How to Add Line Break in PHP
-
Use the
nl2br()
Function and the Newline\n
Escape Sequence to Add the Line Break Withinecho
in PHP - Use the File Handling Method and Its Functions to Add the Line Break in PHP
-
Use the
<br>
Tag to Add a Line Break Within theecho
in PHP
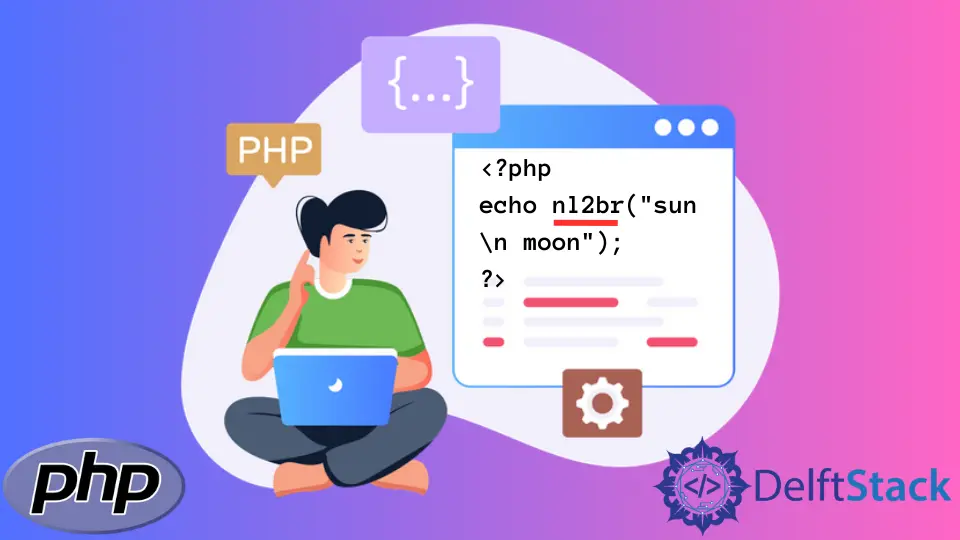
We will introduce a method to add line break within the echo
statement using the nl2br()
function and the \n
escape sequence. The nl2br()
function returns the string with the <br/>
or the <br>
tags of HTML. The \n
escape sequence denotes the new line.
We will introduce another method to add line breaks using the file handling functions in PHP. We use the functions like fopen()
, fwrite()
and file close()
to achieve the goal. We also make use of the \n
escape sequence in this method.
We will demonstrate a straightforward method to add line breaks within the echo
statement using the <br>
tag in PHP. We use the dot operator to use the <br>
tag between the strings to apply the line breaks.
Use the nl2br()
Function and the Newline \n
Escape Sequence to Add the Line Break Within echo
in PHP
We use the nl2br()
function and the newline \n
escape sequence to insert the line break within the echo
statement in PHP. The nl2br()
function inserts the HTML line breaks before the new line, and the \n
escape sequence denotes the new line. The function returns the string with the <br>
or <br/>
HTML tags. For example, use the nl2br()
function in the echo
statement. Write a string sun
inside the nl2br()
function and use the \n
escape sequence. Write another string, moon
, right after the escape sequence.
Do not get confused with /n
as \n
. Firstly we write the backward slash and then n
. Make the use of double quotes for the parameters inside the nl2br()
function. The use of single quotes results in the printing of the exact parameter written in the function. Visit the PHP manual to learn about the nl2br()
function.
Example Code:
# php 7.*
<?php
echo nl2br("sun \n moon");
?>
Output:
sun
moon
Use the File Handling Method and Its Functions to Add the Line Break in PHP
We can use the file handling functions like fopen()
, fwrite()
, and file close()
to create a file and write some text in it. We use the \n
escape sequence as in the first method. As a result, the text written after the \n
will appear in the next line in the file. We use the fopen()
function to create a new text file in write mode. We use the fwrite()
function to write the particular text to the created file. The flclose()
function closes the file stream.
For example, create a variable $file
and assign the fopen()
function to it. Inside the function, write the first parameter as test.txt
, which is the file to be created, and write w+
as the second parameter to denote the write mode. Write a string as Sun \n Moon
in a variable $text
. Use fwrite()
function and pass the $file
and $text
variables as the parameter. Close the file stream using the fclose()
function with the $file
as the parameter.
The example below creates a text file test.txt
in the root directory when the script runs in the server. Then, it inserts the texts into the file. We can view the output by opening the text file. Have a look at the PHP manual to learn about the file operations.
Code Example:
#php 7.x
<?php
$file = fopen("test.txt", "w+");
$text = "Sun \n Moon";
fwrite($file, $text);
fclose($file);
?>
Output:
Sun
Moon
Use the <br>
Tag to Add a Line Break Within the echo
in PHP
We can use the <br>
HTML tags to insert the line break in PHP. This tag is used in HTML to insert the line breaks. We can use the .
dot operator before and after the <br>
tag to specify the string’s line break. The <br>
tag should be enclosed with double quotes. For example, write a string Welcome to Delft
inside double-quotes. Use a dot operator after it and write the <br>
tag enclosed with double quotes. Again use the dot operator and write another string, "Have a great time here"
enclosed with double-quotes. Use an echo
statement to print it.
Example Code:
#php 7.x
<?php
echo "Welcome to Delft" ."<br>". "Have a great time here"
?>
Output:
Welcome to Delft
Have a great time here
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn