cURL GET Request Using PHP
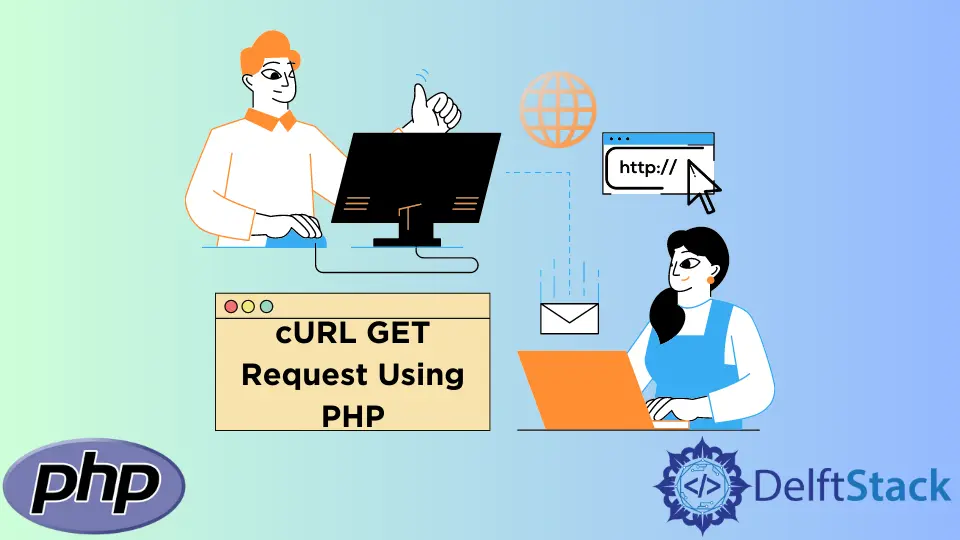
Over the web, and primarily the HTTPS, we use GET
requests to obtain specific resource representation. To obtain data, GET
methods are employed.
Especially in API and web scraping scenarios, developers use language-specific methods. We use cURL
functions to handle GET
requests in PHP.
The cURL
library handles operations from initialization, connection setup and transfers to resource return. To achieve all these operations, built-in functions are available such as curl_close()
, curl_init()
to curl_exec()
.
This tutorial discusses the different use cases for cURL
GET
requests and the corresponding functions that make it happen.
Use curl_init()
and curl_setopt()
to Get Request in PHP
The typical format to get a request from another server or user involves using the following basic functions.
curl_init(); // initializes a cURL session
curl_setopt(); // changes the cURL session behavior with options
curl_exec(); // executes the started cURL session
curl_close(); // closes the cURL session and deletes the variable made by curl_init();
You will see all four functions across this article and most of the GET
request code you will write using the cURL
library.
Now, for some example usage, we will assign the variable $url
with the URL of the site that we want to get a request from and initiate a cURL
session using the curl_init()
functions. Afterward, we specify the option for the cURL
transfer carried out on the specified URL.
The first curl_setopt()
function statement holds the “URL to fetch” option with the $url
variable assigned value. The second curl_setopt()
function statement holds the return the transfer as a string
option, holding a Boolean value.
<?php
$url = "https://reqbin.com/echo";
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//for debug only!
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
$resp = curl_exec($curl);
curl_close($curl);
var_dump($resp);
?>
The output of the above PHP code is below.
Use cURL
With APIs (JSON) in PHP
Typically, when interacting with APIs, the data response would often be JSON and using cURL
functions, we can properly process these data objects in attribute-value pairs and arrays format using curl_getinfo()
and json_encode()
functions, among others.
Here, we will interact with a demo User
API, obtain JSON data using the cURL
library, and encode the JSON file using the appropriate function.
-
Initialize the URL variable and
cURL
session.$url = 'https://jsonplaceholder.typicode.com/users'; // Sample example to get data. $resource = curl_init($url);
-
Setup the
cURL
options and info variables.curl_setopt($resource, CURLOPT_RETURNTRANSFER, true); $result = curl_exec($resource); $info = curl_getinfo($resource); $code = curl_getinfo($resource, CURLINFO_HTTP_CODE);
-
Debug to check for response status code (optional step), but you should see
200
.echo $result.'<br>'; echo "<pre>"; print_r($info); echo "</pre>"; // Get response status code echo "<pre>"; print_r($code); echo "</pre>";
-
Close the
cURL
session to better manage resources.curl_close($resource);
-
Create the array template to store the data obtained from the API via JSON.
// set_opt_array $user = [ 'name' => 'John Doe', 'username' => 'john', 'email' => 'john@example.com' ];
-
Use the
curl_init()
andcurl_setopt_array()
to process the JSON data parsed from the URL using all the important options.$resource = curl_init(); curl_setopt_array($resource, [ CURLOPT_URL => $url, CURLOPT_RETURNTRANSFER => true, CURLOPT_POST => true, CURLOPT_HTTPHEADER => ['content-type: application/json'], CURLOPT_POSTFIELDS => json_encode($user), ]);
-
Use the
curl_exec()
andcurl_close()
function to execute the initializedcURL
session and close thecURL
session and frees all resources, respectively. However, in PHP 8.0.0 and above, thecurl_close()
function has no effect.$result = curl_exec($resource); // creates and returns result curl_close($resource); echo $result;
The output of the code will result in the below PHP multi-dimensional array.
[
{
"id": 1,
"name": "Leanne Graham",
"username": "Bret",
"email": "Sincere@april.biz",
"address": {
"street": "Kulas Light",
"suite": "Apt. 556",
"city": "Gwenborough",
"zipcode": "92998-3874",
"geo": {
"lat": "-37.3159",
"lng": "81.1496"
}
},
"phone": "1-770-736-8031 x56442",
"website": "hildegard.org",
"company": {
"name": "Romaguera-Crona",
"catchPhrase": "Multi-layered client-server neural-net",
"bs": "harness real-time e-markets"
}
},
{
"id": 2,
"name": "Ervin Howell",
"username": "Antonette",
"email": "Shanna@melissa.tv",
"address": {
"street": "Victor Plains",
"suite": "Suite 879",
"city": "Wisokyburgh",
"zipcode": "90566-7771",
"geo": {
"lat": "-43.9509",
"lng": "-34.4618"
}
},
"phone": "010-692-6593 x09125",
"website": "anastasia.net",
"company": {
"name": "Deckow-Crist",
"catchPhrase": "Proactive didactic contingency",
"bs": "synergize scalable supply-chains"
}
},
{
"id": 3,
"name": "Clementine Bauch",
"username": "Samantha",
"email": "Nathan@yesenia.net",
"address": {
"street": "Douglas Extension",
"suite": "Suite 847",
"city": "McKenziehaven",
"zipcode": "59590-4157",
"geo": {
"lat": "-68.6102",
"lng": "-47.0653"
}
},
"phone": "1-463-123-4447",
"website": "ramiro.info",
"company": {
"name": "Romaguera-Jacobson",
"catchPhrase": "Face to face bifurcated interface",
"bs": "e-enable strategic applications"
}
},
....
]
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn