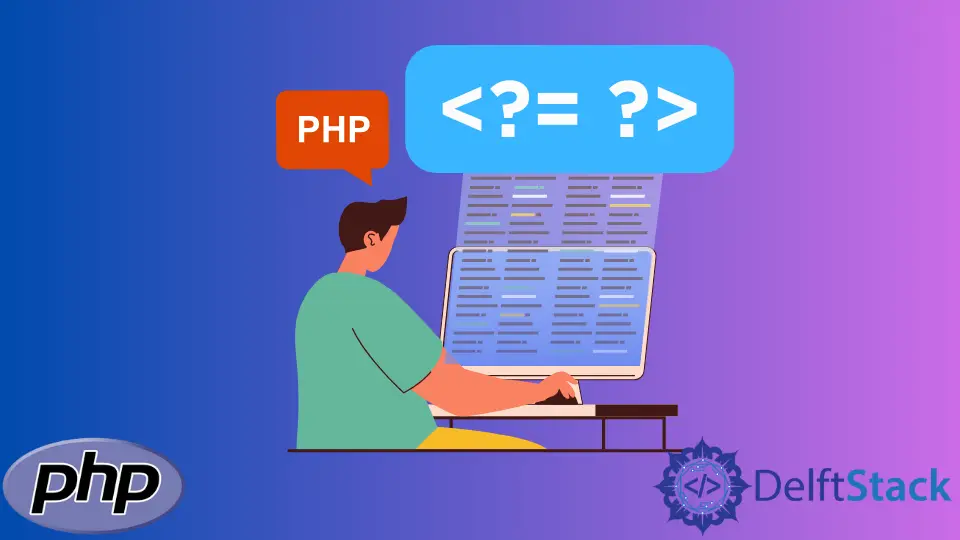
In PHP, the echo
statement is a fundamental tool for outputting content to the browser. It’s a versatile function that allows developers to display text, variables, and even HTML elements.
However, there’s a more concise and convenient way to achieve the same result: using the <?= ?>
shorthand tag.
In this article, we will explore the use of <?= ?>
shorthand tags as a substitute for the traditional echo
statement in PHP.
Introduction to the echo
Statement in PHP
The echo
statement is a language construct in PHP used to output text or HTML content to the browser. It’s incredibly versatile and is essential for generating dynamic web pages, displaying user inputs, and interacting with databases.
The print
statement is also widely used to display the output. The echo
statement can also be written with parenthesis as echo()
.
It can output multiple expressions, unlike print
, which can only output a single expression. It does not have any written value, and it is faster than the print
statement.
The echo
statement lies at the heart of dynamic web development. It allows PHP scripts to generate content on the server and send it to the client’s browser. This enables the creation of interactive and data-driven web applications.
Simple Output
echo "Hello, World!";
The above code will display the "Hello, World!"
text in the browser. The echo
statement is followed by the content we want to display, enclosed in quotes.
Escaping Characters
echo "She said, \"Hello!\"";
To include quotes within the echoed content, we use the backslash \
to escape them. This ensures they are treated as regular characters and not as delimiters.
Displaying Scalars
$name = "John Doe";
echo "Hello, $name!";
The echo
statement can also display the value of a variable. In this example, the value of the variable $name
will be inserted into the string.
Rendering Arrays and Objects
$colors = ['red', 'green', 'blue'];
echo "Available Colors: " . implode(", ", $colors);
Arrays and objects can be displayed using echo
, but they need to be converted to strings first. Here, implode
is used to concatenate array elements into a single string.
Concatenation vs. Comma Separation
$firstName = "John";
$lastName = "Doe";
echo $firstName . " " . $lastName;
Both concatenation (using .
) and comma separation can be used to output multiple values. However, concatenation offers more control over formatting and is often preferred.
In the example below, we have shown different ways to use the echo
statement in PHP.
$num = 2;
$num1 = 3;
$place = "tree";
echo "Hello there!<br>";
echo $num + $num1."<br>";
echo "There are ".$num. " birds in the ".$place."!<br>";
echo "It ", "is ", "raining ", "outside. ";
First, we display a simple text. We can also display the arithmetic operations with the echo
statement.
We have used the HTML <br>
tags along with the echo
statement. We have concatenated numbers along with the text while using the echo
statement.
We have also found out how we can write multiple expressions in the echo
statement.
Output:
Hello there!
5
There are 2 birds in the tree!
It is raining outside.
Replace the echo
Statement With the <?= ?>
Shorthand Tag in PHP
While working in PHP, we may have to use HTML tags most often. We might want to use the PHP inside of HTML sometimes.
In that case, we have to write the PHP opening tag <?php
and the closing tag ?>
every time we want to write PHP.
Suppose we have to display something; we have to use the echo
statement inside the PHP tags. It might become tedious to follow this approach every time.
In PHP, <?= ?>
shorthand tags are a concise alternative to the traditional echo
statement. They are used to output content directly to the browser, making PHP code more readable and compact.
So, instead of writing the echo
statement inside the PHP tags, we can use the <?= ?>
shorthand tag of the echo
tag. It is much simpler and shorter than writing the echo
statement inside the PHP tags.
Replacing echo
With <?= ?>
Here’s a simple example of using <?= ?>
shorthand tags to display text:
<?= "Hello, World!"; ?>
In this code snippet, "Hello, World!"
will be directly displayed in the browser without the need for an explicit echo
statement.
Displaying Variables With Shorthand Tags
You can use shorthand tags to display the values of variables:
<?php $name = "John"; ?>
<?= "Hello, $name!"; ?>
The value of the $name
variable is embedded directly into the string, making it concise and readable.
String Concatenation
String concatenation is also possible with shorthand tags:
<?php
$firstName = "John";
$lastName = "Doe";
?>
<?= $firstName . " " . $lastName; ?>
This code snippet concatenates the values of $firstName
and $lastName
and displays the full name.
Embedding HTML and Variables
Shorthand tags can be used to mix HTML and PHP seamlessly:
<?= "<h1>Welcome, $name!</h1>"; ?>
In this example, the variable $name
is embedded in an HTML <h1>
tag.
Mixing PHP and HTML
Shorthand tags allow for easy mixing of PHP and HTML within the same code block:
<?= "Today is " . date('Y-m-d'); ?>
This code displays the current date within the string, demonstrating the convenience of shorthand tags for integrating PHP and HTML.
Conditional Output
Shorthand tags can handle conditional statements:
<?= ($loggedIn) ? "Welcome back!" : "Please log in"; ?>
This code will display a different message based on the value of the $loggedIn
variable.
Using Functions
Functions and expressions can be used within shorthand tags as well:
<?= strtoupper("This text is in uppercase."); ?>
The strtoupper
function is used to convert the string to uppercase, which is then displayed.
Best Practices
Code Readability
While shorthand tags are convenient, it’s important to maintain code readability. Avoid overusing them, especially for complex logic or large blocks of HTML.
Consistency
Choose a consistent approach throughout your codebase. If you decide to use shorthand tags, stick to that convention.
Error Handling
Be mindful of error handling; if shorthand tags are disabled in the PHP configuration, your code will break. It’s essential to have fallback mechanisms or provide clear instructions for enabling shorthand tags.
Performance Considerations
Shorthand Tags vs. echo
In terms of performance, there is no significant difference between shorthand tags and echo
. The choice between them should be based on code style and readability.
Benchmarking
To compare the performance of both approaches, consider running benchmarks on your specific application. The impact on performance is usually negligible and should not be the sole factor in your decision.
Conclusion
In conclusion, mastering the echo
statement in PHP is essential for dynamic web development. It allows for the seamless generation of content and interaction with users.
However, the introduction of <?= ?>
shorthand tags offers a more concise and readable alternative. These tags prove invaluable for embedding PHP within HTML and simplifying variable output.
While using shorthand tags, it’s crucial to maintain code readability and consistency. Avoid overuse, especially for complex logic or extensive HTML blocks.
Additionally, consider error handling, as disabling shorthand tags in the PHP configuration may affect your code’s functionality. In terms of performance, both shorthand tags and echo
offer similar results, so the choice should be based on code style and readability preferences.
Ultimately, incorporating shorthand tags into your PHP code can enhance its maintainability and make it more concise. Experiment with them to find the balance that best suits your projects.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn