使用 PHP 的 cURL GET 請求
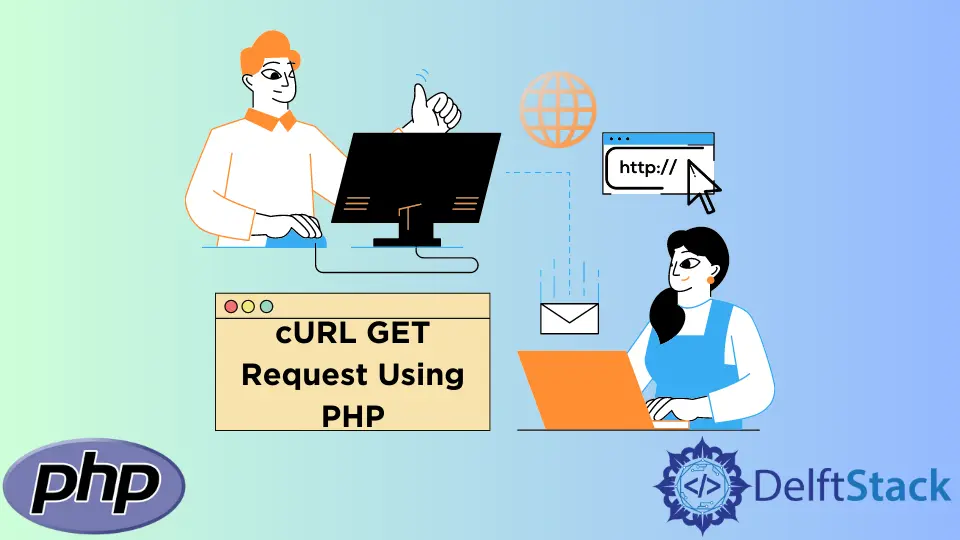
在網路上,主要是 HTTPS,我們使用 GET
請求來獲取特定的資源表示。為了獲取資料,使用了 GET
方法。
特別是在 API 和 Web 抓取場景中,開發人員使用特定於語言的方法。我們使用 cURL
函式來處理 PHP 中的 GET
請求。
cURL
庫處理從初始化、連線設定和傳輸到資源返回的操作。為了實現所有這些操作,可以使用內建函式,例如 curl_close()
、curl_init()
到 curl_exec()
。
本教程討論了 cURL
GET
請求的不同用例以及實現它的相應函式。
使用 curl_init()
和 curl_setopt()
在 PHP 中獲取請求
從另一個伺服器或使用者獲取請求的典型格式涉及使用以下基本功能。
curl_init(); // initializes a cURL session
curl_setopt(); // changes the cURL session behavior with options
curl_exec(); // executes the started cURL session
curl_close(); // closes the cURL session and deletes the variable made by curl_init();
你將看到本文中的所有四個函式以及你將使用 cURL
庫編寫的大部分 GET
請求程式碼。
現在,對於一些示例用法,我們將為變數 $url
分配我們要從中獲取請求的站點的 URL,並使用 curl_init()
函式啟動 cURL
會話。之後,我們指定在指定 URL 上執行 cURL
傳輸的選項。
第一個 curl_setopt()
函式語句包含帶有 $url
變數賦值的 URL to fetch
選項。第二個 curl_setopt()
函式語句包含以字串形式返回傳輸
選項,包含一個布林值。
<?php
$url = "https://reqbin.com/echo";
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//for debug only!
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
$resp = curl_exec($curl);
curl_close($curl);
var_dump($resp);
?>
上述 PHP 程式碼的輸出如下。
在 PHP 中將 cURL
與 API (JSON) 結合使用
通常,當與 API 互動時,資料響應通常是 JSON 並使用 cURL
函式,我們可以使用 curl_getinfo()
和 json_encode()
函式以屬性值對和陣列格式正確處理這些資料物件,其中。
在這裡,我們將與演示 User
API 進行互動,使用 cURL
庫獲取 JSON 資料,並使用適當的函式對 JSON 檔案進行編碼。
-
初始化 URL 變數和
cURL
會話。$url = 'https://jsonplaceholder.typicode.com/users'; // Sample example to get data. $resource = curl_init($url);
-
設定
cURL
選項和資訊變數。curl_setopt($resource, CURLOPT_RETURNTRANSFER, true); $result = curl_exec($resource); $info = curl_getinfo($resource); $code = curl_getinfo($resource, CURLINFO_HTTP_CODE);
-
除錯以檢查響應狀態程式碼(可選步驟),但你應該看到
200
。echo $result.'<br>'; echo "<pre>"; print_r($info); echo "</pre>"; // Get response status code echo "<pre>"; print_r($code); echo "</pre>";
-
關閉
cURL
會話以更好地管理資源。curl_close($resource);
-
建立陣列模板來儲存通過 JSON 從 API 獲取的資料。
// set_opt_array $user = [ 'name' => 'John Doe', 'username' => 'john', 'email' => 'john@example.com' ];
-
使用
curl_init()
和curl_setopt_array()
使用所有重要選項處理從 URL 解析的 JSON 資料。$resource = curl_init(); curl_setopt_array($resource, [ CURLOPT_URL => $url, CURLOPT_RETURNTRANSFER => true, CURLOPT_POST => true, CURLOPT_HTTPHEADER => ['content-type: application/json'], CURLOPT_POSTFIELDS => json_encode($user), ]);
-
分別使用
curl_exec()
和curl_close()
函式執行初始化的cURL
會話和關閉cURL
會話並釋放所有資源。但是,在 PHP 8.0.0 及更高版本中,curl_close()
函式無效。$result = curl_exec($resource); // creates and returns result curl_close($resource); echo $result;
程式碼的輸出將產生下面的 PHP 多維陣列。
[
{
"id": 1,
"name": "Leanne Graham",
"username": "Bret",
"email": "Sincere@april.biz",
"address": {
"street": "Kulas Light",
"suite": "Apt. 556",
"city": "Gwenborough",
"zipcode": "92998-3874",
"geo": {
"lat": "-37.3159",
"lng": "81.1496"
}
},
"phone": "1-770-736-8031 x56442",
"website": "hildegard.org",
"company": {
"name": "Romaguera-Crona",
"catchPhrase": "Multi-layered client-server neural-net",
"bs": "harness real-time e-markets"
}
},
{
"id": 2,
"name": "Ervin Howell",
"username": "Antonette",
"email": "Shanna@melissa.tv",
"address": {
"street": "Victor Plains",
"suite": "Suite 879",
"city": "Wisokyburgh",
"zipcode": "90566-7771",
"geo": {
"lat": "-43.9509",
"lng": "-34.4618"
}
},
"phone": "010-692-6593 x09125",
"website": "anastasia.net",
"company": {
"name": "Deckow-Crist",
"catchPhrase": "Proactive didactic contingency",
"bs": "synergize scalable supply-chains"
}
},
{
"id": 3,
"name": "Clementine Bauch",
"username": "Samantha",
"email": "Nathan@yesenia.net",
"address": {
"street": "Douglas Extension",
"suite": "Suite 847",
"city": "McKenziehaven",
"zipcode": "59590-4157",
"geo": {
"lat": "-68.6102",
"lng": "-47.0653"
}
},
"phone": "1-463-123-4447",
"website": "ramiro.info",
"company": {
"name": "Romaguera-Jacobson",
"catchPhrase": "Face to face bifurcated interface",
"bs": "e-enable strategic applications"
}
},
....
]
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn