How to Use CSS Style in PHP
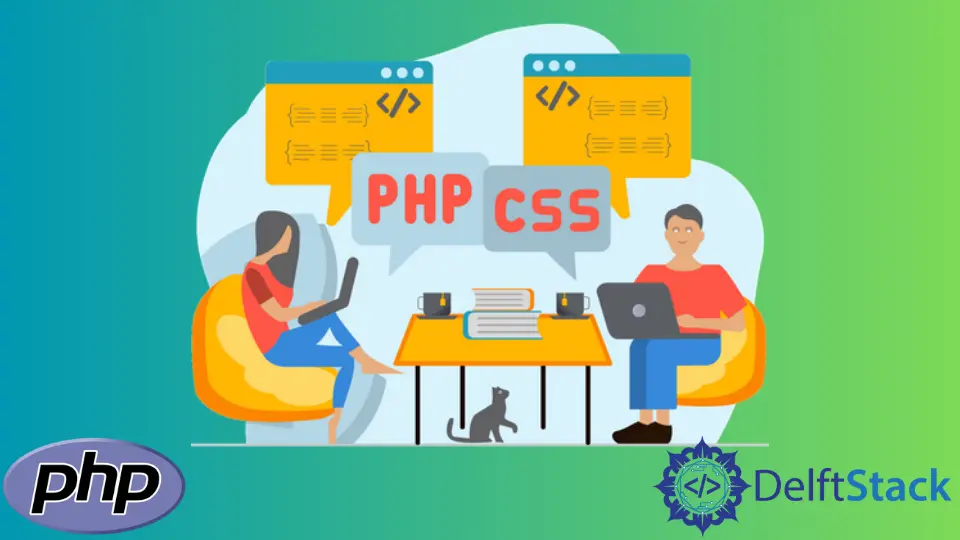
This article will teach you three methods that’ll help you use CSS styles in PHP.
The first method is via a PHP-only file, and the second is to embed PHP in an HTML+CSS file. Then the third method will use inline CSS in PHP echo
statements.
Use CSS in a PHP-Only File
A standard HTML file can embed CSS styles in the <head>
element or link to an external CSS file. By default, this CSS file will have the css
extension, but it can also have the php
extension.
This means you can write CSS code, save it as a PHP file and link it to your HTML. In this PHP file, you can do more than what you’ll do in a CSS file; you can write PHP code.
First, you can define a PHP code block with CSS property and values stored as variables. Then outside the PHP block, you can write normal CSS that’ll use the variables as values of CSS properties.
We’ve done that in the following; save it as styles.php
.
<?php
// The "header" is the most important part of
// this code. Without it, it will not work.
header("Content-type: text/css");
$font_family = 'Trebuchet MS, Verdana, Helvetica';
$font_size = '1.2em';
$background_color = '#000000';
$font_color = '#ffffff';
// Close the PHP code block.
?>
body {
background-color: <?php echo $background_color; ?>;
color: <?php echo $font_color; ?>;
font-size: <?php echo $font_size; ?>;
font-family: <?php echo $font_family; ?>;
}
Save the following HTML, and ensure you’ve linked styles.php
in the <link>
tag.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Webpage using CSS styles generated with PHP</title>
<!-- Link styles.php as the CSS file -->
<link rel="stylesheet" type="text/css" href="styles.php">
</head>
<body>
<h1>Hello, We styled this page using CSS in a PHP file!</h1>
<h1>How cool is that?</h1>
</body>
</html>
Output:
Use CSS in a PHP+HTML File
HTML can use CSS via the <style>
tag or the <link>
tag, which can contain PHP in a dedicated PHP block. If the PHP code generates or manipulates HTML code, the linked CSS code can style the HTML.
For example, you can style the table using CSS if PHP pulls database records to make an HTML table. To show how to do this, create a database called my_website
in MySQL.
Next, create a site_users
table in my_website
using the following queries.
CREATE TABLE site_users (
user_id INT NOT NULL AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
PRIMARY KEY (user_id)) ENGINE = InnoDB;
Insert data into the site_users
table.
INSERT INTO site_users (username, email) VALUES ('user_1', 'user_1@gmail.com');
INSERT INTO site_users (username, email) VALUES ('user_2', 'user_2@gmail.com');
INSERT INTO site_users (username, email) VALUES ('user_3', 'user_3@gmail.com');
Now, in the following, we have HTML, PHP, and CSS. The CSS is in the <head>
element; the PHP is in a PHP block within the HTML.
The PHP creates an HTML table using records from the site_users
table. When the PHP generates the table, the CSS will style it.
<!doctype html>
<head>
<meta charset="utf-8">
<title>Style MySQL table with CSS</title>
<style>
/* This CSS will style the table generated by PHP. */
table { border-collapse: collapse; width: 30em; font-size: 1.2em; }
table, th, td { border: 2px solid #1a1a1a; }
td,th { padding: 0.5em; }
/* Create a striped table. */
tr:nth-child(even) { background-color: #f2f2f2; }
/* General body styles. */
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
</style>
</head>
<body>
<main>
<!--
We generated this table using PHP, and the CSS
style in the <style> tag will apply to it.
This is another way to use CSS in PHP.
-->
<?php
// Connect to the database. Change the connection
/// settings based on your environment.
$connect_to_mysql = new mysqli("localhost", "root", "", "my_website");
// Select the data from the site_users table.
$site_users = $connect_to_mysql->query("SELECT * FROM site_users")->fetch_all(MYSQLI_ASSOC);
// Get keys from the first row.
$table_header = array_keys(reset($site_users));
// Print the table.
echo "<table>";
// Print the table headers.
echo "<tr>";
foreach ($table_header as $value) {
echo "<th align='left'>" . $value . "</th>";
}
echo "</tr>";
// Print the table rows
foreach ($site_users as $row) {
echo "<tr>";
foreach ($row as $value) {
if (is_null($value)) {
echo "<td>NULL</td>";
} else {
echo "<td>" . $value . "</td>";
}
}
echo "</tr>";
}
echo "</table>";
?>
</main>
</body>
Output:
Use Inline CSS in PHP echo
Statements
PHP works well with HTML and has the echo
statement that can send HTML with inline CSS to the web browser. This is useful when debugging or sending large chunks of HTML to the web browser.
The following shows you how to use inline CSS with PHP echo
. We define the text and store three colors in the $colors_array
.
Then we use foreach
to loop through the $colors_array
, and we set the array element as the value of the inline CSS. When you run the code, the text appears three times with different colors.
<?php
$sample_text = "We generated this text color using inline CSS in PHP.";
$colors_array = ['red', 'green', 'blue'];
foreach ($colors_array as $value) {
// Use inline CSS with PHP echo.
echo "<p style='color: $value'>" . $sample_text . "</p>";
}
?>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn